Axisymmetric rotor#
This notebook demonstrates the process of running a Workbench service on a local machine to solve both 2D general axisymmetric rotor and 3D rotor models using PyMechanical. It includes steps for uploading project files, executing scripts, downloading results, and displaying output images.
[1]:
import os
import pathlib
[2]:
from ansys.workbench.core import launch_workbench
from ansys.mechanical.core import connect_to_mechanical
Launch the Workbench service on the local machine using specific options. Define the working directory and subdirectories for assets, scripts, and geometry databases (agdb). The launch_workbench
function starts a Workbench session with the specified directory.
[3]:
workdir = pathlib.Path("__file__").parent
[4]:
assets = workdir / "assets"
scripts = workdir / "scripts"
[5]:
wb = launch_workbench(client_workdir=str(workdir.absolute()))
Upload the project files to the server using the upload_file_from_example_repo
method. The files uploaded are axisymmetric_model.agdb
, rotor_3d_model.agdb
.
[6]:
wb.upload_file_from_example_repo("axisymmetric-rotor/agdb/axisymmetric_model.agdb")
wb.upload_file_from_example_repo("axisymmetric-rotor/agdb/rotor_3d_model.agdb")
Uploading axisymmetric_model.agdb: 100%|██████████| 2.52M/2.52M [00:00<00:00, 70.3MB/s]
Uploading rotor_3d_model.agdb: 100%|██████████| 2.52M/2.52M [00:00<00:00, 84.6MB/s]
Execute a Workbench script (project.wbjn
) to define the project and load the geometry. The log file is set to wb_log_file.log
and the name of the system created is stored in sys_name
and printed.
[7]:
export_path = 'wb_log_file.log'
wb.set_log_file(export_path)
sys_name = wb.run_script_file(str((assets / "project.wbjn").absolute()), log_level='info')
print(sys_name)
['SYS', 'SYS 4']
Start a PyMechanical server for the system and create a PyMechanical client session to solve the 2D general axisymmetric rotor model. The project directory is printed to verify the connection.
[8]:
server_port = wb.start_mechanical_server(system_name=sys_name[1])
[9]:
mechanical = connect_to_mechanical(ip='localhost', port=server_port)
[10]:
print(mechanical.project_directory)
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\
Read and execute the script axisymmetric_rotor.py
via the PyMechanical client to mesh and solve the 2D general axisymmetric rotor model. The output of the script is printed.
[11]:
with open(scripts / "axisymmetric_rotor.py") as sf:
mech_script = sf.read()
mech_output = mechanical.run_python_script(mech_script)
print(mech_output)
{"Total Deformation": "0.79262294403210676 [mm]", "Total Deformation 2": "0.93934788182426 [mm]"}
Specify the Mechanical directory for the Modal Campbell Analysis and fetch the working directory path. Download the solver output file (solve.out
) from the server to the client’s current working directory and print its contents.
[12]:
mechanical.run_python_script(f"solve_dir=ExtAPI.DataModel.AnalysisList[2].WorkingDir")
result_solve_dir_server = mechanical.run_python_script(f"solve_dir")
print(f"All solver files are stored on the server at: {result_solve_dir_server}")
All solver files are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-6\MECH\
[13]:
solve_out_path = os.path.join(result_solve_dir_server, "solve.out")
[14]:
def write_file_contents_to_console(path):
"""Write file contents to console."""
with open(path, "rt") as file:
for line in file:
print(line, end="")
[15]:
current_working_directory = os.getcwd()
mechanical.download(solve_out_path, target_dir=current_working_directory)
solve_out_local_path = os.path.join(current_working_directory, "solve.out")
write_file_contents_to_console(solve_out_local_path)
os.remove(solve_out_local_path)
Downloading dns:///127.0.0.1:54649:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-6\MECH\solve.out to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\solve.out: 100%|██████████| 80.0k/80.0k [00:00<?, ?B/s]
Ansys Mechanical Enterprise
*------------------------------------------------------------------*
| |
| W E L C O M E T O T H E A N S Y S (R) P R O G R A M |
| |
*------------------------------------------------------------------*
***************************************************************
* ANSYS MAPDL 2024 R2 LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2024 Ansys, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of Ansys, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the Ansys, Inc. online documentation or the Ansys, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* Ansys, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* Ansys, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the Ansys, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the Ansys, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
2024 R2
Point Releases and Patches installed:
Ansys Service Pack 2024 R2.01
Ansys Service Pack 2024 R2.02
Ansys Service Pack 2024 R2.03
Ansys, Inc. License Manager 2024 R2
Ansys, Inc. License Manager 2024 R2.01
Ansys, Inc. License Manager 2024 R2.02
Ansys, Inc. License Manager 2024 R2.03
Discovery 2024 R2
Discovery 2024 R2.01
Discovery 2024 R2.02
Discovery 2024 R2.03
Core WB Files 2024 R2
Core WB Files 2024 R2.01
Core WB Files 2024 R2.02
Core WB Files 2024 R2.03
SpaceClaim 2024 R2
SpaceClaim 2024 R2.01
SpaceClaim 2024 R2.02
SpaceClaim 2024 R2.03
Icepak (includes CFD-Post) 2024 R2
Icepak (includes CFD-Post) 2024 R2.01
Icepak (includes CFD-Post) 2024 R2.02
Icepak (includes CFD-Post) 2024 R2.03
CFD-Post only 2024 R2
CFD-Post only 2024 R2.01
CFD-Post only 2024 R2.02
CFD-Post only 2024 R2.03
CFX (includes CFD-Post) 2024 R2
CFX (includes CFD-Post) 2024 R2.01
CFX (includes CFD-Post) 2024 R2.02
CFX (includes CFD-Post) 2024 R2.03
Chemkin 2024 R2
Chemkin 2024 R2.01
Chemkin 2024 R2.02
Chemkin 2024 R2.03
EnSight 2024 R2
EnSight 2024 R2.01
EnSight 2024 R2.02
EnSight 2024 R2.03
FENSAP-ICE 2024 R2
FENSAP-ICE 2024 R2.01
FENSAP-ICE 2024 R2.02
FENSAP-ICE 2024 R2.03
Fluent (includes CFD-Post) 2024 R2
Fluent (includes CFD-Post) 2024 R2.01
Fluent (includes CFD-Post) 2024 R2.02
Fluent (includes CFD-Post) 2024 R2.03
Polyflow (includes CFD-Post) 2024 R2
Polyflow (includes CFD-Post) 2024 R2.01
Polyflow (includes CFD-Post) 2024 R2.02
Polyflow (includes CFD-Post) 2024 R2.03
Forte (includes EnSight) 2024 R2
Forte (includes EnSight) 2024 R2.01
Forte (includes EnSight) 2024 R2.02
Forte (includes EnSight) 2024 R2.03
ICEM CFD 2024 R2
ICEM CFD 2024 R2.01
ICEM CFD 2024 R2.02
ICEM CFD 2024 R2.03
TurboGrid 2024 R2
TurboGrid 2024 R2.01
TurboGrid 2024 R2.02
TurboGrid 2024 R2.03
Speos 2024 R2
Speos 2024 R2.01
Speos 2024 R2.02
Speos 2024 R2.03
Speos HPC 2024 R2
Speos HPC 2024 R2.01
Speos HPC 2024 R2.02
Speos HPC 2024 R2.03
optiSLang 2024 R2
optiSLang 2024 R2.01
optiSLang 2024 R2.02
optiSLang 2024 R2.03
Remote Solve Manager Standalone Services 2024 R2
Remote Solve Manager Standalone Services 2024 R2.01
Remote Solve Manager Standalone Services 2024 R2.02
Remote Solve Manager Standalone Services 2024 R2.03
Additive 2024 R2
Additive 2024 R2.01
Additive 2024 R2.02
Additive 2024 R2.03
Aqwa 2024 R2
Aqwa 2024 R2.01
Aqwa 2024 R2.02
Aqwa 2024 R2.03
Autodyn 2024 R2
Autodyn 2024 R2.01
Autodyn 2024 R2.02
Autodyn 2024 R2.03
Customization Files for User Programmable Features 2024 R2
Customization Files for User Programmable Features 2024 R2.01
Customization Files for User Programmable Features 2024 R2.02
Customization Files for User Programmable Features 2024 R2.03
LS-DYNA 2024 R2
LS-DYNA 2024 R2.01
LS-DYNA 2024 R2.02
LS-DYNA 2024 R2.03
Mechanical Products 2024 R2
Mechanical Products 2024 R2.01
Mechanical Products 2024 R2.02
Mechanical Products 2024 R2.03
Motion 2024 R2
Motion 2024 R2.01
Motion 2024 R2.02
Motion 2024 R2.03
Sherlock 2024 R2
Sherlock 2024 R2.01
Sherlock 2024 R2.02
Sherlock 2024 R2.03
Sound - SAS 2024 R2
Sound - SAS 2024 R2.01
Sound - SAS 2024 R2.02
Sound - SAS 2024 R2.03
ACIS Geometry Interface 2024 R2
ACIS Geometry Interface 2024 R2.01
ACIS Geometry Interface 2024 R2.02
ACIS Geometry Interface 2024 R2.03
AutoCAD Geometry Interface 2024 R2
AutoCAD Geometry Interface 2024 R2.01
AutoCAD Geometry Interface 2024 R2.02
AutoCAD Geometry Interface 2024 R2.03
Catia, Version 4 Geometry Interface 2024 R2
Catia, Version 4 Geometry Interface 2024 R2.01
Catia, Version 4 Geometry Interface 2024 R2.02
Catia, Version 4 Geometry Interface 2024 R2.03
Catia, Version 5 Geometry Interface 2024 R2
Catia, Version 5 Geometry Interface 2024 R2.01
Catia, Version 5 Geometry Interface 2024 R2.02
Catia, Version 5 Geometry Interface 2024 R2.03
Catia, Version 6 Geometry Interface 2024 R2
Catia, Version 6 Geometry Interface 2024 R2.01
Catia, Version 6 Geometry Interface 2024 R2.02
Catia, Version 6 Geometry Interface 2024 R2.03
Creo Elements/Direct Modeling Geometry Interface 2024 R2
Creo Elements/Direct Modeling Geometry Interface 2024 R2.01
Creo Elements/Direct Modeling Geometry Interface 2024 R2.02
Creo Elements/Direct Modeling Geometry Interface 2024 R2.03
Creo Parametric Geometry Interface 2024 R2
Creo Parametric Geometry Interface 2024 R2.01
Creo Parametric Geometry Interface 2024 R2.02
Creo Parametric Geometry Interface 2024 R2.03
Inventor Geometry Interface 2024 R2
Inventor Geometry Interface 2024 R2.01
Inventor Geometry Interface 2024 R2.02
Inventor Geometry Interface 2024 R2.03
JTOpen Geometry Interface 2024 R2
JTOpen Geometry Interface 2024 R2.01
JTOpen Geometry Interface 2024 R2.02
JTOpen Geometry Interface 2024 R2.03
NX Geometry Interface 2024 R2
NX Geometry Interface 2024 R2.01
NX Geometry Interface 2024 R2.02
NX Geometry Interface 2024 R2.03
Parasolid Geometry Interface 2024 R2
Parasolid Geometry Interface 2024 R2.01
Parasolid Geometry Interface 2024 R2.02
Parasolid Geometry Interface 2024 R2.03
Solid Edge Geometry Interface 2024 R2
Solid Edge Geometry Interface 2024 R2.01
Solid Edge Geometry Interface 2024 R2.02
Solid Edge Geometry Interface 2024 R2.03
SOLIDWORKS Geometry Interface 2024 R2
SOLIDWORKS Geometry Interface 2024 R2.01
SOLIDWORKS Geometry Interface 2024 R2.02
SOLIDWORKS Geometry Interface 2024 R2.03
***** MAPDL COMMAND LINE ARGUMENTS *****
BATCH MODE REQUESTED (-b) = NOLIST
INPUT FILE COPY MODE (-c) = COPY
DISTRIBUTED MEMORY PARALLEL REQUESTED
4 PARALLEL PROCESSES REQUESTED WITH SINGLE THREAD PER PROCESS
TOTAL OF 4 CORES REQUESTED
INPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr81BD\dummy.dat
OUTPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr81BD\solve.out
START-UP FILE MODE = NOREAD
STOP FILE MODE = NOREAD
RELEASE= 2024 R2 BUILD= 24.2 UP20240603 VERSION=WINDOWS x64
CURRENT JOBNAME=file0 17:42:44 JAN 08, 2025 CP= 0.094
PARAMETER _DS_PROGRESS = 999.0000000
/INPUT FILE= ds.dat LINE= 0
*** NOTE *** CP = 0.344 TIME= 17:42:45
The /CONFIG,NOELDB command is not valid in a distributed memory
parallel solution. Command is ignored.
*GET _WALLSTRT FROM ACTI ITEM=TIME WALL VALUE= 17.7125000
TITLE=
wbnew--Modal Campbell
ACT Extensions:
LSDYNA, 2024.2
5f463412-bd3e-484b-87e7-cbc0a665e474, wbex
/COM, ANSYSMotion, 2024.2
20180725-3f81-49eb-9f31-41364844c769, wbex
SET PARAMETER DIMENSIONS ON _WB_PROJECTSCRATCH_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_PROJECTSCRATCH_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr81BD\
SET PARAMETER DIMENSIONS ON _WB_SOLVERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_SOLVERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-6\MECH\
SET PARAMETER DIMENSIONS ON _WB_USERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_USERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\user_files\
--- Data in consistent NMM units. See Solving Units in the help system for more
MPA UNITS SPECIFIED FOR INTERNAL
LENGTH = MILLIMETERS (mm)
MASS = TONNE (Mg)
TIME = SECONDS (sec)
TEMPERATURE = CELSIUS (C)
TOFFSET = 273.0
FORCE = NEWTON (N)
HEAT = MILLIJOULES (mJ)
INPUT UNITS ARE ALSO SET TO MPA
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:45 JAN 08, 2025 CP= 0.359
wbnew--Modal Campbell
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
*********** Nodes for the whole assembly ***********
*********** Nodes for all Remote Points ***********
*********** Elements for Body 1 'Surface Body1' ***********
*********** Elements for Body 2 'Surface Body2' ***********
*********** Elements for Body 3 'Surface Body3' ***********
*********** Elements for Body 4 'Surface Body4' ***********
*********** Send User Defined Coordinate System(s) ***********
*********** Set Reference Temperature ***********
*********** Send Materials ***********
*********** Send Sheet Properties ***********
*********** Send General Axisymmetric Properties "General Axisymmetric" *******
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Element Component ***********
*********** Send Named Selection as Node Component ***********
*********** Create Remote Point "PointMass_RemotePoint" ***********
*********** Create Remote Point "RemotePoint_Bearing1" ***********
*********** Create Remote Point "RemotePoint_Bearing2" ***********
*********** Create Remote Point "RemotePoint_FreeStanding1" ***********
*********** Create Remote Point "RemotePoint_FreeStanding2" ***********
*********** Create Remote Point "PointMass_RemotePoint2" ***********
*********** Create Bearing Connection "RemotePoint_FreeStanding1 To Multiple" *
Real Constant Set For Above Bearing Connection Is 13
*********** Create Bearing Connection "RemotePoint_FreeStanding2 To Multiple" *
Real Constant Set For Above Bearing Connection Is 14
*********** Construct Remote Mass Using Remote Attachment ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*** Create a component for all remote displacements ***
*********** Define Rotational Velocity ***********
***** ROUTINE COMPLETED ***** CP = 0.531
--- Number of total nodes = 7698
--- Number of contact elements = 142
--- Number of spring elements = 0
--- Number of bearing elements = 0
--- Number of solid elements = 2428
--- Number of condensed parts = 0
--- Number of total elements = 2577
*GET _WALLBSOL FROM ACTI ITEM=TIME WALL VALUE= 17.7125000
***** MAPDL SOLUTION ROUTINE *****
PERFORM A MODAL ANALYSIS
THIS WILL BE A NEW ANALYSIS
PARAMETER _THICKRATIO = 0.000000000
USE QRDAMP MODE EXTRACTION METHOD
EXTRACT 9 MODES
COMPUTE COMPLEX MODE SHAPES: YES
NORMALIZE THE MODE SHAPES TO THE MASS MATRIX
QRDAMP EIGENSOLVER OPTIONS
REUSE EIGENMODES FROM MODESYM FILE: Yes
SYMMETRIZE CONTACT STIFFNESS MATRIX AT ELEMENT LEVEL
CORIOLIS IN STATIONARY REFERENCE FRAME: GYROSCOPIC DAMPING MATRIX WILL BE CALCULATED
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
PRINTOUT RESUMED BY /GOP
WRITE MISC ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR THE ENTITIES DEFINED BY COMPONENT _ELMISC
EXPAND ALL EXTRACTED MODES
CALCULATE ELEMENT RESULTS AND NODAL DOF SOLUTION
CALCULATE STRESSES IN ANY DOWNSTREAM MODE SUPERPOSITION EXPANSION PASS
DO NOT COMBINE ELEMENT SAVE DATA FILES (.esav) AFTER DISTRIBUTED PARALLEL SOLUTION
DO NOT COMBINE ELEMENT MATRIX FILES (.emat) AFTER DISTRIBUTED PARALLEL SOLUTION
DO NOT COMBINE ASSEMBLED MATRIX FILES (.full) AFTER DISTRIBUTED PARALLEL SOLUTION
---------------- Campbell diagram point 1 ----------------
CMOMEGA on component= CM_ROTVELCITY137
OMEGAX, OMEGAY, OMEGAZ= 0.10472E-14 0.0000 0.0000
X1, Y1, Z1= 0.0000 0.0000 0.0000
*GET ANSINTER_ FROM ACTI ITEM=INT VALUE= 0.00000000
*IF ANSINTER_ ( = 0.00000 ) NE
0 ( = 0.00000 ) THEN
*ENDIF
*** NOTE *** CP = 0.594 TIME= 17:42:45
The automatic domain decomposition logic has selected the MESH domain
decomposition method with 4 processes per solution.
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 0.609 TIME= 17:42:45
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** NOTE *** CP = 0.656 TIME= 17:42:45
The model data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr8
81BD\file0.err ) for these warning messages.
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID272. KEYOPT(6) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID272. KEYOPT(6) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 3 IS SOLID272. KEYOPT(6) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 4 IS SOLID272. KEYOPT(6) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:45 JAN 08, 2025 CP= 0.656
wbnew--Modal Campbell
S O L U T I O N O P T I O N S
PROBLEM DIMENSIONALITY. . . . . . . . . . . . .3-D
DEGREES OF FREEDOM. . . . . . UX UY UZ ROTX ROTY ROTZ
ANALYSIS TYPE . . . . . . . . . . . . . . . . .MODAL
EXTRACTION METHOD. . . . . . . . . . . . . .QRDAMP
QRDAMP BASE EIGENSOLVER. . . . . . . . . . .BLOCK LANCZOS
COMPLEX SOLUTION OUTPUT. . . . . . . . . . .ON
OFFSET TEMPERATURE FROM ABSOLUTE ZERO . . . . . 273.15
NUMBER OF MODES TO EXTRACT. . . . . . . . . . . 9
GLOBALLY ASSEMBLED MATRIX . . . . . . . . . . .UNSYMMETRIC
NUMBER OF MODES TO EXPAND . . . . . . . . . . .ALL
ELEMENT RESULTS CALCULATION . . . . . . . . . .ON
*** WARNING *** CP = 0.656 TIME= 17:42:45
Material number 13 (used by element 2435) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 0.672 TIME= 17:42:45
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr8
81BD\file0.err ) for these warning messages.
*** NOTE *** CP = 0.688 TIME= 17:42:45
Internal nodes from 7699 to 7702 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
*** NOTE *** CP = 0.703 TIME= 17:42:45
Internal nodes from 7699 to 7702 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
*** NOTE *** CP = 0.750 TIME= 17:42:45
Rigid-constraint surface identified by real constant set 5 and contact
element type 5 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7689 which connects to other
element 2583. Internal MPC will be built.
This pair will be merged with other pair defined by real constant set
11.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** NOTE *** CP = 0.750 TIME= 17:42:45
Rigid-constraint surface identified by real constant set 7 and contact
element type 7 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7690 which connects to other
element 2435. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** NOTE *** CP = 0.750 TIME= 17:42:45
Rigid-constraint surface identified by real constant set 9 and contact
element type 9 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7691 which connects to other
element 2436. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** NOTE *** CP = 0.750 TIME= 17:42:45
Rigid-constraint surface identified by real constant set 11 and
contact element type 11 has been set up. The degrees of freedom of
rigid surface are driven by the pilot node 7694. Internal MPC will be
built.
This pair will be merged with other pair defined by real constant set
5.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
Please verify constraints (including rotational degrees of freedom)
on the pilot node by yourself.
****************************************
*** NOTE *** CP = 0.766 TIME= 17:42:45
Internal nodes from 7699 to 7702 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
D I S T R I B U T E D D O M A I N D E C O M P O S E R
...Number of elements: 2577
...Number of nodes: 7702
...Decompose to 4 CPU domains
...Element load balance ratio = 1.002
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 1
THERMAL STRAINS INCLUDED IN THE LOAD VECTOR . . YES
CORIOLIS EFFECT IN STATIONARY REF. FRAME . . . ON
REUSE EIGENMODES FOR QRDAMP SOLVER. . . . . . .YES
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
MISC ALL _ELMISC
*********** PRECISE MASS SUMMARY ***********
TOTAL RIGID BODY MASS MATRIX ABOUT ORIGIN
Translational mass | Coupled translational/rotational mass
0.38553E-02 0.0000 0.0000 | 0.0000 -0.10217E-17 0.11484E-12
0.0000 0.38553E-02 0.13172E-20 | 0.19954E-17 -0.13482E-18 0.62268
0.0000 0.26458E-20 0.38553E-02 | -0.11484E-12 -0.62268 0.62267E-18
------------------------------------------ | ------------------------------------------
| Rotational mass (inertia)
| 2.7510 0.52043E-10 0.43541E-15
| 0.52043E-10 149.62 -0.11702E-15
| 0.42266E-15 0.47130E-16 149.62
TOTAL MASS = 0.38553E-02
The mass principal axes coincide with the global Cartesian axes
CENTER OF MASS (X,Y,Z)= 161.51 -0.29787E-10 -0.26502E-15
TOTAL INERTIA ABOUT CENTER OF MASS
2.7510 0.33495E-10 0.11312E-15
0.33495E-10 49.050 -0.11702E-15
0.11312E-15 -0.11702E-15 49.050
The inertia principal axes coincide with the global Cartesian axes
*** MASS SUMMARY BY ELEMENT TYPE ***
TYPE MASS
1 0.274552E-03
2 0.769219E-03
3 0.621633E-03
4 0.788881E-03
15 0.140100E-02
Range of element maximum matrix coefficients in global coordinates
Maximum = 14269624.8 at element 1019.
Minimum = 35030 at element 2436.
*** ELEMENT MATRIX FORMULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 441 SOLID272 0.172 0.000390
2 580 SOLID272 0.234 0.000404
3 810 SOLID272 0.578 0.000714
4 597 SOLID272 0.266 0.000445
5 43 CONTA175 0.000 0.000000
6 1 TARGE170 0.000 0.000000
7 28 CONTA175 0.000 0.000000
8 1 TARGE170 0.000 0.000000
9 28 CONTA175 0.000 0.000000
10 1 TARGE170 0.000 0.000000
11 43 CONTA175 0.000 0.000000
12 1 TARGE170 0.000 0.000000
13 1 COMBI214 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 MASS21 0.000 0.000000
Time at end of element matrix formulation CP = 1.8125.
*** GYROSCOPIC DAMPING MATRIX CALCULATED FOR LISTED ELEMENTS:
TYPE NUMBER ENAME
1 441 SOLID272
2 580 SOLID272
3 810 SOLID272
4 597 SOLID272
15 1 MASS21
BLOCK LANCZOS CALCULATION OF UP TO 9 EIGENVECTORS.
NUMBER OF EQUATIONS = 22781
MAXIMUM WAVEFRONT = 263
MAXIMUM MODES STORED = 9
MINIMUM EIGENVALUE = 0.00000E+00
MAXIMUM EIGENVALUE = 0.10000E+31
Memory allocated on only this MPI rank (rank 0)
-------------------------------------------------------------------
Equation solver memory allocated = 14.976 MB
Equation solver memory required for in-core mode = 14.280 MB
Equation solver memory required for out-of-core mode = 8.691 MB
Total (solver and non-solver) memory allocated = 488.140 MB
Total memory summed across all MPI ranks on this machines
-------------------------------------------------------------------
Equation solver memory allocated = 65.311 MB
Equation solver memory required for in-core mode = 62.275 MB
Equation solver memory required for out-of-core mode = 36.311 MB
Total (solver and non-solver) memory allocated = 1140.397 MB
*** NOTE *** CP = 2.328 TIME= 17:42:46
The Distributed Sparse Matrix Solver used by the Block Lanczos
eigensolver is currently running in the in-core memory mode. This
memory mode uses the most amount of memory in order to avoid using the
hard drive as much as possible, which most often results in the
fastest solution time. This mode is recommended if enough physical
memory is present to accommodate all of the solver data.
Process memory required for in-core LANCZOS Workspace = 24.423317 MB
Process memory required for out-of-core LANCZOS Workspace = 1.053017 MB
Total memory required for in-core LANCZOS Workspace = 92.959808 MB
Total memory required for out-of-core LANCZOS Workspace = 4.099365 MB
Lanczos Memory Mode : INCORE
>> Shift # 1 : | : 9 Eigenvalues Converged
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:46 JAN 08, 2025 CP= 2.641
wbnew--Modal Campbell
*** UNDAMPED FREQUENCIES FROM BLOCK LANCZOS ITERATION ***
MODE FREQUENCY (HERTZ)
1 190.0656343486
2 209.1598339837
3 639.9190075061
4 660.1934103669
5 736.4670026947
6 812.1381924377
7 991.3423824664
8 1784.269371768
9 1784.805960465
Time at end of state-space matrix formulation CP = 2.671875.
***** DAMPED FREQUENCIES FROM REDUCED DAMPED EIGENSOLVER *****
COMPLEX FREQUENCY (HERTZ)
MODE STABILITY FREQUENCY MODAL DAMPING RATIO
1 0.0000000 190.06563 j 0.0000000
2 0.0000000 209.15983 j 0.0000000
3 0.0000000 639.91901 j 0.0000000
4 0.0000000 660.19341 j 0.0000000
5 0.0000000 736.46700 j 0.0000000
6 0.0000000 812.13819 j 0.0000000
7 0.0000000 991.34238 j 0.0000000
8 0.0000000 1784.2694 j 0.0000000
9 0.0000000 1784.8060 j 0.0000000
Time at end of eigenproblem resolution CP = 2.671875.
Time at end of mode shape normalization CP = 2.703125.
*** ELEMENT RESULT CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 441 SOLID272 0.062 0.000142
2 580 SOLID272 0.062 0.000108
3 810 SOLID272 0.188 0.000231
4 597 SOLID272 0.156 0.000262
5 43 CONTA175 0.000 0.000000
7 28 CONTA175 0.000 0.000000
9 28 CONTA175 0.000 0.000000
11 43 CONTA175 0.000 0.000000
13 1 COMBI214 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 MASS21 0.000 0.000000
*** NODAL LOAD CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 441 SOLID272 0.000 0.000000
2 580 SOLID272 0.000 0.000000
3 810 SOLID272 0.000 0.000000
4 597 SOLID272 0.000 0.000000
5 43 CONTA175 0.000 0.000000
7 28 CONTA175 0.000 0.000000
9 28 CONTA175 0.000 0.000000
11 43 CONTA175 0.000 0.000000
13 1 COMBI214 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 MASS21 0.000 0.000000
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:49 JAN 08, 2025 CP= 4.844
wbnew--Modal Campbell
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 190.066 0.52613E-02 0.11567E-05 0.315019 0.133790E-11 0.328178E-01 0.347031E-09
2 209.160 0.47810E-02 -0.10652E-05 0.290107 0.113466E-11 0.606502E-01 0.294313E-09
3 639.919 0.15627E-02 0.36718E-05 1.000000 0.134819E-10 0.391351 0.349699E-08
4 660.193 0.15147E-02 0.26669E-05 0.726333 0.711251E-11 0.565816 0.184487E-08
5 736.467 0.13578E-02 0.26633E-05 0.725356 0.709339E-11 0.739811 0.183991E-08
6 812.138 0.12313E-02 -0.30911E-05 0.841853 0.955483E-11 0.974184 0.247837E-08
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.974184 0.00000
8 1784.27 0.56045E-03 -0.77988E-06 0.212400 0.608220E-12 0.989104 0.157763E-09
9 1784.81 0.56028E-03 0.66650E-06 0.181520 0.444221E-12 1.00000 0.115224E-09
-----------------------------------------------------------------------------------------------------------------
sum 0.407676E-10 0.105745E-07
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 190.066 0.52613E-02 0.23951E-01 0.696558 0.573664E-03 0.150935 0.148799
2 209.160 0.47810E-02 -0.23245E-01 0.676008 0.540313E-03 0.293095 0.140149
3 639.919 0.15627E-02 0.23857E-01 0.693810 0.569146E-03 0.442841 0.147627
4 660.193 0.15147E-02 0.27745E-01 0.806894 0.769796E-03 0.645380 0.199673
5 736.467 0.13578E-02 0.12841E-01 0.373454 0.164898E-03 0.688765 0.427720E-01
6 812.138 0.12313E-02 -0.34385E-01 1.000000 0.118234E-02 0.999847 0.306680
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999847 0.00000
8 1784.27 0.56045E-03 -0.43658E-03 0.012697 0.190599E-06 0.999897 0.494383E-04
9 1784.81 0.56028E-03 0.62489E-03 0.018173 0.390493E-06 1.00000 0.101288E-03
-----------------------------------------------------------------------------------------------------------------
sum 0.380074E-02 0.985851
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 190.066 0.52613E-02 0.23951E-01 0.696558 0.573664E-03 0.150935 0.148799
2 209.160 0.47810E-02 0.23245E-01 0.676008 0.540313E-03 0.293095 0.140149
3 639.919 0.15627E-02 0.23857E-01 0.693810 0.569146E-03 0.442841 0.147627
4 660.193 0.15147E-02 0.27745E-01 0.806894 0.769796E-03 0.645380 0.199673
5 736.467 0.13578E-02 -0.12841E-01 0.373454 0.164898E-03 0.688765 0.427720E-01
6 812.138 0.12313E-02 0.34385E-01 1.000000 0.118234E-02 0.999847 0.306680
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999847 0.00000
8 1784.27 0.56045E-03 -0.43658E-03 0.012697 0.190599E-06 0.999897 0.494383E-04
9 1784.81 0.56028E-03 -0.62489E-03 0.018173 0.390493E-06 1.00000 0.101288E-03
-----------------------------------------------------------------------------------------------------------------
sum 0.380074E-02 0.985851
-----------------------------------------------------------------------------------------------------------------
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:49 JAN 08, 2025 CP= 4.844
wbnew--Modal Campbell
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 190.066 0.52613E-02 0.0000 0.000000 0.00000 0.00000 0.00000
2 209.160 0.47810E-02 0.0000 0.000000 0.00000 0.00000 0.00000
3 639.919 0.15627E-02 0.0000 0.000000 0.00000 0.00000 0.00000
4 660.193 0.15147E-02 0.0000 0.000000 0.00000 0.00000 0.00000
5 736.467 0.13578E-02 0.0000 0.000000 0.00000 0.00000 0.00000
6 812.138 0.12313E-02 0.0000 0.000000 0.00000 0.00000 0.00000
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.00000 0.00000
8 1784.27 0.56045E-03 0.0000 0.000000 0.00000 0.00000 0.00000
9 1784.81 0.56028E-03 0.0000 0.000000 0.00000 0.00000 0.00000
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 190.066 0.52613E-02 0.0000 0.000000 0.00000 0.00000 0.00000
2 209.160 0.47810E-02 0.0000 0.000000 0.00000 0.00000 0.00000
3 639.919 0.15627E-02 0.0000 0.000000 0.00000 0.00000 0.00000
4 660.193 0.15147E-02 0.0000 0.000000 0.00000 0.00000 0.00000
5 736.467 0.13578E-02 0.0000 0.000000 0.00000 0.00000 0.00000
6 812.138 0.12313E-02 0.0000 0.000000 0.00000 0.00000 0.00000
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.00000 0.00000
8 1784.27 0.56045E-03 0.0000 0.000000 0.00000 0.00000 0.00000
9 1784.81 0.56028E-03 0.0000 0.000000 0.00000 0.00000 0.00000
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 190.066 0.52613E-02 0.0000 0.000000 0.00000 0.00000 0.00000
2 209.160 0.47810E-02 0.0000 0.000000 0.00000 0.00000 0.00000
3 639.919 0.15627E-02 0.0000 0.000000 0.00000 0.00000 0.00000
4 660.193 0.15147E-02 0.0000 0.000000 0.00000 0.00000 0.00000
5 736.467 0.13578E-02 0.0000 0.000000 0.00000 0.00000 0.00000
6 812.138 0.12313E-02 0.0000 0.000000 0.00000 0.00000 0.00000
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.00000 0.00000
8 1784.27 0.56045E-03 0.0000 0.000000 0.00000 0.00000 0.00000
9 1784.81 0.56028E-03 0.0000 0.000000 0.00000 0.00000 0.00000
*** MAPDL BINARY FILE STATISTICS
BUFFER SIZE USED= 16384
19.812 MB WRITTEN ON ELEMENT MATRIX FILE: file0.emat
7.812 MB WRITTEN ON ELEMENT SAVED DATA FILE: file0.esav
10.250 MB WRITTEN ON ASSEMBLED MATRIX FILE: file0.full
1.188 MB WRITTEN ON MODAL MATRIX FILE: file0.mode
1.562 MB WRITTEN ON RESULTS FILE: file0.rst
*************** Write FE CONNECTORS *********
WRITE OUT CONSTRAINT EQUATIONS TO FILE= file.ce
---------------- Campbell diagram point 2 ----------------
CMOMEGA on component= CM_ROTVELCITY137
OMEGAX, OMEGAY, OMEGAZ= 5236.0 0.0000 0.0000
X1, Y1, Z1= 0.0000 0.0000 0.0000
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 4.969 TIME= 17:42:49
Material number 13 (used by element 2435) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 4.984 TIME= 17:42:49
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr8
81BD\file0.err ) for these warning messages.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:50 JAN 08, 2025 CP= 5.109
wbnew--Modal Campbell
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 2
THERMAL STRAINS INCLUDED IN THE LOAD VECTOR . . YES
CORIOLIS EFFECT IN STATIONARY REF. FRAME . . . ON
REUSE EIGENMODES FOR QRDAMP SOLVER. . . . . . .YES
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
MISC ALL _ELMISC
*** GYROSCOPIC DAMPING MATRIX CALCULATED FOR LISTED ELEMENTS:
TYPE NUMBER ENAME
1 441 SOLID272
2 580 SOLID272
3 810 SOLID272
4 597 SOLID272
15 1 MASS21
*** WARNING *** CP = 5.750 TIME= 17:42:50
The file0.modesym file does exist and is being used for the QR damp
eigensolution since the ReuseKey is set to ON on the QRDOPT command.
Time at end of state-space matrix formulation CP = 5.765625.
***** DAMPED FREQUENCIES FROM REDUCED DAMPED EIGENSOLVER *****
COMPLEX FREQUENCY (HERTZ)
MODE STABILITY FREQUENCY MODAL DAMPING RATIO
1 0.0000000 169.34208 j 0.0000000
2 0.0000000 232.82032 j 0.0000000
3 0.0000000 629.72205 j 0.0000000
4 0.0000000 657.09846 j 0.0000000
5 0.0000000 755.96914 j 0.0000000
6 0.0000000 814.38603 j 0.0000000
7 0.0000000 991.34238 j 0.0000000
8 0.0000000 1766.8315 j 0.0000000
9 0.0000000 1802.6778 j 0.0000000
Time at end of eigenproblem resolution CP = 5.765625.
Time at end of mode shape normalization CP = 5.765625.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:53 JAN 08, 2025 CP= 7.953
wbnew--Modal Campbell
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 169.342 0.59052E-02 0.88522E-06 0.257041 0.783611E-12 0.208125E-01 0.203256E-09
2 232.820 0.42952E-02 -0.89446E-06 0.259726 0.800065E-12 0.420620E-01 0.207524E-09
3 629.722 0.15880E-02 0.28870E-05 0.838314 0.833503E-11 0.263438 0.216198E-08
4 657.098 0.15218E-02 0.34439E-05 1.000000 0.118603E-10 0.578443 0.307636E-08
5 755.969 0.13228E-02 0.24496E-05 0.711281 0.600034E-11 0.737810 0.155639E-08
6 814.386 0.12279E-02 -0.30558E-05 0.887324 0.933810E-11 0.985827 0.242215E-08
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.985827 0.00000
8 1766.83 0.56598E-03 -0.57294E-06 0.166364 0.328255E-12 0.994546 0.851443E-10
9 1802.68 0.55473E-03 0.45316E-06 0.131585 0.205356E-12 1.00000 0.532662E-10
-----------------------------------------------------------------------------------------------------------------
sum 0.376510E-10 0.976608E-08
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 169.342 0.59052E-02 0.19193E-01 0.564651 0.368384E-03 0.110343 0.955531E-01
2 232.820 0.42952E-02 -0.18741E-01 0.551345 0.351226E-03 0.215546 0.911026E-01
3 629.722 0.15880E-02 0.17052E-01 0.501654 0.290770E-03 0.302640 0.754211E-01
4 657.098 0.15218E-02 0.32294E-01 0.950051 0.104288E-02 0.615016 0.270507
5 755.969 0.13228E-02 0.11385E-01 0.334930 0.129613E-03 0.653839 0.336196E-01
6 814.386 0.12279E-02 -0.33992E-01 1.000000 0.115542E-02 0.999924 0.299698
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999924 0.00000
8 1766.83 0.56598E-03 -0.34726E-03 0.010216 0.120592E-06 0.999960 0.312795E-04
9 1802.68 0.55473E-03 0.36634E-03 0.010777 0.134203E-06 1.00000 0.348101E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.333855E-02 0.865968
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 169.342 0.59052E-02 0.19193E-01 0.564651 0.368384E-03 0.110343 0.955531E-01
2 232.820 0.42952E-02 0.18741E-01 0.551345 0.351226E-03 0.215546 0.911026E-01
3 629.722 0.15880E-02 0.17052E-01 0.501654 0.290770E-03 0.302640 0.754211E-01
4 657.098 0.15218E-02 0.32294E-01 0.950051 0.104288E-02 0.615016 0.270507
5 755.969 0.13228E-02 -0.11385E-01 0.334930 0.129613E-03 0.653839 0.336196E-01
6 814.386 0.12279E-02 0.33992E-01 1.000000 0.115542E-02 0.999924 0.299698
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999924 0.00000
8 1766.83 0.56598E-03 -0.34726E-03 0.010216 0.120592E-06 0.999960 0.312796E-04
9 1802.68 0.55473E-03 -0.36634E-03 0.010777 0.134203E-06 1.00000 0.348101E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.333855E-02 0.865968
-----------------------------------------------------------------------------------------------------------------
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:53 JAN 08, 2025 CP= 7.953
wbnew--Modal Campbell
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 169.342 0.59052E-02 0.55846E-06 0.567239 0.311872E-12 0.101355 0.808947E-10
2 232.820 0.42952E-02 -0.82728E-06 0.840295 0.684398E-12 0.323778 0.177522E-09
3 629.722 0.15880E-02 0.98452E-06 1.000000 0.969270E-12 0.638781 0.251413E-09
4 657.098 0.15218E-02 0.63675E-07 0.064676 0.405444E-14 0.640099 0.105166E-11
5 755.969 0.13228E-02 0.69326E-06 0.704162 0.480607E-12 0.796291 0.124662E-09
6 814.386 0.12279E-02 -0.33299E-06 0.338228 0.110883E-12 0.832327 0.287613E-10
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.832327 0.00000
8 1766.83 0.56598E-03 -0.48754E-06 0.495207 0.237694E-12 0.909575 0.616542E-10
9 1802.68 0.55473E-03 0.52748E-06 0.535780 0.278239E-12 1.00000 0.721708E-10
-----------------------------------------------------------------------------------------------------------------
sum 0.307702E-11 0.798130E-09
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 169.342 0.59052E-02 0.12977E-01 0.839653 0.168397E-03 0.360343 0.436796E-01
2 232.820 0.42952E-02 -0.15455E-01 1.000000 0.238856E-03 0.871456 0.619554E-01
3 629.722 0.15880E-02 0.65892E-02 0.426349 0.434177E-04 0.964363 0.112619E-01
4 657.098 0.15218E-02 0.25836E-02 0.167172 0.667522E-05 0.978646 0.173145E-02
5 755.969 0.13228E-02 0.15486E-02 0.100204 0.239830E-05 0.983778 0.622082E-03
6 814.386 0.12279E-02 -0.26909E-02 0.174109 0.724070E-05 0.999272 0.187812E-02
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999272 0.00000
8 1766.83 0.56598E-03 -0.51813E-03 0.033525 0.268455E-06 0.999847 0.696330E-04
9 1802.68 0.55473E-03 0.26758E-03 0.017314 0.715997E-07 1.00000 0.185718E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.467325E-03 0.121217
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 169.342 0.59052E-02 -0.12977E-01 0.839653 0.168397E-03 0.360343 0.436796E-01
2 232.820 0.42952E-02 -0.15455E-01 1.000000 0.238856E-03 0.871456 0.619554E-01
3 629.722 0.15880E-02 -0.65892E-02 0.426349 0.434177E-04 0.964363 0.112619E-01
4 657.098 0.15218E-02 -0.25836E-02 0.167172 0.667522E-05 0.978646 0.173145E-02
5 755.969 0.13228E-02 0.15486E-02 0.100204 0.239830E-05 0.983778 0.622082E-03
6 814.386 0.12279E-02 -0.26909E-02 0.174109 0.724070E-05 0.999272 0.187812E-02
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999272 0.00000
8 1766.83 0.56598E-03 0.51813E-03 0.033525 0.268455E-06 0.999847 0.696330E-04
9 1802.68 0.55473E-03 0.26758E-03 0.017314 0.715997E-07 1.00000 0.185718E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.467325E-03 0.121217
-----------------------------------------------------------------------------------------------------------------
---------------- Campbell diagram point 3 ----------------
CMOMEGA on component= CM_ROTVELCITY137
OMEGAX, OMEGAY, OMEGAZ= 10472. 0.0000 0.0000
X1, Y1, Z1= 0.0000 0.0000 0.0000
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 8.062 TIME= 17:42:53
Material number 13 (used by element 2435) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 8.062 TIME= 17:42:53
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr8
81BD\file0.err ) for these warning messages.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:53 JAN 08, 2025 CP= 8.219
wbnew--Modal Campbell
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 3
THERMAL STRAINS INCLUDED IN THE LOAD VECTOR . . YES
CORIOLIS EFFECT IN STATIONARY REF. FRAME . . . ON
REUSE EIGENMODES FOR QRDAMP SOLVER. . . . . . .YES
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
MISC ALL _ELMISC
*** GYROSCOPIC DAMPING MATRIX CALCULATED FOR LISTED ELEMENTS:
TYPE NUMBER ENAME
1 441 SOLID272
2 580 SOLID272
3 810 SOLID272
4 597 SOLID272
15 1 MASS21
*** WARNING *** CP = 8.734 TIME= 17:42:53
The file0.modesym file does exist and is being used for the QR damp
eigensolution since the ReuseKey is set to ON on the QRDOPT command.
Time at end of state-space matrix formulation CP = 8.765625.
***** DAMPED FREQUENCIES FROM REDUCED DAMPED EIGENSOLVER *****
COMPLEX FREQUENCY (HERTZ)
MODE STABILITY FREQUENCY MODAL DAMPING RATIO
1 0.0000000 145.22813 j 0.0000000
2 0.0000000 264.96486 j 0.0000000
3 0.0000000 607.21391 j 0.0000000
4 0.0000000 654.00922 j 0.0000000
5 0.0000000 800.21313 j 0.0000000
6 0.0000000 820.99584 j 0.0000000
7 0.0000000 991.34238 j 0.0000000
8 0.0000000 1749.5152 j 0.0000000
9 0.0000000 1821.3096 j 0.0000000
Time at end of eigenproblem resolution CP = 8.765625.
Time at end of mode shape normalization CP = 8.78125.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:56 JAN 08, 2025 CP= 10.906
wbnew--Modal Campbell
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 145.228 0.68857E-02 0.78045E-06 0.202867 0.609097E-12 0.176453E-01 0.157990E-09
2 264.965 0.37741E-02 -0.91351E-06 0.237455 0.834506E-12 0.418205E-01 0.216458E-09
3 607.214 0.16469E-02 0.20480E-05 0.532343 0.419419E-11 0.163324 0.108791E-08
4 654.009 0.15290E-02 0.38471E-05 1.000000 0.148001E-10 0.592077 0.383891E-08
5 800.213 0.12497E-02 0.21603E-05 0.561550 0.466705E-11 0.727279 0.121056E-08
6 820.996 0.12180E-02 -0.29812E-05 0.774933 0.888778E-11 0.984754 0.230535E-08
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.984754 0.00000
8 1749.52 0.57159E-03 -0.58641E-06 0.152431 0.343882E-12 0.994716 0.891975E-10
9 1821.31 0.54906E-03 0.42708E-06 0.111014 0.182398E-12 1.00000 0.473111E-10
-----------------------------------------------------------------------------------------------------------------
sum 0.345190E-10 0.895369E-08
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 145.228 0.68857E-02 0.17778E-01 0.518900 0.316040E-03 0.102082 0.819757E-01
2 264.965 0.37741E-02 -0.18066E-01 0.527311 0.326368E-03 0.207501 0.846546E-01
3 607.214 0.16469E-02 0.10557E-01 0.308137 0.111445E-03 0.243498 0.289072E-01
4 654.009 0.15290E-02 0.34260E-01 1.000000 0.117375E-02 0.622624 0.304451
5 800.213 0.12497E-02 0.89202E-02 0.260367 0.795692E-04 0.648325 0.206390E-01
6 820.996 0.12180E-02 -0.32993E-01 0.963017 0.108853E-02 0.999927 0.282348
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999927 0.00000
8 1749.52 0.57159E-03 -0.38055E-03 0.011108 0.144819E-06 0.999974 0.375637E-04
9 1821.31 0.54906E-03 0.28475E-03 0.008311 0.810798E-07 1.00000 0.210308E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.309593E-02 0.803034
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 145.228 0.68857E-02 0.17778E-01 0.518900 0.316040E-03 0.102082 0.819757E-01
2 264.965 0.37741E-02 0.18066E-01 0.527311 0.326368E-03 0.207501 0.846546E-01
3 607.214 0.16469E-02 0.10557E-01 0.308137 0.111445E-03 0.243498 0.289072E-01
4 654.009 0.15290E-02 0.34260E-01 1.000000 0.117375E-02 0.622624 0.304451
5 800.213 0.12497E-02 -0.89202E-02 0.260367 0.795692E-04 0.648325 0.206390E-01
6 820.996 0.12180E-02 0.32993E-01 0.963017 0.108853E-02 0.999927 0.282348
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999927 0.00000
8 1749.52 0.57159E-03 -0.38055E-03 0.011108 0.144819E-06 0.999974 0.375637E-04
9 1821.31 0.54906E-03 -0.28475E-03 0.008311 0.810798E-07 1.00000 0.210308E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.309593E-02 0.803034
-----------------------------------------------------------------------------------------------------------------
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:56 JAN 08, 2025 CP= 10.906
wbnew--Modal Campbell
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 145.228 0.68857E-02 0.58602E-06 0.416139 0.343424E-12 0.596446E-01 0.890787E-10
2 264.965 0.37741E-02 -0.10344E-05 0.734539 0.107000E-11 0.245478 0.277541E-09
3 607.214 0.16469E-02 0.14082E-05 1.000000 0.198314E-11 0.589903 0.514395E-09
4 654.009 0.15290E-02 0.58556E-06 0.415809 0.342879E-12 0.649453 0.889375E-10
5 800.213 0.12497E-02 0.10423E-05 0.740170 0.108647E-11 0.838147 0.281812E-09
6 820.996 0.12180E-02 -0.64610E-06 0.458799 0.417444E-12 0.910647 0.108278E-09
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.910647 0.00000
8 1749.52 0.57159E-03 -0.50729E-06 0.360230 0.257344E-12 0.955342 0.667509E-10
9 1821.31 0.54906E-03 0.50709E-06 0.360084 0.257135E-12 1.00000 0.666968E-10
-----------------------------------------------------------------------------------------------------------------
sum 0.575783E-11 0.149349E-08
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 145.228 0.68857E-02 0.14083E-01 0.791251 0.198335E-03 0.284184 0.514449E-01
2 264.965 0.37741E-02 -0.17799E-01 1.000000 0.316789E-03 0.738095 0.821701E-01
3 607.214 0.16469E-02 0.94586E-02 0.531424 0.894647E-04 0.866284 0.232057E-01
4 654.009 0.15290E-02 0.78038E-02 0.438450 0.608990E-04 0.953543 0.157962E-01
5 800.213 0.12497E-02 0.23996E-02 0.134821 0.575818E-05 0.961794 0.149358E-02
6 820.996 0.12180E-02 -0.51238E-02 0.287880 0.262538E-04 0.999412 0.680982E-02
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999412 0.00000
8 1749.52 0.57159E-03 -0.59868E-03 0.033637 0.358422E-06 0.999925 0.929690E-04
9 1821.31 0.54906E-03 0.22819E-03 0.012821 0.520706E-07 1.00000 0.135063E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.697910E-03 0.181027
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 145.228 0.68857E-02 -0.14083E-01 0.791251 0.198335E-03 0.284184 0.514449E-01
2 264.965 0.37741E-02 -0.17799E-01 1.000000 0.316789E-03 0.738095 0.821701E-01
3 607.214 0.16469E-02 -0.94586E-02 0.531424 0.894647E-04 0.866284 0.232057E-01
4 654.009 0.15290E-02 -0.78038E-02 0.438450 0.608990E-04 0.953543 0.157962E-01
5 800.213 0.12497E-02 0.23996E-02 0.134821 0.575818E-05 0.961794 0.149358E-02
6 820.996 0.12180E-02 -0.51238E-02 0.287880 0.262538E-04 0.999412 0.680982E-02
7 991.342 0.10087E-02 0.0000 0.000000 0.00000 0.999412 0.00000
8 1749.52 0.57159E-03 0.59868E-03 0.033637 0.358422E-06 0.999925 0.929690E-04
9 1821.31 0.54906E-03 0.22819E-03 0.012821 0.520706E-07 1.00000 0.135063E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.697910E-03 0.181027
-----------------------------------------------------------------------------------------------------------------
*GET _NMODALSOLPROC FROM ACTI ITEM=NUMC VALUE= 4.00000000
FINISH SOLUTION PROCESSING
***** ROUTINE COMPLETED ***** CP = 11.047
PRINTOUT RESUMED BY /GOP
*GET _WALLASOL FROM ACTI ITEM=TIME WALL VALUE= 17.7158333
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:42:57 JAN 08, 2025 CP= 11.062
wbnew--Modal Campbell
***** MAPDL RESULTS INTERPRETATION (POST1) *****
*** NOTE *** CP = 11.062 TIME= 17:42:57
The model contains an element type ( COMBI214 ) that operates entirely
in the nodal coordinate system. Viewing nodal displacements or forces
in other than the nodal coordinate system may be invalid. See the
elements manual description for more information.
*** NOTE *** CP = 11.062 TIME= 17:42:57
Reading results into the database (SET command) will update the current
displacement and force boundary conditions in the database with the
values from the results file for that load set. Note that any
subsequent solutions will use these values unless action is taken to
either SAVE the current values or not overwrite them (/EXIT,NOSAVE).
PRINT CAMPBELL DIAGRAM
Sorting : ON
X axis unit : rd/s
Driving component : CM_ROTVELCITY137
Printout all frequencies
*** WARNING *** CP = 11.250 TIME= 17:42:57
Sorting process may not be successful due to the shape of some modes.
If results are not satisfactory, try to change the load steps and/or
the number of modes.
***** FREQUENCIES (Hz) FROM CAMPBELL (sorting on) *****
** The driving component is CM_ROTVELCITY137 **
Spin(rd/s) 0.000 5235.988 10471.976
1 BW 190.066 169.342 145.228
2 FW 209.160 232.820 264.965
3 BW 639.919 629.722 607.214
4 BW 660.193 657.098 654.009
5 FW 736.467 755.969 800.213
6 FW 812.138 814.386 820.996
7 991.342 991.342 991.342
8 BW 1784.269 1766.831 1749.515
9 FW 1784.806 1802.678 1821.310
PRINT CAMPBELL DIAGRAM
Sorting : ON
X axis unit : rd/s
Driving component : CM_ROTVELCITY137
Stability value : ON
Printout all frequencies
*** WARNING *** CP = 11.438 TIME= 17:42:57
Sorting process may not be successful due to the shape of some modes.
If results are not satisfactory, try to change the load steps and/or
the number of modes.
***** STABILITY VALUES (Hz) FROM CAMPBELL (sorting on) *****
** The driving component is CM_ROTVELCITY137 **
Spin(rd/s) 0.000 5235.988 10471.976
1 BW 0.000 0.000 0.000
2 FW 0.000 0.000 0.000
3 BW 0.000 0.000 0.000
4 BW 0.000 0.000 0.000
5 FW 0.000 0.000 0.000
6 FW 0.000 0.000 0.000
7 0.000 0.000 0.000
8 BW 0.000 0.000 0.000
9 FW 0.000 0.000 0.000
PRINTOUT RESUMED BY /GOP
PRINTOUT RESUMED BY /GOP
Set Encoding of XML File to:ISO-8859-1
Set Output of XML File to:
PARM, , , , , , , , , , , ,
, , , , , , ,
DATABASE WRITTEN ON FILE parm.xml
EXIT THE MAPDL POST1 DATABASE PROCESSOR
***** ROUTINE COMPLETED ***** CP = 11.594
PRINTOUT RESUMED BY /GOP
*GET _WALLDONE FROM ACTI ITEM=TIME WALL VALUE= 17.7158333
PARAMETER _PREPTIME = 0.000000000
PARAMETER _SOLVTIME = 12.00000000
PARAMETER _POSTTIME = 0.000000000
PARAMETER _TOTALTIM = 12.00000000
*GET _DLBRATIO FROM ACTI ITEM=SOLU DLBR VALUE= 1.00155521
*GET _COMBTIME FROM ACTI ITEM=SOLU COMB VALUE= 0.149815200
*GET _SSMODE FROM ACTI ITEM=SOLU SSMM VALUE= 2.00000000
*GET _NDOFS FROM ACTI ITEM=SOLU NDOF VALUE= 22781.0000
/FCLEAN COMMAND REMOVING ALL LOCAL FILES
--- Total number of nodes = 7698
--- Total number of elements = 2577
--- Element load balance ratio = 1.00155521
--- Time to combine distributed files = 0.1498152
--- Sparse memory mode = 2
--- Number of DOF = 22781
EXIT MAPDL WITHOUT SAVING DATABASE
NUMBER OF WARNING MESSAGES ENCOUNTERED= 8
NUMBER OF ERROR MESSAGES ENCOUNTERED= 0
+--------------------- M A P D L S T A T I S T I C S ------------------------+
Release: 2024 R2 Build: 24.2 Update: UP20240603 Platform: WINDOWS x64
Date Run: 01/08/2025 Time: 17:42 Process ID: 3796
Operating System: Windows 11 (Build: 22631)
Processor Model: Intel(R) Xeon(R) Platinum 8171M CPU @ 2.60GHz
Compiler: Intel(R) Fortran Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) C/C++ Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) oneAPI Math Kernel Library Version 2023.1-Product Build 20230303
Number of machines requested : 1
Total number of cores available : 8
Number of physical cores available : 4
Number of processes requested : 4
Number of threads per process requested : 1
Total number of cores requested : 4 (Distributed Memory Parallel)
MPI Type: INTELMPI
MPI Version: Intel(R) MPI Library 2021.11 for Windows* OS
GPU Acceleration: Not Requested
Job Name: file0
Input File: dummy.dat
Core Machine Name Working Directory
-----------------------------------------------------
0 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr81BD
1 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr81BD
2 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr81BD
3 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr81BD
Latency time from master to core 1 = 3.104 microseconds
Latency time from master to core 2 = 3.049 microseconds
Latency time from master to core 3 = 3.903 microseconds
Communication speed from master to core 1 = 4724.25 MB/sec
Communication speed from master to core 2 = 5233.38 MB/sec
Communication speed from master to core 3 = 4697.23 MB/sec
Total CPU time for main thread : 9.6 seconds
Total CPU time summed for all threads : 11.7 seconds
Elapsed time spent obtaining a license : 0.5 seconds
Elapsed time spent pre-processing model (/PREP7) : 0.1 seconds
Elapsed time spent solution - preprocessing : 0.2 seconds
Elapsed time spent computing solution : 11.5 seconds
Elapsed time spent solution - postprocessing : 0.1 seconds
Elapsed time spent post-processing model (/POST1) : 0.2 seconds
Eigensolver used : QRdamp
Equation solver computational rate : 16.0 Gflops
Sum of disk space used on all processes : 172.0 MB
Sum of memory used on all processes : 651.0 MB
Sum of memory allocated on all processes : 3246.0 MB
Physical memory available : 32 GB
Total amount of I/O written to disk : 0.4 GB
Total amount of I/O read from disk : 5.7 GB
+------------------ E N D M A P D L S T A T I S T I C S -------------------+
*-----------------------------------------------------------------------------*
| |
| RUN COMPLETED |
| |
|-----------------------------------------------------------------------------|
| |
| Ansys MAPDL 2024 R2 Build 24.2 UP20240603 WINDOWS x64 |
| |
|-----------------------------------------------------------------------------|
| |
| Database Requested(-db) 1024 MB Scratch Memory Requested 1024 MB |
| Max Database Used(Master) 5 MB Max Scratch Used(Master) 163 MB |
| Max Database Used(Workers) 1 MB Max Scratch Used(Workers) 161 MB |
| Sum Database Used(All) 8 MB Sum Scratch Used(All) 643 MB |
| |
|-----------------------------------------------------------------------------|
| |
| CP Time (sec) = 11.734 Time = 17:42:58 |
| Elapsed Time (sec) = 15.000 Date = 01/08/2025 |
| |
*-----------------------------------------------------------------------------*
Specify the Mechanical directory path for the Modal Campbell Analysis and fetch the image directory path. Download an image file (tot_deform_2D.png
) from the server to the client’s current working directory and display it using matplotlib
.
[16]:
from matplotlib import image as mpimg
from matplotlib import pyplot as plt
[17]:
mechanical.run_python_script(f"image_dir=ExtAPI.DataModel.AnalysisList[2].WorkingDir")
result_image_dir_server = mechanical.run_python_script(f"image_dir")
print(f"Images are stored on the server at: {result_image_dir_server}")
Images are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-6\MECH\
[18]:
def get_image_path(image_name):
return os.path.join(result_image_dir_server, image_name)
[19]:
def display_image(path):
print(f"Printing {path} using matplotlib")
image1 = mpimg.imread(path)
plt.figure(figsize=(15, 15))
plt.axis("off")
plt.imshow(image1)
plt.show()
[20]:
image_name = "tot_deform_2D.png"
image_path_server = get_image_path(image_name)
[21]:
if image_path_server != "":
current_working_directory = os.getcwd()
local_file_path_list = mechanical.download(
image_path_server, target_dir=current_working_directory
)
image_local_path = local_file_path_list[0]
print(f"Local image path : {image_local_path}")
display_image(image_local_path)
Downloading dns:///127.0.0.1:54649:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-6\MECH\tot_deform_2D.png to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\tot_deform_2D.png: 100%|██████████| 72.7k/72.7k [00:00<?, ?B/s]
Local image path : C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\tot_deform_2D.png
Printing C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\tot_deform_2D.png using matplotlib
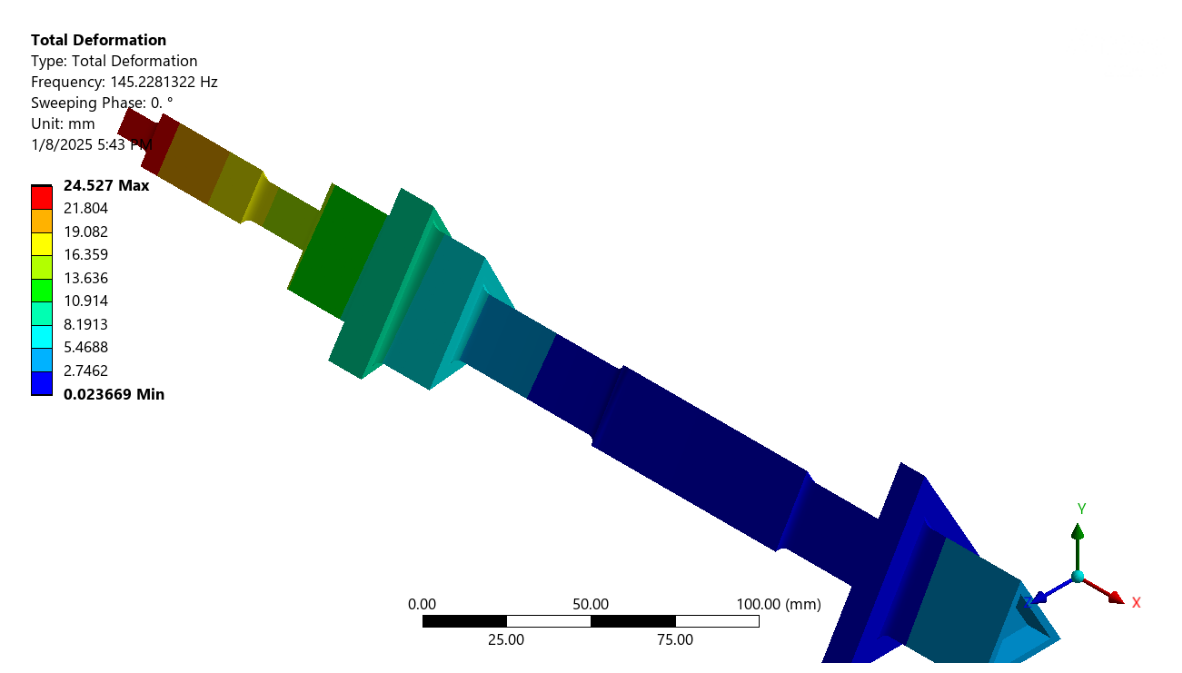
Specify the Mechanical directory for the Unbalance Response Analysis and fetch the working directory path. Download the solver output file (solve.out
) from the server to the client’s current working directory and print its contents.
[22]:
mechanical.run_python_script(f"solve_dir=ExtAPI.DataModel.AnalysisList[3].WorkingDir")
result_solve_dir_server = mechanical.run_python_script(f"solve_dir")
print(f"All solver files are stored on the server at: {result_solve_dir_server}")
All solver files are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-7\MECH\
[23]:
solve_out_path = os.path.join(result_solve_dir_server, "solve.out")
[24]:
def write_file_contents_to_console(path):
"""Write file contents to console."""
with open(path, "rt") as file:
for line in file:
print(line, end="")
[25]:
current_working_directory = os.getcwd()
mechanical.download(solve_out_path, target_dir=current_working_directory)
solve_out_local_path = os.path.join(current_working_directory, "solve.out")
write_file_contents_to_console(solve_out_local_path)
os.remove(solve_out_local_path)
Downloading dns:///127.0.0.1:54649:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-7\MECH\solve.out to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\solve.out: 100%|██████████| 70.7k/70.7k [00:00<?, ?B/s]
Ansys Mechanical Enterprise
*------------------------------------------------------------------*
| |
| W E L C O M E T O T H E A N S Y S (R) P R O G R A M |
| |
*------------------------------------------------------------------*
***************************************************************
* ANSYS MAPDL 2024 R2 LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2024 Ansys, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of Ansys, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the Ansys, Inc. online documentation or the Ansys, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* Ansys, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* Ansys, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the Ansys, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the Ansys, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
2024 R2
Point Releases and Patches installed:
Ansys Service Pack 2024 R2.01
Ansys Service Pack 2024 R2.02
Ansys Service Pack 2024 R2.03
Ansys, Inc. License Manager 2024 R2
Ansys, Inc. License Manager 2024 R2.01
Ansys, Inc. License Manager 2024 R2.02
Ansys, Inc. License Manager 2024 R2.03
Discovery 2024 R2
Discovery 2024 R2.01
Discovery 2024 R2.02
Discovery 2024 R2.03
Core WB Files 2024 R2
Core WB Files 2024 R2.01
Core WB Files 2024 R2.02
Core WB Files 2024 R2.03
SpaceClaim 2024 R2
SpaceClaim 2024 R2.01
SpaceClaim 2024 R2.02
SpaceClaim 2024 R2.03
Icepak (includes CFD-Post) 2024 R2
Icepak (includes CFD-Post) 2024 R2.01
Icepak (includes CFD-Post) 2024 R2.02
Icepak (includes CFD-Post) 2024 R2.03
CFD-Post only 2024 R2
CFD-Post only 2024 R2.01
CFD-Post only 2024 R2.02
CFD-Post only 2024 R2.03
CFX (includes CFD-Post) 2024 R2
CFX (includes CFD-Post) 2024 R2.01
CFX (includes CFD-Post) 2024 R2.02
CFX (includes CFD-Post) 2024 R2.03
Chemkin 2024 R2
Chemkin 2024 R2.01
Chemkin 2024 R2.02
Chemkin 2024 R2.03
EnSight 2024 R2
EnSight 2024 R2.01
EnSight 2024 R2.02
EnSight 2024 R2.03
FENSAP-ICE 2024 R2
FENSAP-ICE 2024 R2.01
FENSAP-ICE 2024 R2.02
FENSAP-ICE 2024 R2.03
Fluent (includes CFD-Post) 2024 R2
Fluent (includes CFD-Post) 2024 R2.01
Fluent (includes CFD-Post) 2024 R2.02
Fluent (includes CFD-Post) 2024 R2.03
Polyflow (includes CFD-Post) 2024 R2
Polyflow (includes CFD-Post) 2024 R2.01
Polyflow (includes CFD-Post) 2024 R2.02
Polyflow (includes CFD-Post) 2024 R2.03
Forte (includes EnSight) 2024 R2
Forte (includes EnSight) 2024 R2.01
Forte (includes EnSight) 2024 R2.02
Forte (includes EnSight) 2024 R2.03
ICEM CFD 2024 R2
ICEM CFD 2024 R2.01
ICEM CFD 2024 R2.02
ICEM CFD 2024 R2.03
TurboGrid 2024 R2
TurboGrid 2024 R2.01
TurboGrid 2024 R2.02
TurboGrid 2024 R2.03
Speos 2024 R2
Speos 2024 R2.01
Speos 2024 R2.02
Speos 2024 R2.03
Speos HPC 2024 R2
Speos HPC 2024 R2.01
Speos HPC 2024 R2.02
Speos HPC 2024 R2.03
optiSLang 2024 R2
optiSLang 2024 R2.01
optiSLang 2024 R2.02
optiSLang 2024 R2.03
Remote Solve Manager Standalone Services 2024 R2
Remote Solve Manager Standalone Services 2024 R2.01
Remote Solve Manager Standalone Services 2024 R2.02
Remote Solve Manager Standalone Services 2024 R2.03
Additive 2024 R2
Additive 2024 R2.01
Additive 2024 R2.02
Additive 2024 R2.03
Aqwa 2024 R2
Aqwa 2024 R2.01
Aqwa 2024 R2.02
Aqwa 2024 R2.03
Autodyn 2024 R2
Autodyn 2024 R2.01
Autodyn 2024 R2.02
Autodyn 2024 R2.03
Customization Files for User Programmable Features 2024 R2
Customization Files for User Programmable Features 2024 R2.01
Customization Files for User Programmable Features 2024 R2.02
Customization Files for User Programmable Features 2024 R2.03
LS-DYNA 2024 R2
LS-DYNA 2024 R2.01
LS-DYNA 2024 R2.02
LS-DYNA 2024 R2.03
Mechanical Products 2024 R2
Mechanical Products 2024 R2.01
Mechanical Products 2024 R2.02
Mechanical Products 2024 R2.03
Motion 2024 R2
Motion 2024 R2.01
Motion 2024 R2.02
Motion 2024 R2.03
Sherlock 2024 R2
Sherlock 2024 R2.01
Sherlock 2024 R2.02
Sherlock 2024 R2.03
Sound - SAS 2024 R2
Sound - SAS 2024 R2.01
Sound - SAS 2024 R2.02
Sound - SAS 2024 R2.03
ACIS Geometry Interface 2024 R2
ACIS Geometry Interface 2024 R2.01
ACIS Geometry Interface 2024 R2.02
ACIS Geometry Interface 2024 R2.03
AutoCAD Geometry Interface 2024 R2
AutoCAD Geometry Interface 2024 R2.01
AutoCAD Geometry Interface 2024 R2.02
AutoCAD Geometry Interface 2024 R2.03
Catia, Version 4 Geometry Interface 2024 R2
Catia, Version 4 Geometry Interface 2024 R2.01
Catia, Version 4 Geometry Interface 2024 R2.02
Catia, Version 4 Geometry Interface 2024 R2.03
Catia, Version 5 Geometry Interface 2024 R2
Catia, Version 5 Geometry Interface 2024 R2.01
Catia, Version 5 Geometry Interface 2024 R2.02
Catia, Version 5 Geometry Interface 2024 R2.03
Catia, Version 6 Geometry Interface 2024 R2
Catia, Version 6 Geometry Interface 2024 R2.01
Catia, Version 6 Geometry Interface 2024 R2.02
Catia, Version 6 Geometry Interface 2024 R2.03
Creo Elements/Direct Modeling Geometry Interface 2024 R2
Creo Elements/Direct Modeling Geometry Interface 2024 R2.01
Creo Elements/Direct Modeling Geometry Interface 2024 R2.02
Creo Elements/Direct Modeling Geometry Interface 2024 R2.03
Creo Parametric Geometry Interface 2024 R2
Creo Parametric Geometry Interface 2024 R2.01
Creo Parametric Geometry Interface 2024 R2.02
Creo Parametric Geometry Interface 2024 R2.03
Inventor Geometry Interface 2024 R2
Inventor Geometry Interface 2024 R2.01
Inventor Geometry Interface 2024 R2.02
Inventor Geometry Interface 2024 R2.03
JTOpen Geometry Interface 2024 R2
JTOpen Geometry Interface 2024 R2.01
JTOpen Geometry Interface 2024 R2.02
JTOpen Geometry Interface 2024 R2.03
NX Geometry Interface 2024 R2
NX Geometry Interface 2024 R2.01
NX Geometry Interface 2024 R2.02
NX Geometry Interface 2024 R2.03
Parasolid Geometry Interface 2024 R2
Parasolid Geometry Interface 2024 R2.01
Parasolid Geometry Interface 2024 R2.02
Parasolid Geometry Interface 2024 R2.03
Solid Edge Geometry Interface 2024 R2
Solid Edge Geometry Interface 2024 R2.01
Solid Edge Geometry Interface 2024 R2.02
Solid Edge Geometry Interface 2024 R2.03
SOLIDWORKS Geometry Interface 2024 R2
SOLIDWORKS Geometry Interface 2024 R2.01
SOLIDWORKS Geometry Interface 2024 R2.02
SOLIDWORKS Geometry Interface 2024 R2.03
***** MAPDL COMMAND LINE ARGUMENTS *****
BATCH MODE REQUESTED (-b) = NOLIST
INPUT FILE COPY MODE (-c) = COPY
DISTRIBUTED MEMORY PARALLEL REQUESTED
4 PARALLEL PROCESSES REQUESTED WITH SINGLE THREAD PER PROCESS
TOTAL OF 4 CORES REQUESTED
INPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB8DE\dummy.dat
OUTPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB8DE\solve.out
START-UP FILE MODE = NOREAD
STOP FILE MODE = NOREAD
RELEASE= 2024 R2 BUILD= 24.2 UP20240603 VERSION=WINDOWS x64
CURRENT JOBNAME=file0 17:43:11 JAN 08, 2025 CP= 0.078
PARAMETER _DS_PROGRESS = 999.0000000
/INPUT FILE= ds.dat LINE= 0
*** NOTE *** CP = 0.297 TIME= 17:43:11
The /CONFIG,NOELDB command is not valid in a distributed memory
parallel solution. Command is ignored.
*GET _WALLSTRT FROM ACTI ITEM=TIME WALL VALUE= 17.7197222
TITLE=
wbnew--Unbalance Response
ACT Extensions:
LSDYNA, 2024.2
5f463412-bd3e-484b-87e7-cbc0a665e474, wbex
/COM, ANSYSMotion, 2024.2
20180725-3f81-49eb-9f31-41364844c769, wbex
SET PARAMETER DIMENSIONS ON _WB_PROJECTSCRATCH_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_PROJECTSCRATCH_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB8DE\
SET PARAMETER DIMENSIONS ON _WB_SOLVERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_SOLVERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-7\MECH\
SET PARAMETER DIMENSIONS ON _WB_USERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_USERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\user_files\
--- Data in consistent NMM units. See Solving Units in the help system for more
MPA UNITS SPECIFIED FOR INTERNAL
LENGTH = MILLIMETERS (mm)
MASS = TONNE (Mg)
TIME = SECONDS (sec)
TEMPERATURE = CELSIUS (C)
TOFFSET = 273.0
FORCE = NEWTON (N)
HEAT = MILLIJOULES (mJ)
INPUT UNITS ARE ALSO SET TO MPA
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:43:11 JAN 08, 2025 CP= 0.328
wbnew--Unbalance Response
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
*********** Nodes for the whole assembly ***********
*********** Nodes for all Remote Points ***********
*********** Elements for Body 1 'Surface Body1' ***********
*********** Elements for Body 2 'Surface Body2' ***********
*********** Elements for Body 3 'Surface Body3' ***********
*********** Elements for Body 4 'Surface Body4' ***********
*********** Send User Defined Coordinate System(s) ***********
*********** Set Reference Temperature ***********
*********** Send Materials ***********
*********** Send Sheet Properties ***********
*********** Send General Axisymmetric Properties "General Axisymmetric" *******
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Element Component ***********
*********** Send Named Selection as Node Component ***********
*********** Create Remote Point "PointMass_RemotePoint" ***********
*********** Create Remote Point "RemotePoint_Bearing1" ***********
*********** Create Remote Point "RemotePoint_Bearing2" ***********
*********** Create Remote Point "RemotePoint_FreeStanding1" ***********
*********** Create Remote Point "RemotePoint_FreeStanding2" ***********
*********** Create Remote Point "PointMass_RemotePoint2" ***********
*********** Construct Remote Mass Using Remote Attachment ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*** Create a component for all remote displacements ***
*********** Create Bearing Connection "RemotePoint_FreeStanding1 To Multiple" *
Real Constant Set For Above Bearing Connection Is 14
*********** Create Bearing Connection "RemotePoint_FreeStanding2 To Multiple" *
Real Constant Set For Above Bearing Connection Is 15
*********** Component For Rotating Forces ***********
Component For : All Bodies
*********** SYNCHRO & CMOMEGA For Rotating Forces ***********
SYNCHRONOUS NODAL FORCE EXCITATION
*********** Define Rotating Force "Rotating Force" ***********
RotatingForceComponentName,cm_SynchroComponent
RotatingForceLoc,89.0100021362305,0.5,0.
UnbalancedMass,3.8e-003
UnbalancedForce,1.9e-003
RotatingRadius,0.5
PhaseAngle,0.
RotatingForceAxisLoc,89.01,0.,0.
RotatingForceAxisComponents,1.,0.,0.
HitPointLocation,89.0100021362305,0.,0.
HitPointNodeId,7689
***** ROUTINE COMPLETED ***** CP = 0.438
--- Number of total nodes = 7698
--- Number of contact elements = 142
--- Number of spring elements = 0
--- Number of bearing elements = 0
--- Number of solid elements = 2428
--- Number of condensed parts = 0
--- Number of total elements = 2577
*GET _WALLBSOL FROM ACTI ITEM=TIME WALL VALUE= 17.7197222
***** MAPDL SOLUTION ROUTINE *****
PERFORM A HARMONIC ANALYSIS
THIS WILL BE A NEW ANALYSIS
PERFORM A FULL HARMONIC RESPONSE ANALYSIS
THERMAL STRAINS ARE NOT INCLUDED IN THE LOAD VECTOR.
STEP BOUNDARY CONDITION KEY= 1
HARMONIC FREQUENCY RANGE - END= 1666.7 BEGIN= 0.0000
USE 200 SUBSTEP(S) THIS LOAD STEP FOR ALL DEGREES OF FREEDOM
STRUCTURAL DAMPING COEFFICIENT = 0.20000E-01
CORIOLIS IN STATIONARY REFERENCE FRAME: GYROSCOPIC DAMPING MATRIX WILL BE CALCULATED
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EANG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE ETMP ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE VENG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE STRS ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPEL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE RSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE CONT ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
PRINTOUT RESUMED BY /GOP
WRITE MISC ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR THE ENTITIES DEFINED BY COMPONENT _ELMISC
*GET ANSINTER_ FROM ACTI ITEM=INT VALUE= 0.00000000
*IF ANSINTER_ ( = 0.00000 ) NE
0 ( = 0.00000 ) THEN
*ENDIF
*** NOTE *** CP = 0.469 TIME= 17:43:11
The automatic domain decomposition logic has selected the FREQ domain
decomposition method with 1 processes per frequency solution.
***** MAPDL SOLVE COMMAND *****
D I S T R I B U T E D D O M A I N D E C O M P O S E R
...Number of frequency solutions: 200
...Decompose to 4 frequency domains (with 1 processes per domain)
*** WARNING *** CP = 0.750 TIME= 17:43:12
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
HARMONIC EXCITATION IS UNBALANCE
Rotational velocity equals excitation frequency
Rotating component considered = CM_SYNCHROCOMPONENT
*** NOTE *** CP = 0.781 TIME= 17:43:12
The model data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB
B8DE\file0.err ) for these warning messages.
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID272. KEYOPT(6) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID272. KEYOPT(6) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 3 IS SOLID272. KEYOPT(6) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
ELEMENT TYPE 4 IS SOLID272. KEYOPT(6) IS ALREADY SET AS SUGGESTED AND NO
RESETTING IS NEEDED.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:43:12 JAN 08, 2025 CP= 0.781
wbnew--Unbalance Response
S O L U T I O N O P T I O N S
PROBLEM DIMENSIONALITY. . . . . . . . . . . . .3-D
DEGREES OF FREEDOM. . . . . . UX UY UZ ROTX ROTY ROTZ
ANALYSIS TYPE . . . . . . . . . . . . . . . . .HARMONIC
SOLUTION METHOD. . . . . . . . . . . . . . .FULL
OFFSET TEMPERATURE FROM ABSOLUTE ZERO . . . . . 273.15
THERMAL EXPANSION . . . . . . . . . . . . . . .OFF
COMPLEX DISPLACEMENT PRINT OPTION . . . . . . .REAL AND IMAGINARY
GLOBALLY ASSEMBLED MATRIX . . . . . . . . . . .UNSYMMETRIC
*** WARNING *** CP = 0.797 TIME= 17:43:12
Material number 14 (used by element 2435) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 0.797 TIME= 17:43:12
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB
B8DE\file0.err ) for these warning messages.
*** NOTE *** CP = 0.812 TIME= 17:43:12
Internal nodes from 7699 to 7702 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
*** NOTE *** CP = 0.828 TIME= 17:43:12
Internal nodes from 7699 to 7702 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
*WARNING*: Node 324 has been used on different contact pairs (real ID 5
& 11). These two pairs will be merged. Please check the model
carefully.
*** NOTE *** CP = 0.859 TIME= 17:43:12
Rigid-constraint surface identified by real constant set 5 and contact
element type 5 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7689 which connects to other
element 2583. Internal MPC will be built.
This pair will be merged with other pair defined by real constant set
11.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** WARNING *** CP = 0.859 TIME= 17:43:12
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** WARNING *** CP = 0.859 TIME= 17:43:12
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** NOTE *** CP = 0.859 TIME= 17:43:12
Rigid-constraint surface identified by real constant set 7 and contact
element type 7 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7690 which connects to other
element 2435. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** WARNING *** CP = 0.859 TIME= 17:43:12
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** NOTE *** CP = 0.859 TIME= 17:43:12
Rigid-constraint surface identified by real constant set 9 and contact
element type 9 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7691 which connects to other
element 2436. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** WARNING *** CP = 0.859 TIME= 17:43:12
Material number 14 (used by element 2435) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 0.859 TIME= 17:43:12
Rigid-constraint surface identified by real constant set 11 and
contact element type 11 has been set up. The degrees of freedom of
rigid surface are driven by the pilot node 7694. Internal MPC will be
built.
This pair will be merged with other pair defined by real constant set
5.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
Please verify constraints (including rotational degrees of freedom)
on the pilot node by yourself.
****************************************
*** WARNING *** CP = 0.859 TIME= 17:43:12
Material number 14 (used by element 2435) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 0.875 TIME= 17:43:12
Internal nodes from 7699 to 7702 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 1
FREQUENCY RANGE . . . . . . . . . . . . . . . . 0.0000 TO 1666.7
NUMBER OF SUBSTEPS. . . . . . . . . . . . . . . 200
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . YES
STRUCTURAL DAMPING COEFFICIENT. . . . . . . . . 0.20000E-01
CORIOLIS EFFECT IN STATIONARY REF. FRAME . . . ON
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
RSOL ALL
CONT ALL
MISC ALL _ELMISC
*WARNING*: Node 324 has been used on different contact pairs (real ID 5
& 11). These two pairs will be merged. Please check the model
carefully.
*** WARNING *** CP = 1.000 TIME= 17:43:12
Material number 14 (used by element 2435) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 1.000 TIME= 17:43:12
Rigid-constraint surface identified by real constant set 5 and contact
element type 5 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7689 which connects to other
element 2583. Internal MPC will be built.
This pair will be merged with other pair defined by real constant set
11.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** NOTE *** CP = 1.000 TIME= 17:43:12
Rigid-constraint surface identified by real constant set 7 and contact
element type 7 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7690 which connects to other
element 2435. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** NOTE *** CP = 1.000 TIME= 17:43:12
Rigid-constraint surface identified by real constant set 9 and contact
element type 9 has been set up. The degrees of freedom of the rigid
surface are driven by the pilot node 7691 which connects to other
element 2436. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** NOTE *** CP = 1.000 TIME= 17:43:12
Rigid-constraint surface identified by real constant set 11 and
contact element type 11 has been set up. The degrees of freedom of
rigid surface are driven by the pilot node 7694. Internal MPC will be
built.
This pair will be merged with other pair defined by real constant set
5.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
Please verify constraints (including rotational degrees of freedom)
on the pilot node by yourself.
****************************************
*********** PRECISE MASS SUMMARY ***********
TOTAL RIGID BODY MASS MATRIX ABOUT ORIGIN
Translational mass | Coupled translational/rotational mass
0.38553E-02 0.0000 0.0000 | 0.0000 -0.10217E-17 0.11484E-12
0.0000 0.38553E-02 0.13172E-20 | 0.19954E-17 -0.13482E-18 0.62268
0.0000 0.26458E-20 0.38553E-02 | -0.11484E-12 -0.62268 0.62267E-18
------------------------------------------ | ------------------------------------------
| Rotational mass (inertia)
| 2.7510 0.52043E-10 0.43541E-15
| 0.52043E-10 149.62 -0.11702E-15
| 0.42266E-15 0.47130E-16 149.62
TOTAL MASS = 0.38553E-02
The mass principal axes coincide with the global Cartesian axes
CENTER OF MASS (X,Y,Z)= 161.51 -0.29787E-10 -0.26502E-15
TOTAL INERTIA ABOUT CENTER OF MASS
2.7510 0.33495E-10 0.11312E-15
0.33495E-10 49.050 -0.11702E-15
0.11312E-15 -0.11702E-15 49.050
The inertia principal axes coincide with the global Cartesian axes
*** MASS SUMMARY BY ELEMENT TYPE ***
TYPE MASS
1 0.274552E-03
2 0.769219E-03
3 0.621633E-03
4 0.788881E-03
13 0.140100E-02
Range of element maximum matrix coefficients in global coordinates
Maximum = 14269624.8 at element 1019.
Minimum = 35030 at element 2436.
*** ELEMENT MATRIX FORMULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 441 SOLID272 0.203 0.000461
2 580 SOLID272 0.188 0.000323
3 810 SOLID272 0.281 0.000347
4 597 SOLID272 0.172 0.000288
5 43 CONTA175 0.000 0.000000
6 1 TARGE170 0.000 0.000000
7 28 CONTA175 0.000 0.000000
8 1 TARGE170 0.000 0.000000
9 28 CONTA175 0.000 0.000000
10 1 TARGE170 0.000 0.000000
11 43 CONTA175 0.000 0.000000
12 1 TARGE170 0.000 0.000000
13 1 MASS21 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 COMBI214 0.000 0.000000
Time at end of element matrix formulation CP = 1.96875.
*** GYROSCOPIC DAMPING MATRIX CALCULATED FOR LISTED ELEMENTS:
TYPE NUMBER ENAME
1 441 SOLID272
2 580 SOLID272
3 810 SOLID272
4 597 SOLID272
13 1 MASS21
SPARSE MATRIX DIRECT SOLVER.
Number of equations = 22781, Maximum wavefront = 558
Memory allocated on this process
-------------------------------------------------------------------
Equation solver memory allocated = 209.307 MB
Equation solver memory required for in-core mode = 201.306 MB
Equation solver memory required for out-of-core mode = 104.723 MB
Total (solver and non-solver) memory allocated = 860.588 MB
*** NOTE *** CP = 2.391 TIME= 17:43:13
The Sparse Matrix Solver is currently running in the in-core memory
mode. This memory mode uses the most amount of memory in order to
avoid using the hard drive as much as possible, which most often
results in the fastest solution time. This mode is recommended if
enough physical memory is present to accommodate all of the solver
data.
*** NOTE *** CP = 2.391 TIME= 17:43:13
The system matrix is unsymmetric.
Sparse solver maximum pivot= 3.005101191E+09 at node 7689 ROTY.
Sparse solver minimum pivot= 20922.4616 at node 4388 UY.
Sparse solver minimum pivot in absolute value= 20922.4616 at node 4388
UY.
*** ELEMENT RESULT CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 441 SOLID272 0.156 0.000354
2 580 SOLID272 0.156 0.000269
3 810 SOLID272 0.266 0.000328
4 597 SOLID272 0.141 0.000236
5 43 CONTA175 0.000 0.000000
7 28 CONTA175 0.000 0.000000
9 28 CONTA175 0.000 0.000000
11 43 CONTA175 0.000 0.000000
13 1 MASS21 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 COMBI214 0.000 0.000000
*** NODAL LOAD CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 441 SOLID272 0.000 0.000000
2 580 SOLID272 0.047 0.000081
3 810 SOLID272 0.016 0.000019
4 597 SOLID272 0.031 0.000052
5 43 CONTA175 0.000 0.000000
7 28 CONTA175 0.000 0.000000
9 28 CONTA175 0.000 0.000000
11 43 CONTA175 0.000 0.000000
13 1 MASS21 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 COMBI214 0.000 0.000000
*** LOAD STEP 1 SUBSTEP 1 COMPLETED. FREQUENCY= 8.33350
--- CMOMEGA for CM_SYNCH= 52.361 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 2 COMPLETED. FREQUENCY= 16.6670
--- CMOMEGA for CM_SYNCH= 104.72 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 3 COMPLETED. FREQUENCY= 25.0005
--- CMOMEGA for CM_SYNCH= 157.08 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 4 COMPLETED. FREQUENCY= 33.3340
--- CMOMEGA for CM_SYNCH= 209.44 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 5 COMPLETED. FREQUENCY= 41.6675
--- CMOMEGA for CM_SYNCH= 261.80 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 6 COMPLETED. FREQUENCY= 50.0010
--- CMOMEGA for CM_SYNCH= 314.17 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 7 COMPLETED. FREQUENCY= 58.3345
--- CMOMEGA for CM_SYNCH= 366.53 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 8 COMPLETED. FREQUENCY= 66.6680
--- CMOMEGA for CM_SYNCH= 418.89 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 9 COMPLETED. FREQUENCY= 75.0015
--- CMOMEGA for CM_SYNCH= 471.25 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 10 COMPLETED. FREQUENCY= 83.3350
--- CMOMEGA for CM_SYNCH= 523.61 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 11 COMPLETED. FREQUENCY= 91.6685
--- CMOMEGA for CM_SYNCH= 575.97 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 12 COMPLETED. FREQUENCY= 100.002
--- CMOMEGA for CM_SYNCH= 628.33 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 13 COMPLETED. FREQUENCY= 108.336
--- CMOMEGA for CM_SYNCH= 680.69 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 14 COMPLETED. FREQUENCY= 116.669
--- CMOMEGA for CM_SYNCH= 733.05 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 15 COMPLETED. FREQUENCY= 125.002
--- CMOMEGA for CM_SYNCH= 785.41 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 16 COMPLETED. FREQUENCY= 133.336
--- CMOMEGA for CM_SYNCH= 837.77 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 17 COMPLETED. FREQUENCY= 141.670
--- CMOMEGA for CM_SYNCH= 890.14 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 18 COMPLETED. FREQUENCY= 150.003
--- CMOMEGA for CM_SYNCH= 942.50 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 19 COMPLETED. FREQUENCY= 158.337
--- CMOMEGA for CM_SYNCH= 994.86 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 20 COMPLETED. FREQUENCY= 166.670
--- CMOMEGA for CM_SYNCH= 1047.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 21 COMPLETED. FREQUENCY= 175.004
--- CMOMEGA for CM_SYNCH= 1099.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 22 COMPLETED. FREQUENCY= 183.337
--- CMOMEGA for CM_SYNCH= 1151.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 23 COMPLETED. FREQUENCY= 191.671
--- CMOMEGA for CM_SYNCH= 1204.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 24 COMPLETED. FREQUENCY= 200.004
--- CMOMEGA for CM_SYNCH= 1256.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 25 COMPLETED. FREQUENCY= 208.338
--- CMOMEGA for CM_SYNCH= 1309.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 26 COMPLETED. FREQUENCY= 216.671
--- CMOMEGA for CM_SYNCH= 1361.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 27 COMPLETED. FREQUENCY= 225.005
--- CMOMEGA for CM_SYNCH= 1413.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 28 COMPLETED. FREQUENCY= 233.338
--- CMOMEGA for CM_SYNCH= 1466.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 29 COMPLETED. FREQUENCY= 241.671
--- CMOMEGA for CM_SYNCH= 1518.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 30 COMPLETED. FREQUENCY= 250.005
--- CMOMEGA for CM_SYNCH= 1570.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 31 COMPLETED. FREQUENCY= 258.339
--- CMOMEGA for CM_SYNCH= 1623.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 32 COMPLETED. FREQUENCY= 266.672
--- CMOMEGA for CM_SYNCH= 1675.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 33 COMPLETED. FREQUENCY= 275.006
--- CMOMEGA for CM_SYNCH= 1727.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 34 COMPLETED. FREQUENCY= 283.339
--- CMOMEGA for CM_SYNCH= 1780.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 35 COMPLETED. FREQUENCY= 291.673
--- CMOMEGA for CM_SYNCH= 1832.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 36 COMPLETED. FREQUENCY= 300.006
--- CMOMEGA for CM_SYNCH= 1885.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 37 COMPLETED. FREQUENCY= 308.339
--- CMOMEGA for CM_SYNCH= 1937.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 38 COMPLETED. FREQUENCY= 316.673
--- CMOMEGA for CM_SYNCH= 1989.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 39 COMPLETED. FREQUENCY= 325.007
--- CMOMEGA for CM_SYNCH= 2042.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 40 COMPLETED. FREQUENCY= 333.340
--- CMOMEGA for CM_SYNCH= 2094.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 41 COMPLETED. FREQUENCY= 341.674
--- CMOMEGA for CM_SYNCH= 2146.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 42 COMPLETED. FREQUENCY= 350.007
--- CMOMEGA for CM_SYNCH= 2199.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 43 COMPLETED. FREQUENCY= 358.341
--- CMOMEGA for CM_SYNCH= 2251.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 44 COMPLETED. FREQUENCY= 366.674
--- CMOMEGA for CM_SYNCH= 2303.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 45 COMPLETED. FREQUENCY= 375.007
--- CMOMEGA for CM_SYNCH= 2356.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 46 COMPLETED. FREQUENCY= 383.341
--- CMOMEGA for CM_SYNCH= 2408.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 47 COMPLETED. FREQUENCY= 391.675
--- CMOMEGA for CM_SYNCH= 2461.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 48 COMPLETED. FREQUENCY= 400.008
--- CMOMEGA for CM_SYNCH= 2513.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 49 COMPLETED. FREQUENCY= 408.341
--- CMOMEGA for CM_SYNCH= 2565.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 50 COMPLETED. FREQUENCY= 416.675
--- CMOMEGA for CM_SYNCH= 2618.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 51 COMPLETED. FREQUENCY= 425.009
--- CMOMEGA for CM_SYNCH= 2670.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 52 COMPLETED. FREQUENCY= 433.342
--- CMOMEGA for CM_SYNCH= 2722.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 53 COMPLETED. FREQUENCY= 441.676
--- CMOMEGA for CM_SYNCH= 2775.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 54 COMPLETED. FREQUENCY= 450.009
--- CMOMEGA for CM_SYNCH= 2827.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 55 COMPLETED. FREQUENCY= 458.343
--- CMOMEGA for CM_SYNCH= 2879.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 56 COMPLETED. FREQUENCY= 466.676
--- CMOMEGA for CM_SYNCH= 2932.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 57 COMPLETED. FREQUENCY= 475.010
--- CMOMEGA for CM_SYNCH= 2984.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 58 COMPLETED. FREQUENCY= 483.343
--- CMOMEGA for CM_SYNCH= 3036.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 59 COMPLETED. FREQUENCY= 491.676
--- CMOMEGA for CM_SYNCH= 3089.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 60 COMPLETED. FREQUENCY= 500.010
--- CMOMEGA for CM_SYNCH= 3141.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 61 COMPLETED. FREQUENCY= 508.344
--- CMOMEGA for CM_SYNCH= 3194.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 62 COMPLETED. FREQUENCY= 516.677
--- CMOMEGA for CM_SYNCH= 3246.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 63 COMPLETED. FREQUENCY= 525.010
--- CMOMEGA for CM_SYNCH= 3298.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 64 COMPLETED. FREQUENCY= 533.344
--- CMOMEGA for CM_SYNCH= 3351.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 65 COMPLETED. FREQUENCY= 541.678
--- CMOMEGA for CM_SYNCH= 3403.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 66 COMPLETED. FREQUENCY= 550.011
--- CMOMEGA for CM_SYNCH= 3455.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 67 COMPLETED. FREQUENCY= 558.345
--- CMOMEGA for CM_SYNCH= 3508.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 68 COMPLETED. FREQUENCY= 566.678
--- CMOMEGA for CM_SYNCH= 3560.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 69 COMPLETED. FREQUENCY= 575.012
--- CMOMEGA for CM_SYNCH= 3612.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 70 COMPLETED. FREQUENCY= 583.345
--- CMOMEGA for CM_SYNCH= 3665.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 71 COMPLETED. FREQUENCY= 591.678
--- CMOMEGA for CM_SYNCH= 3717.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 72 COMPLETED. FREQUENCY= 600.012
--- CMOMEGA for CM_SYNCH= 3770.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 73 COMPLETED. FREQUENCY= 608.346
--- CMOMEGA for CM_SYNCH= 3822.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 74 COMPLETED. FREQUENCY= 616.679
--- CMOMEGA for CM_SYNCH= 3874.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 75 COMPLETED. FREQUENCY= 625.013
--- CMOMEGA for CM_SYNCH= 3927.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 76 COMPLETED. FREQUENCY= 633.346
--- CMOMEGA for CM_SYNCH= 3979.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 77 COMPLETED. FREQUENCY= 641.680
--- CMOMEGA for CM_SYNCH= 4031.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 78 COMPLETED. FREQUENCY= 650.013
--- CMOMEGA for CM_SYNCH= 4084.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 79 COMPLETED. FREQUENCY= 658.346
--- CMOMEGA for CM_SYNCH= 4136.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 80 COMPLETED. FREQUENCY= 666.680
--- CMOMEGA for CM_SYNCH= 4188.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 81 COMPLETED. FREQUENCY= 675.014
--- CMOMEGA for CM_SYNCH= 4241.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 82 COMPLETED. FREQUENCY= 683.347
--- CMOMEGA for CM_SYNCH= 4293.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 83 COMPLETED. FREQUENCY= 691.681
--- CMOMEGA for CM_SYNCH= 4346.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 84 COMPLETED. FREQUENCY= 700.014
--- CMOMEGA for CM_SYNCH= 4398.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 85 COMPLETED. FREQUENCY= 708.347
--- CMOMEGA for CM_SYNCH= 4450.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 86 COMPLETED. FREQUENCY= 716.681
--- CMOMEGA for CM_SYNCH= 4503.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 87 COMPLETED. FREQUENCY= 725.014
--- CMOMEGA for CM_SYNCH= 4555.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 88 COMPLETED. FREQUENCY= 733.348
--- CMOMEGA for CM_SYNCH= 4607.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 89 COMPLETED. FREQUENCY= 741.682
--- CMOMEGA for CM_SYNCH= 4660.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 90 COMPLETED. FREQUENCY= 750.015
--- CMOMEGA for CM_SYNCH= 4712.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 91 COMPLETED. FREQUENCY= 758.349
--- CMOMEGA for CM_SYNCH= 4764.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 92 COMPLETED. FREQUENCY= 766.682
--- CMOMEGA for CM_SYNCH= 4817.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 93 COMPLETED. FREQUENCY= 775.016
--- CMOMEGA for CM_SYNCH= 4869.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 94 COMPLETED. FREQUENCY= 783.349
--- CMOMEGA for CM_SYNCH= 4921.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 95 COMPLETED. FREQUENCY= 791.683
--- CMOMEGA for CM_SYNCH= 4974.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 96 COMPLETED. FREQUENCY= 800.016
--- CMOMEGA for CM_SYNCH= 5026.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 97 COMPLETED. FREQUENCY= 808.350
--- CMOMEGA for CM_SYNCH= 5079.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 98 COMPLETED. FREQUENCY= 816.683
--- CMOMEGA for CM_SYNCH= 5131.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 99 COMPLETED. FREQUENCY= 825.017
--- CMOMEGA for CM_SYNCH= 5183.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 100 COMPLETED. FREQUENCY= 833.350
--- CMOMEGA for CM_SYNCH= 5236.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 101 COMPLETED. FREQUENCY= 841.683
--- CMOMEGA for CM_SYNCH= 5288.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 102 COMPLETED. FREQUENCY= 850.017
--- CMOMEGA for CM_SYNCH= 5340.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 103 COMPLETED. FREQUENCY= 858.351
--- CMOMEGA for CM_SYNCH= 5393.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 104 COMPLETED. FREQUENCY= 866.684
--- CMOMEGA for CM_SYNCH= 5445.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 105 COMPLETED. FREQUENCY= 875.018
--- CMOMEGA for CM_SYNCH= 5497.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 106 COMPLETED. FREQUENCY= 883.351
--- CMOMEGA for CM_SYNCH= 5550.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 107 COMPLETED. FREQUENCY= 891.685
--- CMOMEGA for CM_SYNCH= 5602.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 108 COMPLETED. FREQUENCY= 900.018
--- CMOMEGA for CM_SYNCH= 5655.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 109 COMPLETED. FREQUENCY= 908.352
--- CMOMEGA for CM_SYNCH= 5707.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 110 COMPLETED. FREQUENCY= 916.685
--- CMOMEGA for CM_SYNCH= 5759.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 111 COMPLETED. FREQUENCY= 925.019
--- CMOMEGA for CM_SYNCH= 5812.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 112 COMPLETED. FREQUENCY= 933.352
--- CMOMEGA for CM_SYNCH= 5864.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 113 COMPLETED. FREQUENCY= 941.686
--- CMOMEGA for CM_SYNCH= 5916.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 114 COMPLETED. FREQUENCY= 950.019
--- CMOMEGA for CM_SYNCH= 5969.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 115 COMPLETED. FREQUENCY= 958.353
--- CMOMEGA for CM_SYNCH= 6021.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 116 COMPLETED. FREQUENCY= 966.686
--- CMOMEGA for CM_SYNCH= 6073.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 117 COMPLETED. FREQUENCY= 975.019
--- CMOMEGA for CM_SYNCH= 6126.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 118 COMPLETED. FREQUENCY= 983.353
--- CMOMEGA for CM_SYNCH= 6178.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 119 COMPLETED. FREQUENCY= 991.687
--- CMOMEGA for CM_SYNCH= 6231.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 120 COMPLETED. FREQUENCY= 1000.02
--- CMOMEGA for CM_SYNCH= 6283.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 121 COMPLETED. FREQUENCY= 1008.35
--- CMOMEGA for CM_SYNCH= 6335.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 122 COMPLETED. FREQUENCY= 1016.69
--- CMOMEGA for CM_SYNCH= 6388.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 123 COMPLETED. FREQUENCY= 1025.02
--- CMOMEGA for CM_SYNCH= 6440.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 124 COMPLETED. FREQUENCY= 1033.35
--- CMOMEGA for CM_SYNCH= 6492.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 125 COMPLETED. FREQUENCY= 1041.69
--- CMOMEGA for CM_SYNCH= 6545.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 126 COMPLETED. FREQUENCY= 1050.02
--- CMOMEGA for CM_SYNCH= 6597.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 127 COMPLETED. FREQUENCY= 1058.35
--- CMOMEGA for CM_SYNCH= 6649.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 128 COMPLETED. FREQUENCY= 1066.69
--- CMOMEGA for CM_SYNCH= 6702.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 129 COMPLETED. FREQUENCY= 1075.02
--- CMOMEGA for CM_SYNCH= 6754.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 130 COMPLETED. FREQUENCY= 1083.36
--- CMOMEGA for CM_SYNCH= 6806.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 131 COMPLETED. FREQUENCY= 1091.69
--- CMOMEGA for CM_SYNCH= 6859.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 132 COMPLETED. FREQUENCY= 1100.02
--- CMOMEGA for CM_SYNCH= 6911.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 133 COMPLETED. FREQUENCY= 1108.36
--- CMOMEGA for CM_SYNCH= 6964.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 134 COMPLETED. FREQUENCY= 1116.69
--- CMOMEGA for CM_SYNCH= 7016.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 135 COMPLETED. FREQUENCY= 1125.02
--- CMOMEGA for CM_SYNCH= 7068.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 136 COMPLETED. FREQUENCY= 1133.36
--- CMOMEGA for CM_SYNCH= 7121.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 137 COMPLETED. FREQUENCY= 1141.69
--- CMOMEGA for CM_SYNCH= 7173.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 138 COMPLETED. FREQUENCY= 1150.02
--- CMOMEGA for CM_SYNCH= 7225.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 139 COMPLETED. FREQUENCY= 1158.36
--- CMOMEGA for CM_SYNCH= 7278.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 140 COMPLETED. FREQUENCY= 1166.69
--- CMOMEGA for CM_SYNCH= 7330.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 141 COMPLETED. FREQUENCY= 1175.02
--- CMOMEGA for CM_SYNCH= 7382.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 142 COMPLETED. FREQUENCY= 1183.36
--- CMOMEGA for CM_SYNCH= 7435.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 143 COMPLETED. FREQUENCY= 1191.69
--- CMOMEGA for CM_SYNCH= 7487.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 144 COMPLETED. FREQUENCY= 1200.02
--- CMOMEGA for CM_SYNCH= 7540.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 145 COMPLETED. FREQUENCY= 1208.36
--- CMOMEGA for CM_SYNCH= 7592.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 146 COMPLETED. FREQUENCY= 1216.69
--- CMOMEGA for CM_SYNCH= 7644.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 147 COMPLETED. FREQUENCY= 1225.02
--- CMOMEGA for CM_SYNCH= 7697.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 148 COMPLETED. FREQUENCY= 1233.36
--- CMOMEGA for CM_SYNCH= 7749.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 149 COMPLETED. FREQUENCY= 1241.69
--- CMOMEGA for CM_SYNCH= 7801.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 150 COMPLETED. FREQUENCY= 1250.03
--- CMOMEGA for CM_SYNCH= 7854.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 151 COMPLETED. FREQUENCY= 1258.36
--- CMOMEGA for CM_SYNCH= 7906.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 152 COMPLETED. FREQUENCY= 1266.69
--- CMOMEGA for CM_SYNCH= 7958.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 153 COMPLETED. FREQUENCY= 1275.03
--- CMOMEGA for CM_SYNCH= 8011.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 154 COMPLETED. FREQUENCY= 1283.36
--- CMOMEGA for CM_SYNCH= 8063.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 155 COMPLETED. FREQUENCY= 1291.69
--- CMOMEGA for CM_SYNCH= 8115.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 156 COMPLETED. FREQUENCY= 1300.03
--- CMOMEGA for CM_SYNCH= 8168.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 157 COMPLETED. FREQUENCY= 1308.36
--- CMOMEGA for CM_SYNCH= 8220.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 158 COMPLETED. FREQUENCY= 1316.69
--- CMOMEGA for CM_SYNCH= 8273.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 159 COMPLETED. FREQUENCY= 1325.03
--- CMOMEGA for CM_SYNCH= 8325.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 160 COMPLETED. FREQUENCY= 1333.36
--- CMOMEGA for CM_SYNCH= 8377.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 161 COMPLETED. FREQUENCY= 1341.69
--- CMOMEGA for CM_SYNCH= 8430.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 162 COMPLETED. FREQUENCY= 1350.03
--- CMOMEGA for CM_SYNCH= 8482.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 163 COMPLETED. FREQUENCY= 1358.36
--- CMOMEGA for CM_SYNCH= 8534.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 164 COMPLETED. FREQUENCY= 1366.69
--- CMOMEGA for CM_SYNCH= 8587.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 165 COMPLETED. FREQUENCY= 1375.03
--- CMOMEGA for CM_SYNCH= 8639.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 166 COMPLETED. FREQUENCY= 1383.36
--- CMOMEGA for CM_SYNCH= 8691.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 167 COMPLETED. FREQUENCY= 1391.69
--- CMOMEGA for CM_SYNCH= 8744.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 168 COMPLETED. FREQUENCY= 1400.03
--- CMOMEGA for CM_SYNCH= 8796.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 169 COMPLETED. FREQUENCY= 1408.36
--- CMOMEGA for CM_SYNCH= 8849.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 170 COMPLETED. FREQUENCY= 1416.69
--- CMOMEGA for CM_SYNCH= 8901.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 171 COMPLETED. FREQUENCY= 1425.03
--- CMOMEGA for CM_SYNCH= 8953.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 172 COMPLETED. FREQUENCY= 1433.36
--- CMOMEGA for CM_SYNCH= 9006.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 173 COMPLETED. FREQUENCY= 1441.70
--- CMOMEGA for CM_SYNCH= 9058.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 174 COMPLETED. FREQUENCY= 1450.03
--- CMOMEGA for CM_SYNCH= 9110.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 175 COMPLETED. FREQUENCY= 1458.36
--- CMOMEGA for CM_SYNCH= 9163.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 176 COMPLETED. FREQUENCY= 1466.70
--- CMOMEGA for CM_SYNCH= 9215.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 177 COMPLETED. FREQUENCY= 1475.03
--- CMOMEGA for CM_SYNCH= 9267.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 178 COMPLETED. FREQUENCY= 1483.36
--- CMOMEGA for CM_SYNCH= 9320.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 179 COMPLETED. FREQUENCY= 1491.70
--- CMOMEGA for CM_SYNCH= 9372.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 180 COMPLETED. FREQUENCY= 1500.03
--- CMOMEGA for CM_SYNCH= 9425.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 181 COMPLETED. FREQUENCY= 1508.36
--- CMOMEGA for CM_SYNCH= 9477.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 182 COMPLETED. FREQUENCY= 1516.70
--- CMOMEGA for CM_SYNCH= 9529.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 183 COMPLETED. FREQUENCY= 1525.03
--- CMOMEGA for CM_SYNCH= 9582.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 184 COMPLETED. FREQUENCY= 1533.36
--- CMOMEGA for CM_SYNCH= 9634.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 185 COMPLETED. FREQUENCY= 1541.70
--- CMOMEGA for CM_SYNCH= 9686.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 186 COMPLETED. FREQUENCY= 1550.03
--- CMOMEGA for CM_SYNCH= 9739.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 187 COMPLETED. FREQUENCY= 1558.36
--- CMOMEGA for CM_SYNCH= 9791.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 188 COMPLETED. FREQUENCY= 1566.70
--- CMOMEGA for CM_SYNCH= 9843.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 189 COMPLETED. FREQUENCY= 1575.03
--- CMOMEGA for CM_SYNCH= 9896.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 190 COMPLETED. FREQUENCY= 1583.37
--- CMOMEGA for CM_SYNCH= 9948.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 191 COMPLETED. FREQUENCY= 1591.70
--- CMOMEGA for CM_SYNCH= 10001. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 192 COMPLETED. FREQUENCY= 1600.03
--- CMOMEGA for CM_SYNCH= 10053. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 193 COMPLETED. FREQUENCY= 1608.37
--- CMOMEGA for CM_SYNCH= 10106. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 194 COMPLETED. FREQUENCY= 1616.70
--- CMOMEGA for CM_SYNCH= 10158. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 195 COMPLETED. FREQUENCY= 1625.03
--- CMOMEGA for CM_SYNCH= 10210. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 196 COMPLETED. FREQUENCY= 1633.37
--- CMOMEGA for CM_SYNCH= 10263. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 197 COMPLETED. FREQUENCY= 1641.70
--- CMOMEGA for CM_SYNCH= 10315. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 198 COMPLETED. FREQUENCY= 1650.03
--- CMOMEGA for CM_SYNCH= 10367. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 199 COMPLETED. FREQUENCY= 1658.37
--- CMOMEGA for CM_SYNCH= 10420. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 200 COMPLETED. FREQUENCY= 1666.70
--- CMOMEGA for CM_SYNCH= 10472. 0.0000 0.0000
*** MAPDL BINARY FILE STATISTICS
BUFFER SIZE USED= 16384
0.250 MB WRITTEN ON ELEMENT MATRIX FILE: file0.emat
29.188 MB WRITTEN ON ELEMENT SAVED DATA FILE: file0.esav
34.062 MB WRITTEN ON ASSEMBLED MATRIX FILE: file0.full
413.000 MB WRITTEN ON RESULTS FILE: file0.rst
*************** Write FE CONNECTORS *********
WRITE OUT CONSTRAINT EQUATIONS TO FILE= file.ce
FINISH SOLUTION PROCESSING
***** ROUTINE COMPLETED ***** CP = 181.469
PRINTOUT RESUMED BY /GOP
*GET _WALLASOL FROM ACTI ITEM=TIME WALL VALUE= 17.7794444
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:46:46 JAN 08, 2025 CP= 181.469
wbnew--Unbalance Response
***** MAPDL RESULTS INTERPRETATION (POST1) *****
*** NOTE *** CP = 181.469 TIME= 17:46:46
The model contains an element type ( COMBI214 ) that operates entirely
in the nodal coordinate system. Viewing nodal displacements or forces
in other than the nodal coordinate system may be invalid. See the
elements manual description for more information.
*** NOTE *** CP = 181.469 TIME= 17:46:46
Reading results into the database (SET command) will update the current
displacement and force boundary conditions in the database with the
values from the results file for that load set. Note that any
subsequent solutions will use these values unless action is taken to
either SAVE the current values or not overwrite them (/EXIT,NOSAVE).
Set Encoding of XML File to:ISO-8859-1
Set Output of XML File to:
PARM, , , , , , , , , , , ,
, , , , , , ,
DATABASE WRITTEN ON FILE parm.xml
EXIT THE MAPDL POST1 DATABASE PROCESSOR
***** ROUTINE COMPLETED ***** CP = 181.484
PRINTOUT RESUMED BY /GOP
*GET _WALLDONE FROM ACTI ITEM=TIME WALL VALUE= 17.7794444
PARAMETER _PREPTIME = 0.000000000
PARAMETER _SOLVTIME = 215.0000000
PARAMETER _POSTTIME = 0.000000000
PARAMETER _TOTALTIM = 215.0000000
*GET _DLBRATIO FROM ACTI ITEM=SOLU DLBR VALUE= 0.00000000
*GET _COMBTIME FROM ACTI ITEM=SOLU COMB VALUE= 2.82741800
*GET _SSMODE FROM ACTI ITEM=SOLU SSMM VALUE= 2.00000000
*GET _NDOFS FROM ACTI ITEM=SOLU NDOF VALUE= 22781.0000
/FCLEAN COMMAND REMOVING ALL LOCAL FILES
--- Total number of nodes = 7698
--- Total number of elements = 2577
--- Element load balance ratio = 0
--- Time to combine distributed files = 2.827418
--- Sparse memory mode = 2
--- Number of DOF = 22781
EXIT MAPDL WITHOUT SAVING DATABASE
NUMBER OF WARNING MESSAGES ENCOUNTERED= 8
NUMBER OF ERROR MESSAGES ENCOUNTERED= 0
+--------------------- M A P D L S T A T I S T I C S ------------------------+
Release: 2024 R2 Build: 24.2 Update: UP20240603 Platform: WINDOWS x64
Date Run: 01/08/2025 Time: 17:46 Process ID: 20200
Operating System: Windows 11 (Build: 22631)
Processor Model: Intel(R) Xeon(R) Platinum 8171M CPU @ 2.60GHz
Compiler: Intel(R) Fortran Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) C/C++ Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) oneAPI Math Kernel Library Version 2023.1-Product Build 20230303
Number of machines requested : 1
Total number of cores available : 8
Number of physical cores available : 4
Number of processes requested : 4
Number of threads per process requested : 1
Total number of cores requested : 4 (Distributed Memory Parallel)
MPI Type: INTELMPI
MPI Version: Intel(R) MPI Library 2021.11 for Windows* OS
GPU Acceleration: Not Requested
Job Name: file0
Input File: dummy.dat
Core Machine Name Working Directory
-----------------------------------------------------
0 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB8DE
1 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB8DE
2 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB8DE
3 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\ScrB8DE
Latency time from master to core 1 = 3.259 microseconds
Latency time from master to core 2 = 3.806 microseconds
Latency time from master to core 3 = 3.655 microseconds
Communication speed from master to core 1 = 4923.05 MB/sec
Communication speed from master to core 2 = 4970.27 MB/sec
Communication speed from master to core 3 = 5002.00 MB/sec
Total CPU time for main thread : 181.1 seconds
Total CPU time summed for all threads : 181.7 seconds
Elapsed time spent obtaining a license : 0.5 seconds
Elapsed time spent pre-processing model (/PREP7) : 0.1 seconds
Elapsed time spent solution - preprocessing : 0.2 seconds
Elapsed time spent computing solution : 211.1 seconds
Elapsed time spent solution - postprocessing : 2.8 seconds
Elapsed time spent post-processing model (/POST1) : 0.0 seconds
Equation solver used : Sparse (unsymmetric)
Equation solver computational rate : 4.5 Gflops
Sum of disk space used on all processes : 3150.9 MB
Sum of memory used on all processes : 1125.0 MB
Sum of memory allocated on all processes : 3960.0 MB
Physical memory available : 32 GB
Total amount of I/O written to disk : 19.2 GB
Total amount of I/O read from disk : 16.0 GB
+------------------ E N D M A P D L S T A T I S T I C S -------------------+
*-----------------------------------------------------------------------------*
| |
| RUN COMPLETED |
| |
|-----------------------------------------------------------------------------|
| |
| Ansys MAPDL 2024 R2 Build 24.2 UP20240603 WINDOWS x64 |
| |
|-----------------------------------------------------------------------------|
| |
| Database Requested(-db) 1024 MB Scratch Memory Requested 1024 MB |
| Max Database Used(Master) 5 MB Max Scratch Used(Master) 280 MB |
| Max Database Used(Workers) 5 MB Max Scratch Used(Workers) 275 MB |
| Sum Database Used(All) 20 MB Sum Scratch Used(All) 1105 MB |
| |
|-----------------------------------------------------------------------------|
| |
| CP Time (sec) = 181.656 Time = 17:46:46 |
| Elapsed Time (sec) = 217.000 Date = 01/08/2025 |
| |
*-----------------------------------------------------------------------------*
You can save, archive and download the project using download_project_archive()
method.
[26]:
#wb.download_project_archive("test_name", show_progress=True)
Start a PyMechanical server for the 3D rotor model system and create a PyMechanical client session. The project directory is printed to verify the connection.
[27]:
server_port = wb.start_mechanical_server(system_name=sys_name[0])
[28]:
mechanical = connect_to_mechanical(ip='localhost', port=server_port)
[29]:
print(mechanical.project_directory)
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\
Read and execute the script rotor_3d.py
via the PyMechanical client to mesh and solve the 3D rotor model. The output of the script is printed.
[30]:
with open(scripts / "rotor_3d.py") as sf:
mech_script = sf.read()
mech_output = mechanical.run_python_script(mech_script)
print(mech_output)
{"Total Deformation": "0.7770134826146281 [mm]", "Total Deformation 2": "0.95766479418025063 [mm]"}
Specify the Mechanical directory for the Modal Campbell Analysis and fetch the working directory path. Download the solver output file (solve.out
) from the server to the client’s current working directory and print its contents.
[31]:
mechanical.run_python_script(f"solve_dir=ExtAPI.DataModel.AnalysisList[2].WorkingDir")
result_solve_dir_server = mechanical.run_python_script(f"solve_dir")
print(f"All solver files are stored on the server at: {result_solve_dir_server}")
All solver files are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-2\MECH\
[32]:
solve_out_path = os.path.join(result_solve_dir_server, "solve.out")
[33]:
def write_file_contents_to_console(path):
"""Write file contents to console."""
with open(path, "rt") as file:
for line in file:
print(line, end="")
[34]:
current_working_directory = os.getcwd()
mechanical.download(solve_out_path, target_dir=current_working_directory)
solve_out_local_path = os.path.join(current_working_directory, "solve.out")
write_file_contents_to_console(solve_out_local_path)
os.remove(solve_out_local_path)
Downloading dns:///127.0.0.1:55013:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-2\MECH\solve.out to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\solve.out: 100%|██████████| 82.4k/82.4k [00:00<?, ?B/s]
Ansys Mechanical Enterprise
*------------------------------------------------------------------*
| |
| W E L C O M E T O T H E A N S Y S (R) P R O G R A M |
| |
*------------------------------------------------------------------*
***************************************************************
* ANSYS MAPDL 2024 R2 LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2024 Ansys, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of Ansys, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the Ansys, Inc. online documentation or the Ansys, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* Ansys, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* Ansys, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the Ansys, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the Ansys, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
2024 R2
Point Releases and Patches installed:
Ansys Service Pack 2024 R2.01
Ansys Service Pack 2024 R2.02
Ansys Service Pack 2024 R2.03
Ansys, Inc. License Manager 2024 R2
Ansys, Inc. License Manager 2024 R2.01
Ansys, Inc. License Manager 2024 R2.02
Ansys, Inc. License Manager 2024 R2.03
Discovery 2024 R2
Discovery 2024 R2.01
Discovery 2024 R2.02
Discovery 2024 R2.03
Core WB Files 2024 R2
Core WB Files 2024 R2.01
Core WB Files 2024 R2.02
Core WB Files 2024 R2.03
SpaceClaim 2024 R2
SpaceClaim 2024 R2.01
SpaceClaim 2024 R2.02
SpaceClaim 2024 R2.03
Icepak (includes CFD-Post) 2024 R2
Icepak (includes CFD-Post) 2024 R2.01
Icepak (includes CFD-Post) 2024 R2.02
Icepak (includes CFD-Post) 2024 R2.03
CFD-Post only 2024 R2
CFD-Post only 2024 R2.01
CFD-Post only 2024 R2.02
CFD-Post only 2024 R2.03
CFX (includes CFD-Post) 2024 R2
CFX (includes CFD-Post) 2024 R2.01
CFX (includes CFD-Post) 2024 R2.02
CFX (includes CFD-Post) 2024 R2.03
Chemkin 2024 R2
Chemkin 2024 R2.01
Chemkin 2024 R2.02
Chemkin 2024 R2.03
EnSight 2024 R2
EnSight 2024 R2.01
EnSight 2024 R2.02
EnSight 2024 R2.03
FENSAP-ICE 2024 R2
FENSAP-ICE 2024 R2.01
FENSAP-ICE 2024 R2.02
FENSAP-ICE 2024 R2.03
Fluent (includes CFD-Post) 2024 R2
Fluent (includes CFD-Post) 2024 R2.01
Fluent (includes CFD-Post) 2024 R2.02
Fluent (includes CFD-Post) 2024 R2.03
Polyflow (includes CFD-Post) 2024 R2
Polyflow (includes CFD-Post) 2024 R2.01
Polyflow (includes CFD-Post) 2024 R2.02
Polyflow (includes CFD-Post) 2024 R2.03
Forte (includes EnSight) 2024 R2
Forte (includes EnSight) 2024 R2.01
Forte (includes EnSight) 2024 R2.02
Forte (includes EnSight) 2024 R2.03
ICEM CFD 2024 R2
ICEM CFD 2024 R2.01
ICEM CFD 2024 R2.02
ICEM CFD 2024 R2.03
TurboGrid 2024 R2
TurboGrid 2024 R2.01
TurboGrid 2024 R2.02
TurboGrid 2024 R2.03
Speos 2024 R2
Speos 2024 R2.01
Speos 2024 R2.02
Speos 2024 R2.03
Speos HPC 2024 R2
Speos HPC 2024 R2.01
Speos HPC 2024 R2.02
Speos HPC 2024 R2.03
optiSLang 2024 R2
optiSLang 2024 R2.01
optiSLang 2024 R2.02
optiSLang 2024 R2.03
Remote Solve Manager Standalone Services 2024 R2
Remote Solve Manager Standalone Services 2024 R2.01
Remote Solve Manager Standalone Services 2024 R2.02
Remote Solve Manager Standalone Services 2024 R2.03
Additive 2024 R2
Additive 2024 R2.01
Additive 2024 R2.02
Additive 2024 R2.03
Aqwa 2024 R2
Aqwa 2024 R2.01
Aqwa 2024 R2.02
Aqwa 2024 R2.03
Autodyn 2024 R2
Autodyn 2024 R2.01
Autodyn 2024 R2.02
Autodyn 2024 R2.03
Customization Files for User Programmable Features 2024 R2
Customization Files for User Programmable Features 2024 R2.01
Customization Files for User Programmable Features 2024 R2.02
Customization Files for User Programmable Features 2024 R2.03
LS-DYNA 2024 R2
LS-DYNA 2024 R2.01
LS-DYNA 2024 R2.02
LS-DYNA 2024 R2.03
Mechanical Products 2024 R2
Mechanical Products 2024 R2.01
Mechanical Products 2024 R2.02
Mechanical Products 2024 R2.03
Motion 2024 R2
Motion 2024 R2.01
Motion 2024 R2.02
Motion 2024 R2.03
Sherlock 2024 R2
Sherlock 2024 R2.01
Sherlock 2024 R2.02
Sherlock 2024 R2.03
Sound - SAS 2024 R2
Sound - SAS 2024 R2.01
Sound - SAS 2024 R2.02
Sound - SAS 2024 R2.03
ACIS Geometry Interface 2024 R2
ACIS Geometry Interface 2024 R2.01
ACIS Geometry Interface 2024 R2.02
ACIS Geometry Interface 2024 R2.03
AutoCAD Geometry Interface 2024 R2
AutoCAD Geometry Interface 2024 R2.01
AutoCAD Geometry Interface 2024 R2.02
AutoCAD Geometry Interface 2024 R2.03
Catia, Version 4 Geometry Interface 2024 R2
Catia, Version 4 Geometry Interface 2024 R2.01
Catia, Version 4 Geometry Interface 2024 R2.02
Catia, Version 4 Geometry Interface 2024 R2.03
Catia, Version 5 Geometry Interface 2024 R2
Catia, Version 5 Geometry Interface 2024 R2.01
Catia, Version 5 Geometry Interface 2024 R2.02
Catia, Version 5 Geometry Interface 2024 R2.03
Catia, Version 6 Geometry Interface 2024 R2
Catia, Version 6 Geometry Interface 2024 R2.01
Catia, Version 6 Geometry Interface 2024 R2.02
Catia, Version 6 Geometry Interface 2024 R2.03
Creo Elements/Direct Modeling Geometry Interface 2024 R2
Creo Elements/Direct Modeling Geometry Interface 2024 R2.01
Creo Elements/Direct Modeling Geometry Interface 2024 R2.02
Creo Elements/Direct Modeling Geometry Interface 2024 R2.03
Creo Parametric Geometry Interface 2024 R2
Creo Parametric Geometry Interface 2024 R2.01
Creo Parametric Geometry Interface 2024 R2.02
Creo Parametric Geometry Interface 2024 R2.03
Inventor Geometry Interface 2024 R2
Inventor Geometry Interface 2024 R2.01
Inventor Geometry Interface 2024 R2.02
Inventor Geometry Interface 2024 R2.03
JTOpen Geometry Interface 2024 R2
JTOpen Geometry Interface 2024 R2.01
JTOpen Geometry Interface 2024 R2.02
JTOpen Geometry Interface 2024 R2.03
NX Geometry Interface 2024 R2
NX Geometry Interface 2024 R2.01
NX Geometry Interface 2024 R2.02
NX Geometry Interface 2024 R2.03
Parasolid Geometry Interface 2024 R2
Parasolid Geometry Interface 2024 R2.01
Parasolid Geometry Interface 2024 R2.02
Parasolid Geometry Interface 2024 R2.03
Solid Edge Geometry Interface 2024 R2
Solid Edge Geometry Interface 2024 R2.01
Solid Edge Geometry Interface 2024 R2.02
Solid Edge Geometry Interface 2024 R2.03
SOLIDWORKS Geometry Interface 2024 R2
SOLIDWORKS Geometry Interface 2024 R2.01
SOLIDWORKS Geometry Interface 2024 R2.02
SOLIDWORKS Geometry Interface 2024 R2.03
***** MAPDL COMMAND LINE ARGUMENTS *****
BATCH MODE REQUESTED (-b) = NOLIST
INPUT FILE COPY MODE (-c) = COPY
DISTRIBUTED MEMORY PARALLEL REQUESTED
4 PARALLEL PROCESSES REQUESTED WITH SINGLE THREAD PER PROCESS
TOTAL OF 4 CORES REQUESTED
INPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3EE6\dummy.dat
OUTPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3EE6\solve.out
START-UP FILE MODE = NOREAD
STOP FILE MODE = NOREAD
RELEASE= 2024 R2 BUILD= 24.2 UP20240603 VERSION=WINDOWS x64
CURRENT JOBNAME=file0 17:48:34 JAN 08, 2025 CP= 0.078
PARAMETER _DS_PROGRESS = 999.0000000
/INPUT FILE= ds.dat LINE= 0
*** NOTE *** CP = 0.391 TIME= 17:48:34
The /CONFIG,NOELDB command is not valid in a distributed memory
parallel solution. Command is ignored.
*GET _WALLSTRT FROM ACTI ITEM=TIME WALL VALUE= 17.8094444
TITLE=
wbnew--Modal Campbell (C5)
ACT Extensions:
LSDYNA, 2024.2
5f463412-bd3e-484b-87e7-cbc0a665e474, wbex
/COM, ANSYSMotion, 2024.2
20180725-3f81-49eb-9f31-41364844c769, wbex
SET PARAMETER DIMENSIONS ON _WB_PROJECTSCRATCH_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_PROJECTSCRATCH_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3EE6\
SET PARAMETER DIMENSIONS ON _WB_SOLVERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_SOLVERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-2\MECH\
SET PARAMETER DIMENSIONS ON _WB_USERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_USERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\user_files\
--- Data in consistent NMM units. See Solving Units in the help system for more
MPA UNITS SPECIFIED FOR INTERNAL
LENGTH = MILLIMETERS (mm)
MASS = TONNE (Mg)
TIME = SECONDS (sec)
TEMPERATURE = CELSIUS (C)
TOFFSET = 273.0
FORCE = NEWTON (N)
HEAT = MILLIJOULES (mJ)
INPUT UNITS ARE ALSO SET TO MPA
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:34 JAN 08, 2025 CP= 0.422
wbnew--Modal Campbell (C5)
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
*********** Nodes for the whole assembly ***********
*********** Nodes for all Remote Points ***********
*********** Elements for Body 1 'Solid1' ***********
*********** Elements for Body 2 'Solid4' ***********
*********** Elements for Body 3 'Solid3' ***********
*********** Elements for Body 4 'Solid2' ***********
*********** Send User Defined Coordinate System(s) ***********
*********** Set Reference Temperature ***********
*********** Send Materials ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Element Component ***********
*********** Create Remote Point "PointMass_RemotePoint" ***********
*********** Create Remote Point "RemotePoint_Bearing1" ***********
*********** Create Remote Point "RemotePoint_Bearing2" ***********
*********** Create Remote Point "RemotePoint_FreeStanding1" ***********
*********** Create Remote Point "RemotePoint_FreeStanding2" ***********
*********** Create Remote Point "PointMass_RemotePoint2" ***********
*********** Create Bearing Connection "RemotePoint_FreeStanding1 To Multiple" *
Real Constant Set For Above Bearing Connection Is 13
*********** Create Bearing Connection "RemotePoint_FreeStanding2 To Multiple" *
Real Constant Set For Above Bearing Connection Is 14
*********** Construct Remote Mass Using Remote Attachment ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*** Create a component for all remote displacements ***
*********** Define Rotational Velocity ***********
***** ROUTINE COMPLETED ***** CP = 0.984
--- Number of total nodes = 17554
--- Number of contact elements = 134
--- Number of spring elements = 0
--- Number of bearing elements = 0
--- Number of solid elements = 10970
--- Number of condensed parts = 0
--- Number of total elements = 11111
*GET _WALLBSOL FROM ACTI ITEM=TIME WALL VALUE= 17.8094444
***** MAPDL SOLUTION ROUTINE *****
PERFORM A MODAL ANALYSIS
THIS WILL BE A NEW ANALYSIS
PARAMETER _THICKRATIO = 1.000000000
USE QRDAMP MODE EXTRACTION METHOD
EXTRACT 9 MODES
COMPUTE COMPLEX MODE SHAPES: YES
NORMALIZE THE MODE SHAPES TO THE MASS MATRIX
QRDAMP EIGENSOLVER OPTIONS
REUSE EIGENMODES FROM MODESYM FILE: Yes
SYMMETRIZE CONTACT STIFFNESS MATRIX AT ELEMENT LEVEL
CORIOLIS IN STATIONARY REFERENCE FRAME: GYROSCOPIC DAMPING MATRIX WILL BE CALCULATED
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
PRINTOUT RESUMED BY /GOP
WRITE MISC ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR THE ENTITIES DEFINED BY COMPONENT _ELMISC
EXPAND ALL EXTRACTED MODES
CALCULATE ELEMENT RESULTS AND NODAL DOF SOLUTION
CALCULATE STRESSES IN ANY DOWNSTREAM MODE SUPERPOSITION EXPANSION PASS
DO NOT COMBINE ELEMENT SAVE DATA FILES (.esav) AFTER DISTRIBUTED PARALLEL SOLUTION
DO NOT COMBINE ELEMENT MATRIX FILES (.emat) AFTER DISTRIBUTED PARALLEL SOLUTION
DO NOT COMBINE ASSEMBLED MATRIX FILES (.full) AFTER DISTRIBUTED PARALLEL SOLUTION
---------------- Campbell diagram point 1 ----------------
CMOMEGA on component= CM_ROTVELCITY132
OMEGAX, OMEGAY, OMEGAZ= 0.10472E-14 0.0000 0.0000
X1, Y1, Z1= 0.0000 0.0000 0.0000
*GET ANSINTER_ FROM ACTI ITEM=INT VALUE= 0.00000000
*IF ANSINTER_ ( = 0.00000 ) NE
0 ( = 0.00000 ) THEN
*ENDIF
*** NOTE *** CP = 1.047 TIME= 17:48:34
The automatic domain decomposition logic has selected the MESH domain
decomposition method with 4 processes per solution.
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 1.250 TIME= 17:48:34
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** WARNING *** CP = 1.266 TIME= 17:48:34
Make sure that the rotating element component 1 (component name
CM_ROTVELCITY132) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 1.266 TIME= 17:48:34
Make sure that the rotating element component 1 (component name
CM_ROTVELCITY132) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 1.266 TIME= 17:48:34
Make sure that the rotating element component 1 (component name
CM_ROTVELCITY132) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 1.266 TIME= 17:48:34
Make sure that the rotating element component 1 (component name
CM_ROTVELCITY132) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** NOTE *** CP = 1.328 TIME= 17:48:34
The model data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3
3EE6\file0.err ) for these warning messages.
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 3 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 4 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:34 JAN 08, 2025 CP= 1.328
wbnew--Modal Campbell (C5)
S O L U T I O N O P T I O N S
PROBLEM DIMENSIONALITY. . . . . . . . . . . . .3-D
DEGREES OF FREEDOM. . . . . . UX UY UZ ROTX ROTY ROTZ
ANALYSIS TYPE . . . . . . . . . . . . . . . . .MODAL
EXTRACTION METHOD. . . . . . . . . . . . . .QRDAMP
QRDAMP BASE EIGENSOLVER. . . . . . . . . . .BLOCK LANCZOS
COMPLEX SOLUTION OUTPUT. . . . . . . . . . .ON
OFFSET TEMPERATURE FROM ABSOLUTE ZERO . . . . . 273.15
NUMBER OF MODES TO EXTRACT. . . . . . . . . . . 9
GLOBALLY ASSEMBLED MATRIX . . . . . . . . . . .UNSYMMETRIC
NUMBER OF MODES TO EXPAND . . . . . . . . . . .ALL
ELEMENT RESULTS CALCULATION . . . . . . . . . .ON
*** WARNING *** CP = 1.359 TIME= 17:48:34
Material number 13 (used by element 13909) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 1.375 TIME= 17:48:34
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3
3EE6\file0.err ) for these warning messages.
*** NOTE *** CP = 1.422 TIME= 17:48:34
Internal nodes from 17555 to 17558 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
*** NOTE *** CP = 1.500 TIME= 17:48:34
Internal nodes from 17555 to 17558 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
*WARNING*: Some MPC/Lagrange based elements (e.g.13911) in real
constant set 5 overlap with other MPC/Lagrange based elements
(e.g.13998) in real constant set 11 which can cause overconstraint.
*** NOTE *** CP = 1.578 TIME= 17:48:34
Force-distributed-surface identified by real constant set 5 and
contact element type 5 has been set up. The pilot node 17545 is used
to apply the force which connects to other element 14049. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
*WARNING*: Certain contact elements (for example 13960&14047) overlap
each other.
****************************************
*** NOTE *** CP = 1.578 TIME= 17:48:34
Force-distributed-surface identified by real constant set 7 and
contact element type 7 has been set up. The pilot node 17546 is used
to apply the force which connects to other element 13909. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** NOTE *** CP = 1.578 TIME= 17:48:34
Force-distributed-surface identified by real constant set 9 and
contact element type 9 has been set up. The pilot node 17547 is used
to apply the force which connects to other element 13910. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** NOTE *** CP = 1.578 TIME= 17:48:34
Force-distributed-surface identified by real constant set 11 and
contact element type 11 has been set up. The pilot node 17550 is used
to apply the force. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
*WARNING*: Most likely no MPC equations will be built due to
overlapping with other pairs (i.e. Real constant set 5).
Please verify constraints (including rotational degrees of freedom)
on the pilot node by yourself.
****************************************
*** WARNING *** CP = 1.578 TIME= 17:48:34
Overconstraint may occur for Lagrange multiplier or MPC based contact
algorithm.
The reasons for possible overconstraint are:
*** WARNING *** CP = 1.578 TIME= 17:48:34
Certain contact elements (for example 13960 & 14047) overlap with
other.
*** WARNING *** CP = 1.578 TIME= 17:48:34
Certain contact pairs (for example 11 & 5) overlap with other.
****************************************
*** NOTE *** CP = 1.625 TIME= 17:48:34
Internal nodes from 17555 to 17558 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
D I S T R I B U T E D D O M A I N D E C O M P O S E R
...Number of elements: 11111
...Number of nodes: 17558
...Decompose to 4 CPU domains
...Element load balance ratio = 1.001
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 1
THERMAL STRAINS INCLUDED IN THE LOAD VECTOR . . YES
CORIOLIS EFFECT IN STATIONARY REF. FRAME . . . ON
REUSE EIGENMODES FOR QRDAMP SOLVER. . . . . . .YES
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
MISC ALL _ELMISC
*********** PRECISE MASS SUMMARY ***********
TOTAL RIGID BODY MASS MATRIX ABOUT ORIGIN
Translational mass | Coupled translational/rotational mass
0.38541E-02 0.0000 0.0000 | 0.0000 -0.31030E-07 0.13548E-06
0.0000 0.38541E-02 0.0000 | 0.31030E-07 0.0000 0.62248
0.0000 0.0000 0.38541E-02 | -0.13548E-06 -0.62248 0.0000
------------------------------------------ | ------------------------------------------
| Rotational mass (inertia)
| 2.7505 0.15255E-04 0.91712E-05
| 0.15255E-04 149.58 -0.22072E-05
| 0.91712E-05 -0.22072E-05 149.58
TOTAL MASS = 0.38541E-02
The mass principal axes coincide with the global Cartesian axes
CENTER OF MASS (X,Y,Z)= 161.51 -0.35152E-04 -0.80514E-05
TOTAL INERTIA ABOUT CENTER OF MASS
2.7505 -0.66264E-05 0.41594E-05
-0.66264E-05 49.038 -0.22072E-05
0.41594E-05 -0.22072E-05 49.038
The inertia principal axes coincide with the global Cartesian axes
*** MASS SUMMARY BY ELEMENT TYPE ***
TYPE MASS
1 0.788597E-03
2 0.274317E-03
3 0.768710E-03
4 0.621430E-03
15 0.140100E-02
Range of element maximum matrix coefficients in global coordinates
Maximum = 8468651.17 at element 4255.
Minimum = 35030 at element 13909.
*** ELEMENT MATRIX FORMULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 3498 SOLID187 0.562 0.000161
2 1408 SOLID187 0.266 0.000189
3 3842 SOLID187 0.688 0.000179
4 2222 SOLID187 0.297 0.000134
5 50 CONTA174 0.000 0.000000
6 1 TARGE170 0.000 0.000000
7 17 CONTA174 0.000 0.000000
8 1 TARGE170 0.000 0.000000
9 17 CONTA174 0.000 0.000000
10 1 TARGE170 0.000 0.000000
11 50 CONTA174 0.000 0.000000
12 1 TARGE170 0.000 0.000000
13 1 COMBI214 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 MASS21 0.000 0.000000
Time at end of element matrix formulation CP = 3.125.
*** GYROSCOPIC DAMPING MATRIX CALCULATED FOR LISTED ELEMENTS:
TYPE NUMBER ENAME
1 3498 SOLID187
2 1408 SOLID187
3 3842 SOLID187
4 2222 SOLID187
15 1 MASS21
BLOCK LANCZOS CALCULATION OF UP TO 9 EIGENVECTORS.
NUMBER OF EQUATIONS = 52628
MAXIMUM WAVEFRONT = 373
MAXIMUM MODES STORED = 9
MINIMUM EIGENVALUE = 0.00000E+00
MAXIMUM EIGENVALUE = 0.10000E+31
Memory allocated on only this MPI rank (rank 0)
-------------------------------------------------------------------
Equation solver memory allocated = 59.970 MB
Equation solver memory required for in-core mode = 57.160 MB
Equation solver memory required for out-of-core mode = 27.165 MB
Total (solver and non-solver) memory allocated = 659.516 MB
Total memory summed across all MPI ranks on this machines
-------------------------------------------------------------------
Equation solver memory allocated = 252.404 MB
Equation solver memory required for in-core mode = 240.579 MB
Equation solver memory required for out-of-core mode = 108.683 MB
Total (solver and non-solver) memory allocated = 1739.095 MB
*** NOTE *** CP = 4.141 TIME= 17:48:37
The Distributed Sparse Matrix Solver used by the Block Lanczos
eigensolver is currently running in the in-core memory mode. This
memory mode uses the most amount of memory in order to avoid using the
hard drive as much as possible, which most often results in the
fastest solution time. This mode is recommended if enough physical
memory is present to accommodate all of the solver data.
Process memory required for in-core LANCZOS Workspace = 62.763954 MB
Process memory required for out-of-core LANCZOS Workspace = 1.965889 MB
Total memory required for in-core LANCZOS Workspace = 232.133514 MB
Total memory required for out-of-core LANCZOS Workspace = 7.413025 MB
Lanczos Memory Mode : INCORE
>> Shift # 1 : | : 9 Eigenvalues Converged
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:38 JAN 08, 2025 CP= 5.078
wbnew--Modal Campbell (C5)
*** UNDAMPED FREQUENCIES FROM BLOCK LANCZOS ITERATION ***
MODE FREQUENCY (HERTZ)
1 189.1898145226
2 207.9876132983
3 639.0605422493
4 658.8539992383
5 735.3975262256
6 810.6490114470
7 991.0151537309
8 1776.767736641
9 1777.727070344
Time at end of state-space matrix formulation CP = 5.140625.
***** DAMPED FREQUENCIES FROM REDUCED DAMPED EIGENSOLVER *****
COMPLEX FREQUENCY (HERTZ)
MODE STABILITY FREQUENCY MODAL DAMPING RATIO
1 0.0000000 189.18981 j 0.0000000
2 0.0000000 207.98761 j 0.0000000
3 0.0000000 639.06054 j 0.0000000
4 0.0000000 658.85400 j 0.0000000
5 0.0000000 735.39753 j 0.0000000
6 0.0000000 810.64901 j 0.0000000
7 0.0000000 991.01515 j 0.0000000
8 0.0000000 1776.7677 j 0.0000000
9 0.0000000 1777.7271 j 0.0000000
Time at end of eigenproblem resolution CP = 5.15625.
Time at end of mode shape normalization CP = 5.21875.
*** ELEMENT RESULT CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 3498 SOLID187 0.188 0.000054
2 1408 SOLID187 0.078 0.000055
3 3842 SOLID187 0.266 0.000069
4 2222 SOLID187 0.156 0.000070
5 50 CONTA174 0.000 0.000000
7 17 CONTA174 0.000 0.000000
9 17 CONTA174 0.000 0.000000
11 50 CONTA174 0.000 0.000000
13 1 COMBI214 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 MASS21 0.000 0.000000
*** NODAL LOAD CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 3498 SOLID187 0.031 0.000009
2 1408 SOLID187 0.000 0.000000
3 3842 SOLID187 0.000 0.000000
4 2222 SOLID187 0.031 0.000014
5 50 CONTA174 0.000 0.000000
7 17 CONTA174 0.000 0.000000
9 17 CONTA174 0.000 0.000000
11 50 CONTA174 0.000 0.000000
13 1 COMBI214 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 MASS21 0.000 0.000000
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:42 JAN 08, 2025 CP= 9.188
wbnew--Modal Campbell (C5)
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 189.190 0.52857E-02 -0.63546E-05 0.190045 0.403808E-10 0.203904E-01 0.104775E-07
2 207.988 0.48080E-02 0.47074E-05 0.140783 0.221596E-10 0.315800E-01 0.574968E-08
3 639.061 0.15648E-02 -0.33437E-04 1.000000 0.111805E-08 0.596142 0.290096E-06
4 658.854 0.15178E-02 0.26430E-04 0.790452 0.698571E-09 0.948888 0.181256E-06
5 735.398 0.13598E-02 -0.13379E-05 0.040011 0.178987E-11 0.949792 0.464413E-09
6 810.649 0.12336E-02 0.97198E-05 0.290687 0.944741E-10 0.997497 0.245129E-07
7 991.015 0.10091E-02 -0.24351E-06 0.007283 0.592969E-13 0.997527 0.153856E-10
8 1776.77 0.56282E-03 -0.20950E-05 0.062656 0.438915E-11 0.999743 0.113884E-08
9 1777.73 0.56252E-03 0.71311E-06 0.021327 0.508526E-12 1.00000 0.131946E-09
-----------------------------------------------------------------------------------------------------------------
sum 0.198038E-08 0.513843E-06
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 189.190 0.52857E-02 0.23925E-01 0.692522 0.572424E-03 0.150703 0.148525
2 207.988 0.48080E-02 0.23247E-01 0.672883 0.540418E-03 0.292979 0.140221
3 639.061 0.15648E-02 0.22763E-01 0.658885 0.518166E-03 0.429397 0.134447
4 658.854 0.15178E-02 0.28638E-01 0.828920 0.820118E-03 0.645311 0.212794
5 735.398 0.13598E-02 -0.12376E-01 0.358228 0.153169E-03 0.685636 0.397422E-01
6 810.649 0.12336E-02 -0.34548E-01 1.000000 0.119358E-02 0.999870 0.309694
7 991.015 0.10091E-02 0.84868E-06 0.000025 0.720260E-12 0.999870 0.186884E-09
8 1776.77 0.56282E-03 -0.46974E-03 0.013597 0.220653E-06 0.999928 0.572522E-04
9 1777.73 0.56252E-03 0.52196E-03 0.015108 0.272442E-06 1.00000 0.706898E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.379836E-02 0.985551
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 189.190 0.52857E-02 0.23947E-01 0.693263 0.573441E-03 0.150972 0.148789
2 207.988 0.48080E-02 -0.23222E-01 0.672297 0.539281E-03 0.292951 0.139926
3 639.061 0.15648E-02 0.22786E-01 0.659668 0.519209E-03 0.429646 0.134718
4 658.854 0.15178E-02 0.28635E-01 0.829001 0.819978E-03 0.645526 0.212757
5 735.398 0.13598E-02 0.12360E-01 0.357826 0.152769E-03 0.685746 0.396385E-01
6 810.649 0.12336E-02 0.34542E-01 1.000000 0.119314E-02 0.999870 0.309581
7 991.015 0.10091E-02 -0.13381E-05 0.000039 0.179057E-11 0.999870 0.464594E-09
8 1776.77 0.56282E-03 -0.32818E-03 0.009501 0.107699E-06 0.999898 0.279443E-04
9 1777.73 0.56252E-03 -0.62130E-03 0.017987 0.386016E-06 1.00000 0.100158E-03
-----------------------------------------------------------------------------------------------------------------
sum 0.379831E-02 0.985538
-----------------------------------------------------------------------------------------------------------------
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:42 JAN 08, 2025 CP= 9.188
wbnew--Modal Campbell (C5)
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 189.190 0.52857E-02 0.0000 0.000000 0.00000 0.00000 0.00000
2 207.988 0.48080E-02 0.0000 0.000000 0.00000 0.00000 0.00000
3 639.061 0.15648E-02 0.0000 0.000000 0.00000 0.00000 0.00000
4 658.854 0.15178E-02 0.0000 0.000000 0.00000 0.00000 0.00000
5 735.398 0.13598E-02 0.0000 0.000000 0.00000 0.00000 0.00000
6 810.649 0.12336E-02 0.0000 0.000000 0.00000 0.00000 0.00000
7 991.015 0.10091E-02 0.0000 0.000000 0.00000 0.00000 0.00000
8 1776.77 0.56282E-03 0.0000 0.000000 0.00000 0.00000 0.00000
9 1777.73 0.56252E-03 0.0000 0.000000 0.00000 0.00000 0.00000
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 189.190 0.52857E-02 0.0000 0.000000 0.00000 0.00000 0.00000
2 207.988 0.48080E-02 0.0000 0.000000 0.00000 0.00000 0.00000
3 639.061 0.15648E-02 0.0000 0.000000 0.00000 0.00000 0.00000
4 658.854 0.15178E-02 0.0000 0.000000 0.00000 0.00000 0.00000
5 735.398 0.13598E-02 0.0000 0.000000 0.00000 0.00000 0.00000
6 810.649 0.12336E-02 0.0000 0.000000 0.00000 0.00000 0.00000
7 991.015 0.10091E-02 0.0000 0.000000 0.00000 0.00000 0.00000
8 1776.77 0.56282E-03 0.0000 0.000000 0.00000 0.00000 0.00000
9 1777.73 0.56252E-03 0.0000 0.000000 0.00000 0.00000 0.00000
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 189.190 0.52857E-02 0.0000 0.000000 0.00000 0.00000 0.00000
2 207.988 0.48080E-02 0.0000 0.000000 0.00000 0.00000 0.00000
3 639.061 0.15648E-02 0.0000 0.000000 0.00000 0.00000 0.00000
4 658.854 0.15178E-02 0.0000 0.000000 0.00000 0.00000 0.00000
5 735.398 0.13598E-02 0.0000 0.000000 0.00000 0.00000 0.00000
6 810.649 0.12336E-02 0.0000 0.000000 0.00000 0.00000 0.00000
7 991.015 0.10091E-02 0.0000 0.000000 0.00000 0.00000 0.00000
8 1776.77 0.56282E-03 0.0000 0.000000 0.00000 0.00000 0.00000
9 1777.73 0.56252E-03 0.0000 0.000000 0.00000 0.00000 0.00000
*** MAPDL BINARY FILE STATISTICS
BUFFER SIZE USED= 16384
59.375 MB WRITTEN ON ELEMENT MATRIX FILE: file0.emat
1.500 MB WRITTEN ON ELEMENT SAVED DATA FILE: file0.esav
19.312 MB WRITTEN ON ASSEMBLED MATRIX FILE: file0.full
2.625 MB WRITTEN ON MODAL MATRIX FILE: file0.mode
3.125 MB WRITTEN ON RESULTS FILE: file0.rst
*************** Write FE CONNECTORS *********
WRITE OUT CONSTRAINT EQUATIONS TO FILE= file.ce
---------------- Campbell diagram point 2 ----------------
CMOMEGA on component= CM_ROTVELCITY132
OMEGAX, OMEGAY, OMEGAZ= 5236.0 0.0000 0.0000
X1, Y1, Z1= 0.0000 0.0000 0.0000
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 9.453 TIME= 17:48:42
Material number 13 (used by element 13909) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 9.469 TIME= 17:48:42
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3
3EE6\file0.err ) for these warning messages.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:43 JAN 08, 2025 CP= 9.578
wbnew--Modal Campbell (C5)
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 2
THERMAL STRAINS INCLUDED IN THE LOAD VECTOR . . YES
CORIOLIS EFFECT IN STATIONARY REF. FRAME . . . ON
REUSE EIGENMODES FOR QRDAMP SOLVER. . . . . . .YES
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
MISC ALL _ELMISC
*** GYROSCOPIC DAMPING MATRIX CALCULATED FOR LISTED ELEMENTS:
TYPE NUMBER ENAME
1 3498 SOLID187
2 1408 SOLID187
3 3842 SOLID187
4 2222 SOLID187
15 1 MASS21
*** WARNING *** CP = 11.062 TIME= 17:48:44
The file0.modesym file does exist and is being used for the QR damp
eigensolution since the ReuseKey is set to ON on the QRDOPT command.
Time at end of state-space matrix formulation CP = 11.109375.
***** DAMPED FREQUENCIES FROM REDUCED DAMPED EIGENSOLVER *****
COMPLEX FREQUENCY (HERTZ)
MODE STABILITY FREQUENCY MODAL DAMPING RATIO
1 0.0000000 168.31116 j 0.0000000
2 0.0000000 231.85840 j 0.0000000
3 0.0000000 628.55118 j 0.0000000
4 0.0000000 656.05083 j 0.0000000
5 0.0000000 754.87782 j 0.0000000
6 0.0000000 812.95857 j 0.0000000
7 0.0000000 991.01515 j 0.0000000
8 0.0000000 1759.5985 j 0.0000000
9 0.0000000 1795.3315 j 0.0000000
Time at end of eigenproblem resolution CP = 11.109375.
Time at end of mode shape normalization CP = 11.140625.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:48 JAN 08, 2025 CP= 15.188
wbnew--Modal Campbell (C5)
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 168.311 0.59414E-02 -0.46580E-05 0.130375 0.216971E-10 0.125821E-01 0.562969E-08
2 231.858 0.43130E-02 0.36622E-05 0.102502 0.134114E-10 0.203593E-01 0.347982E-08
3 628.551 0.15910E-02 -0.35728E-04 1.000000 0.127648E-08 0.760583 0.331204E-06
4 656.051 0.15243E-02 0.17776E-04 0.497536 0.315982E-09 0.943820 0.819870E-07
5 754.878 0.13247E-02 -0.97387E-06 0.027258 0.948422E-12 0.944370 0.246084E-09
6 812.959 0.12301E-02 0.96797E-05 0.270928 0.936962E-10 0.998704 0.243111E-07
7 991.015 0.10091E-02 -0.24353E-06 0.006816 0.593052E-13 0.998739 0.153878E-10
8 1759.60 0.56831E-03 -0.13638E-05 0.038172 0.185994E-11 0.999817 0.482593E-09
9 1795.33 0.55700E-03 0.56119E-06 0.015707 0.314932E-12 1.00000 0.817144E-10
-----------------------------------------------------------------------------------------------------------------
sum 0.172445E-08 0.447437E-06
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 168.311 0.59414E-02 0.19135E-01 0.560149 0.366137E-03 0.109838 0.950004E-01
2 231.858 0.43130E-02 0.18710E-01 0.547714 0.350061E-03 0.214853 0.908293E-01
3 628.551 0.15910E-02 0.16055E-01 0.469987 0.257755E-03 0.292178 0.668790E-01
4 656.051 0.15243E-02 0.32770E-01 0.959298 0.107385E-02 0.614324 0.278629
5 754.878 0.13247E-02 -0.10885E-01 0.318642 0.118479E-03 0.649866 0.307414E-01
6 812.959 0.12301E-02 -0.34160E-01 1.000000 0.116691E-02 0.999929 0.302774
7 991.015 0.10091E-02 0.84193E-06 0.000025 0.708844E-12 0.999929 0.183922E-09
8 1759.60 0.56831E-03 -0.37988E-03 0.011120 0.144305E-06 0.999972 0.374425E-04
9 1795.33 0.55700E-03 0.30488E-03 0.008925 0.929491E-07 1.00000 0.241172E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.333343E-02 0.864915
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 168.311 0.59414E-02 0.19152E-01 0.560756 0.366793E-03 0.110036 0.951707E-01
2 231.858 0.43130E-02 -0.18690E-01 0.547230 0.349311E-03 0.214829 0.906348E-01
3 628.551 0.15910E-02 0.16076E-01 0.470708 0.258451E-03 0.292363 0.670594E-01
4 656.051 0.15243E-02 0.32772E-01 0.959543 0.107400E-02 0.614558 0.278667
5 754.878 0.13247E-02 0.10870E-01 0.318261 0.118152E-03 0.650003 0.306566E-01
6 812.959 0.12301E-02 0.34154E-01 1.000000 0.116647E-02 0.999939 0.302661
7 991.015 0.10091E-02 -0.13323E-05 0.000039 0.177492E-11 0.999939 0.460533E-09
8 1759.60 0.56831E-03 -0.26018E-03 0.007618 0.676911E-07 0.999960 0.175636E-04
9 1795.33 0.55700E-03 -0.36713E-03 0.010749 0.134781E-06 1.00000 0.349713E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.333338E-02 0.864901
-----------------------------------------------------------------------------------------------------------------
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:48 JAN 08, 2025 CP= 15.188
wbnew--Modal Campbell (C5)
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 168.311 0.59414E-02 0.27602E-05 0.177343 0.761846E-11 0.268019E-01 0.197674E-08
2 231.858 0.43130E-02 -0.49117E-05 0.315584 0.241252E-10 0.111675 0.625969E-08
3 628.551 0.15910E-02 0.91224E-06 0.058613 0.832191E-12 0.114602 0.215926E-09
4 656.051 0.15243E-02 -0.84342E-06 0.054191 0.711364E-12 0.117105 0.184576E-09
5 754.878 0.13247E-02 0.15564E-04 1.000000 0.242237E-09 0.969301 0.628527E-07
6 812.959 0.12301E-02 -0.24239E-05 0.155739 0.587536E-11 0.989971 0.152446E-08
7 991.015 0.10091E-02 0.0000 0.000000 0.00000 0.989971 0.00000
8 1759.60 0.56831E-03 -0.44662E-06 0.028696 0.199470E-12 0.990673 0.517559E-10
9 1795.33 0.55700E-03 0.16283E-05 0.104618 0.265127E-11 1.00000 0.687918E-09
-----------------------------------------------------------------------------------------------------------------
sum 0.284251E-09 0.737537E-07
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 168.311 0.59414E-02 0.13033E-01 0.841970 0.169862E-03 0.362167 0.440737E-01
2 231.858 0.43130E-02 0.15479E-01 1.000000 0.239609E-03 0.873042 0.621707E-01
3 628.551 0.15910E-02 0.64212E-02 0.414822 0.412313E-04 0.960952 0.106982E-01
4 656.051 0.15243E-02 0.28617E-02 0.184876 0.818960E-05 0.978413 0.212493E-02
5 754.878 0.13247E-02 -0.15046E-02 0.097203 0.226391E-05 0.983240 0.587411E-03
6 812.959 0.12301E-02 -0.27554E-02 0.178008 0.759249E-05 0.999428 0.197000E-02
7 991.015 0.10091E-02 0.11911E-06 0.000008 0.141871E-13 0.999428 0.368108E-11
8 1759.60 0.56831E-03 -0.43482E-03 0.028090 0.189064E-06 0.999831 0.490559E-04
9 1795.33 0.55700E-03 0.28130E-03 0.018172 0.791285E-07 1.00000 0.205312E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.469017E-03 0.121695
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 168.311 0.59414E-02 -0.13019E-01 0.840341 0.169507E-03 0.361312 0.439815E-01
2 231.858 0.43130E-02 0.15493E-01 1.000000 0.240036E-03 0.872959 0.622814E-01
3 628.551 0.15910E-02 -0.64172E-02 0.414199 0.411807E-04 0.960738 0.106850E-01
4 656.051 0.15243E-02 -0.28652E-02 0.184934 0.820938E-05 0.978236 0.213006E-02
5 754.878 0.13247E-02 -0.15171E-02 0.097924 0.230173E-05 0.983143 0.597224E-03
6 812.959 0.12301E-02 -0.27577E-02 0.177997 0.760505E-05 0.999353 0.197326E-02
7 991.015 0.10091E-02 0.15514E-07 0.000001 0.240684E-15 0.999353 0.624495E-13
8 1759.60 0.56831E-03 0.51283E-03 0.033101 0.262998E-06 0.999914 0.682393E-04
9 1795.33 0.55700E-03 0.20130E-03 0.012993 0.405235E-07 1.00000 0.105145E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.469143E-03 0.121727
-----------------------------------------------------------------------------------------------------------------
---------------- Campbell diagram point 3 ----------------
CMOMEGA on component= CM_ROTVELCITY132
OMEGAX, OMEGAY, OMEGAZ= 10472. 0.0000 0.0000
X1, Y1, Z1= 0.0000 0.0000 0.0000
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 15.453 TIME= 17:48:48
Material number 13 (used by element 13909) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 15.469 TIME= 17:48:48
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3
3EE6\file0.err ) for these warning messages.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:48 JAN 08, 2025 CP= 15.547
wbnew--Modal Campbell (C5)
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 3
THERMAL STRAINS INCLUDED IN THE LOAD VECTOR . . YES
CORIOLIS EFFECT IN STATIONARY REF. FRAME . . . ON
REUSE EIGENMODES FOR QRDAMP SOLVER. . . . . . .YES
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
MISC ALL _ELMISC
*** GYROSCOPIC DAMPING MATRIX CALCULATED FOR LISTED ELEMENTS:
TYPE NUMBER ENAME
1 3498 SOLID187
2 1408 SOLID187
3 3842 SOLID187
4 2222 SOLID187
15 1 MASS21
*** WARNING *** CP = 16.766 TIME= 17:48:49
The file0.modesym file does exist and is being used for the QR damp
eigensolution since the ReuseKey is set to ON on the QRDOPT command.
Time at end of state-space matrix formulation CP = 16.8125.
***** DAMPED FREQUENCIES FROM REDUCED DAMPED EIGENSOLVER *****
COMPLEX FREQUENCY (HERTZ)
MODE STABILITY FREQUENCY MODAL DAMPING RATIO
1 0.0000000 144.17300 j 0.0000000
2 0.0000000 264.17611 j 0.0000000
3 0.0000000 605.75395 j 0.0000000
4 0.0000000 653.14028 j 0.0000000
5 0.0000000 799.09507 j 0.0000000
6 0.0000000 819.76611 j 0.0000000
7 0.0000000 991.01515 j 0.0000000
8 0.0000000 1742.3461 j 0.0000000
9 0.0000000 1813.9033 j 0.0000000
Time at end of eigenproblem resolution CP = 16.8125.
Time at end of mode shape normalization CP = 16.890625.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:54 JAN 08, 2025 CP= 20.469
wbnew--Modal Campbell (C5)
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 144.173 0.69361E-02 -0.39132E-05 0.111188 0.153135E-10 0.102978E-01 0.397334E-08
2 264.176 0.37854E-02 0.33390E-05 0.094871 0.111486E-10 0.177949E-01 0.289271E-08
3 605.754 0.16508E-02 -0.35195E-04 1.000000 0.123867E-08 0.850765 0.321395E-06
4 653.140 0.15311E-02 0.11314E-04 0.321458 0.127999E-09 0.936840 0.332115E-07
5 799.095 0.12514E-02 -0.66309E-06 0.018841 0.439688E-12 0.937136 0.114085E-09
6 819.766 0.12199E-02 0.95671E-05 0.271832 0.915290E-10 0.998686 0.237488E-07
7 991.015 0.10091E-02 -0.24358E-06 0.006921 0.593333E-13 0.998726 0.153950E-10
8 1742.35 0.57394E-03 -0.12339E-05 0.035059 0.152248E-11 0.999750 0.395034E-09
9 1813.90 0.55130E-03 0.60984E-06 0.017328 0.371907E-12 1.00000 0.964975E-10
-----------------------------------------------------------------------------------------------------------------
sum 0.148706E-08 0.385843E-06
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 144.173 0.69361E-02 0.17737E-01 0.515341 0.314607E-03 0.101741 0.816303E-01
2 264.176 0.37854E-02 0.18052E-01 0.524485 0.325871E-03 0.207125 0.845528E-01
3 605.754 0.16508E-02 0.99010E-02 0.287667 0.980305E-04 0.238828 0.254357E-01
4 653.140 0.15311E-02 0.34418E-01 1.000000 0.118462E-02 0.621925 0.307371
5 799.095 0.12514E-02 -0.78991E-02 0.229501 0.623950E-04 0.642103 0.161895E-01
6 819.766 0.12199E-02 -0.33264E-01 0.966451 0.110647E-02 0.999926 0.287092
7 991.015 0.10091E-02 0.81202E-06 0.000024 0.659381E-12 0.999926 0.171088E-09
8 1742.35 0.57394E-03 -0.41952E-03 0.012189 0.175998E-06 0.999983 0.456656E-04
9 1813.90 0.55130E-03 0.23206E-03 0.006742 0.538521E-07 1.00000 0.139728E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.309223E-02 0.802331
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
1 144.173 0.69361E-02 0.17753E-01 0.515738 0.315176E-03 0.101937 0.817778E-01
2 264.176 0.37854E-02 -0.18032E-01 0.523836 0.325152E-03 0.207099 0.843661E-01
3 605.754 0.16508E-02 0.99205E-02 0.288194 0.984157E-04 0.238930 0.255356E-01
4 653.140 0.15311E-02 0.34423E-01 1.000000 0.118494E-02 0.622170 0.307452
5 799.095 0.12514E-02 0.78818E-02 0.228970 0.621232E-04 0.642263 0.161189E-01
6 819.766 0.12199E-02 0.33255E-01 0.966084 0.110592E-02 0.999948 0.286951
7 991.015 0.10091E-02 -0.13038E-05 0.000038 0.169979E-11 0.999948 0.441040E-09
8 1742.35 0.57394E-03 -0.28201E-03 0.008192 0.795280E-07 0.999974 0.206349E-04
9 1813.90 0.55130E-03 -0.28432E-03 0.008260 0.808360E-07 1.00000 0.209743E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.309189E-02 0.802243
-----------------------------------------------------------------------------------------------------------------
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:54 JAN 08, 2025 CP= 20.469
wbnew--Modal Campbell (C5)
***** PARTICIPATION FACTOR CALCULATION ***** X DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 144.173 0.69361E-02 0.30542E-05 0.143500 0.932839E-11 0.176607E-01 0.242041E-08
2 264.176 0.37854E-02 -0.64341E-05 0.302298 0.413973E-10 0.960350E-01 0.107412E-07
3 605.754 0.16508E-02 0.16926E-05 0.079525 0.286494E-11 0.101459 0.743357E-09
4 653.140 0.15311E-02 -0.12468E-05 0.058579 0.155446E-11 0.104402 0.403331E-09
5 799.095 0.12514E-02 0.21284E-04 1.000000 0.453005E-09 0.962040 0.117540E-06
6 819.766 0.12199E-02 -0.40648E-05 0.190981 0.165228E-10 0.993321 0.428712E-08
7 991.015 0.10091E-02 0.10926E-08 0.000051 0.119367E-17 0.993321 0.309719E-15
8 1742.35 0.57394E-03 -0.40099E-06 0.018840 0.160790E-12 0.993626 0.417197E-10
9 1813.90 0.55130E-03 0.18349E-05 0.086211 0.336689E-11 1.00000 0.873598E-09
-----------------------------------------------------------------------------------------------------------------
sum 0.528201E-09 0.137051E-06
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Y DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 144.173 0.69361E-02 0.14118E-01 0.793355 0.199306E-03 0.285284 0.517132E-01
2 264.176 0.37854E-02 0.17795E-01 1.000000 0.316653E-03 0.738537 0.821611E-01
3 605.754 0.16508E-02 0.91945E-02 0.516697 0.845389E-04 0.859545 0.219351E-01
4 653.140 0.15311E-02 0.80698E-02 0.453494 0.651218E-04 0.952760 0.168970E-01
5 799.095 0.12514E-02 -0.22088E-02 0.124125 0.487869E-05 0.959743 0.126586E-02
6 819.766 0.12199E-02 -0.52736E-02 0.296360 0.278113E-04 0.999552 0.721613E-02
7 991.015 0.10091E-02 0.22680E-06 0.000013 0.514389E-13 0.999552 0.133467E-10
8 1742.35 0.57394E-03 -0.50724E-03 0.028505 0.257290E-06 0.999920 0.667582E-04
9 1813.90 0.55130E-03 0.23574E-03 0.013248 0.555722E-07 1.00000 0.144191E-04
-----------------------------------------------------------------------------------------------------------------
sum 0.698622E-03 0.181270
-----------------------------------------------------------------------------------------------------------------
***** PARTICIPATION FACTOR CALCULATION ***** Z DIRECTION
CUMULATIVE RATIO EFF.MASS
MODE FREQUENCY PERIOD PARTIC.FACTOR RATIO EFFECTIVE MASS MASS FRACTION TO TOTAL MASS
IMAGINARY COMPONENT
1 144.173 0.69361E-02 -0.14103E-01 0.791822 0.198890E-03 0.284516 0.516053E-01
2 264.176 0.37854E-02 0.17811E-01 1.000000 0.317218E-03 0.738303 0.823075E-01
3 605.754 0.16508E-02 -0.91887E-02 0.515912 0.844322E-04 0.859086 0.219074E-01
4 653.140 0.15311E-02 -0.80724E-02 0.453236 0.651636E-04 0.952304 0.169078E-01
5 799.095 0.12514E-02 -0.22476E-02 0.126192 0.505151E-05 0.959530 0.131070E-02
6 819.766 0.12199E-02 -0.52827E-02 0.296607 0.279074E-04 0.999452 0.724106E-02
7 991.015 0.10091E-02 0.24558E-07 0.000001 0.603096E-15 0.999452 0.156484E-12
8 1742.35 0.57394E-03 0.59386E-03 0.033343 0.352671E-06 0.999957 0.915064E-04
9 1813.90 0.55130E-03 0.17375E-03 0.009755 0.301877E-07 1.00000 0.783271E-05
-----------------------------------------------------------------------------------------------------------------
sum 0.699045E-03 0.181379
-----------------------------------------------------------------------------------------------------------------
*GET _NMODALSOLPROC FROM ACTI ITEM=NUMC VALUE= 4.00000000
FINISH SOLUTION PROCESSING
***** ROUTINE COMPLETED ***** CP = 20.875
PRINTOUT RESUMED BY /GOP
*GET _WALLASOL FROM ACTI ITEM=TIME WALL VALUE= 17.8150000
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:48:54 JAN 08, 2025 CP= 20.875
wbnew--Modal Campbell (C5)
***** MAPDL RESULTS INTERPRETATION (POST1) *****
*** NOTE *** CP = 20.875 TIME= 17:48:54
The model contains an element type ( COMBI214 ) that operates entirely
in the nodal coordinate system. Viewing nodal displacements or forces
in other than the nodal coordinate system may be invalid. See the
elements manual description for more information.
*** NOTE *** CP = 20.891 TIME= 17:48:54
Reading results into the database (SET command) will update the current
displacement and force boundary conditions in the database with the
values from the results file for that load set. Note that any
subsequent solutions will use these values unless action is taken to
either SAVE the current values or not overwrite them (/EXIT,NOSAVE).
PRINT CAMPBELL DIAGRAM
Sorting : ON
X axis unit : rd/s
Driving component : CM_ROTVELCITY132
Printout all frequencies
*** WARNING *** CP = 21.391 TIME= 17:48:54
Sorting process may not be successful due to the shape of some modes.
If results are not satisfactory, try to change the load steps and/or
the number of modes.
***** FREQUENCIES (Hz) FROM CAMPBELL (sorting on) *****
** The driving component is CM_ROTVELCITY132 **
Spin(rd/s) 0.000 5235.988 10471.976
1 BW 189.190 168.311 144.173
2 FW 207.988 231.858 264.176
3 BW 639.061 628.551 605.754
4 BW 658.854 656.051 653.140
5 FW 735.398 754.878 799.095
6 FW 810.649 812.959 819.766
7 FW 991.015 991.015 991.015
8 BW 1776.768 1759.598 1742.346
9 FW 1777.727 1795.331 1813.903
PRINT CAMPBELL DIAGRAM
Sorting : ON
X axis unit : rd/s
Driving component : CM_ROTVELCITY132
Stability value : ON
Printout all frequencies
*** WARNING *** CP = 21.641 TIME= 17:48:54
Sorting process may not be successful due to the shape of some modes.
If results are not satisfactory, try to change the load steps and/or
the number of modes.
***** STABILITY VALUES (Hz) FROM CAMPBELL (sorting on) *****
** The driving component is CM_ROTVELCITY132 **
Spin(rd/s) 0.000 5235.988 10471.976
1 BW 0.000 0.000 0.000
2 FW 0.000 0.000 0.000
3 BW 0.000 0.000 0.000
4 BW 0.000 0.000 0.000
5 FW 0.000 0.000 0.000
6 FW 0.000 0.000 0.000
7 FW 0.000 0.000 0.000
8 BW 0.000 0.000 0.000
9 FW 0.000 0.000 0.000
PRINTOUT RESUMED BY /GOP
PRINTOUT RESUMED BY /GOP
Set Encoding of XML File to:ISO-8859-1
Set Output of XML File to:
PARM, , , , , , , , , , , ,
, , , , , , ,
DATABASE WRITTEN ON FILE parm.xml
EXIT THE MAPDL POST1 DATABASE PROCESSOR
***** ROUTINE COMPLETED ***** CP = 21.703
PRINTOUT RESUMED BY /GOP
*GET _WALLDONE FROM ACTI ITEM=TIME WALL VALUE= 17.8152778
PARAMETER _PREPTIME = 0.000000000
PARAMETER _SOLVTIME = 20.00000000
PARAMETER _POSTTIME = 1.000000000
PARAMETER _TOTALTIM = 21.00000000
*GET _DLBRATIO FROM ACTI ITEM=SOLU DLBR VALUE= 1.00072046
*GET _COMBTIME FROM ACTI ITEM=SOLU COMB VALUE= 0.426847700
*GET _SSMODE FROM ACTI ITEM=SOLU SSMM VALUE= 2.00000000
*GET _NDOFS FROM ACTI ITEM=SOLU NDOF VALUE= 52628.0000
/FCLEAN COMMAND REMOVING ALL LOCAL FILES
--- Total number of nodes = 17554
--- Total number of elements = 11111
--- Element load balance ratio = 1.00072046
--- Time to combine distributed files = 0.4268477
--- Sparse memory mode = 2
--- Number of DOF = 52628
EXIT MAPDL WITHOUT SAVING DATABASE
NUMBER OF WARNING MESSAGES ENCOUNTERED= 15
NUMBER OF ERROR MESSAGES ENCOUNTERED= 0
+--------------------- M A P D L S T A T I S T I C S ------------------------+
Release: 2024 R2 Build: 24.2 Update: UP20240603 Platform: WINDOWS x64
Date Run: 01/08/2025 Time: 17:48 Process ID: 21372
Operating System: Windows 11 (Build: 22631)
Processor Model: Intel(R) Xeon(R) Platinum 8171M CPU @ 2.60GHz
Compiler: Intel(R) Fortran Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) C/C++ Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) oneAPI Math Kernel Library Version 2023.1-Product Build 20230303
Number of machines requested : 1
Total number of cores available : 8
Number of physical cores available : 4
Number of processes requested : 4
Number of threads per process requested : 1
Total number of cores requested : 4 (Distributed Memory Parallel)
MPI Type: INTELMPI
MPI Version: Intel(R) MPI Library 2021.11 for Windows* OS
GPU Acceleration: Not Requested
Job Name: file0
Input File: dummy.dat
Core Machine Name Working Directory
-----------------------------------------------------
0 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3EE6
1 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3EE6
2 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3EE6
3 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr3EE6
Latency time from master to core 1 = 3.032 microseconds
Latency time from master to core 2 = 3.383 microseconds
Latency time from master to core 3 = 4.196 microseconds
Communication speed from master to core 1 = 4738.21 MB/sec
Communication speed from master to core 2 = 5034.68 MB/sec
Communication speed from master to core 3 = 5373.49 MB/sec
Total CPU time for main thread : 18.4 seconds
Total CPU time summed for all threads : 21.8 seconds
Elapsed time spent obtaining a license : 0.5 seconds
Elapsed time spent pre-processing model (/PREP7) : 0.2 seconds
Elapsed time spent solution - preprocessing : 0.4 seconds
Elapsed time spent computing solution : 18.8 seconds
Elapsed time spent solution - postprocessing : 0.4 seconds
Elapsed time spent post-processing model (/POST1) : 0.4 seconds
Eigensolver used : QRdamp
Equation solver computational rate : 38.7 Gflops
Equation solver effective I/O rate : 13.0 GB/sec
Sum of disk space used on all processes : 429.4 MB
Sum of memory used on all processes : 791.0 MB
Sum of memory allocated on all processes : 3342.0 MB
Physical memory available : 32 GB
Total amount of I/O written to disk : 0.9 GB
Total amount of I/O read from disk : 13.1 GB
+------------------ E N D M A P D L S T A T I S T I C S -------------------+
*-----------------------------------------------------------------------------*
| |
| RUN COMPLETED |
| |
|-----------------------------------------------------------------------------|
| |
| Ansys MAPDL 2024 R2 Build 24.2 UP20240603 WINDOWS x64 |
| |
|-----------------------------------------------------------------------------|
| |
| Database Requested(-db) 1024 MB Scratch Memory Requested 1024 MB |
| Max Database Used(Master) 13 MB Max Scratch Used(Master) 198 MB |
| Max Database Used(Workers) 1 MB Max Scratch Used(Workers) 196 MB |
| Sum Database Used(All) 16 MB Sum Scratch Used(All) 775 MB |
| |
|-----------------------------------------------------------------------------|
| |
| CP Time (sec) = 21.828 Time = 17:48:56 |
| Elapsed Time (sec) = 24.000 Date = 01/08/2025 |
| |
*-----------------------------------------------------------------------------*
Specify the Mechanical directory path for the Modal Campbell Analysis and fetch the image directory path. Download an image file (tot_deform_3D.png
) from the server to the client’s current working directory and display it using matplotlib
.
[35]:
from matplotlib import image as mpimg
from matplotlib import pyplot as plt
[36]:
mechanical.run_python_script(f"image_dir=ExtAPI.DataModel.AnalysisList[2].WorkingDir")
result_image_dir_server = mechanical.run_python_script(f"image_dir")
print(f"Images are stored on the server at: {result_image_dir_server}")
Images are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-2\MECH\
[37]:
def get_image_path(image_name):
return os.path.join(result_image_dir_server, image_name)
[38]:
def display_image(path):
print(f"Printing {path} using matplotlib")
image1 = mpimg.imread(path)
plt.figure(figsize=(15, 15))
plt.axis("off")
plt.imshow(image1)
plt.show()
[39]:
image_name = "tot_deform_3D.png"
image_path_server = get_image_path(image_name)
[40]:
if image_path_server != "":
current_working_directory = os.getcwd()
local_file_path_list = mechanical.download(
image_path_server, target_dir=current_working_directory
)
image_local_path = local_file_path_list[0]
print(f"Local image path : {image_local_path}")
display_image(image_local_path)
Downloading dns:///127.0.0.1:55013:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-2\MECH\tot_deform_3D.png to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\tot_deform_3D.png: 100%|██████████| 112k/112k [00:00<00:00, 115MB/s]
Local image path : C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\tot_deform_3D.png
Printing C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\tot_deform_3D.png using matplotlib
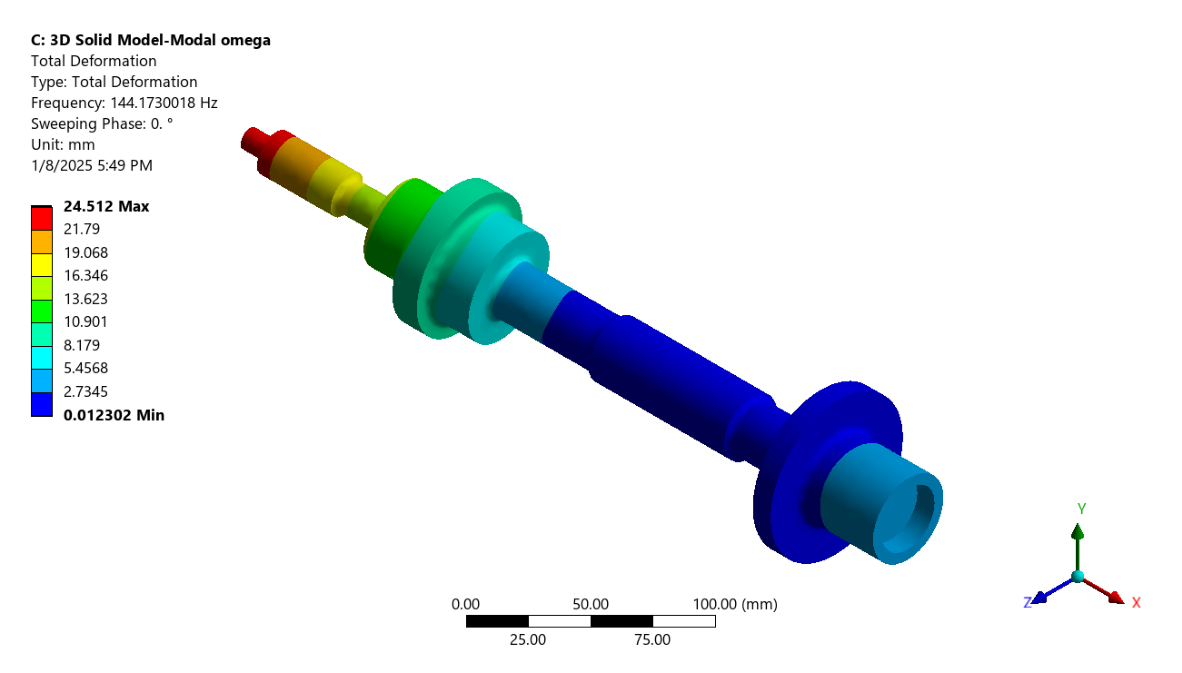
Specify the Mechanical directory for the Unbalance Response Analysis and fetch the working directory path. Download the solver output file (solve.out
) from the server to the client’s current working directory and print its contents.
[41]:
mechanical.run_python_script(f"solve_dir=ExtAPI.DataModel.AnalysisList[3].WorkingDir")
result_solve_dir_server = mechanical.run_python_script(f"solve_dir")
print(f"All solver files are stored on the server at: {result_solve_dir_server}")
All solver files are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-3\MECH\
[42]:
solve_out_path = os.path.join(result_solve_dir_server, "solve.out")
[43]:
def write_file_contents_to_console(path):
"""Write file contents to console."""
with open(path, "rt") as file:
for line in file:
print(line, end="")
[44]:
current_working_directory = os.getcwd()
mechanical.download(solve_out_path, target_dir=current_working_directory)
solve_out_local_path = os.path.join(current_working_directory, "solve.out")
write_file_contents_to_console(solve_out_local_path)
os.remove(solve_out_local_path)
Downloading dns:///127.0.0.1:55013:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-3\MECH\solve.out to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor\solve.out: 100%|██████████| 79.7k/79.7k [00:00<00:00, 82.4MB/s]
Ansys Mechanical Enterprise
*------------------------------------------------------------------*
| |
| W E L C O M E T O T H E A N S Y S (R) P R O G R A M |
| |
*------------------------------------------------------------------*
***************************************************************
* ANSYS MAPDL 2024 R2 LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2024 Ansys, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of Ansys, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the Ansys, Inc. online documentation or the Ansys, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* Ansys, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* Ansys, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the Ansys, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the Ansys, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
2024 R2
Point Releases and Patches installed:
Ansys Service Pack 2024 R2.01
Ansys Service Pack 2024 R2.02
Ansys Service Pack 2024 R2.03
Ansys, Inc. License Manager 2024 R2
Ansys, Inc. License Manager 2024 R2.01
Ansys, Inc. License Manager 2024 R2.02
Ansys, Inc. License Manager 2024 R2.03
Discovery 2024 R2
Discovery 2024 R2.01
Discovery 2024 R2.02
Discovery 2024 R2.03
Core WB Files 2024 R2
Core WB Files 2024 R2.01
Core WB Files 2024 R2.02
Core WB Files 2024 R2.03
SpaceClaim 2024 R2
SpaceClaim 2024 R2.01
SpaceClaim 2024 R2.02
SpaceClaim 2024 R2.03
Icepak (includes CFD-Post) 2024 R2
Icepak (includes CFD-Post) 2024 R2.01
Icepak (includes CFD-Post) 2024 R2.02
Icepak (includes CFD-Post) 2024 R2.03
CFD-Post only 2024 R2
CFD-Post only 2024 R2.01
CFD-Post only 2024 R2.02
CFD-Post only 2024 R2.03
CFX (includes CFD-Post) 2024 R2
CFX (includes CFD-Post) 2024 R2.01
CFX (includes CFD-Post) 2024 R2.02
CFX (includes CFD-Post) 2024 R2.03
Chemkin 2024 R2
Chemkin 2024 R2.01
Chemkin 2024 R2.02
Chemkin 2024 R2.03
EnSight 2024 R2
EnSight 2024 R2.01
EnSight 2024 R2.02
EnSight 2024 R2.03
FENSAP-ICE 2024 R2
FENSAP-ICE 2024 R2.01
FENSAP-ICE 2024 R2.02
FENSAP-ICE 2024 R2.03
Fluent (includes CFD-Post) 2024 R2
Fluent (includes CFD-Post) 2024 R2.01
Fluent (includes CFD-Post) 2024 R2.02
Fluent (includes CFD-Post) 2024 R2.03
Polyflow (includes CFD-Post) 2024 R2
Polyflow (includes CFD-Post) 2024 R2.01
Polyflow (includes CFD-Post) 2024 R2.02
Polyflow (includes CFD-Post) 2024 R2.03
Forte (includes EnSight) 2024 R2
Forte (includes EnSight) 2024 R2.01
Forte (includes EnSight) 2024 R2.02
Forte (includes EnSight) 2024 R2.03
ICEM CFD 2024 R2
ICEM CFD 2024 R2.01
ICEM CFD 2024 R2.02
ICEM CFD 2024 R2.03
TurboGrid 2024 R2
TurboGrid 2024 R2.01
TurboGrid 2024 R2.02
TurboGrid 2024 R2.03
Speos 2024 R2
Speos 2024 R2.01
Speos 2024 R2.02
Speos 2024 R2.03
Speos HPC 2024 R2
Speos HPC 2024 R2.01
Speos HPC 2024 R2.02
Speos HPC 2024 R2.03
optiSLang 2024 R2
optiSLang 2024 R2.01
optiSLang 2024 R2.02
optiSLang 2024 R2.03
Remote Solve Manager Standalone Services 2024 R2
Remote Solve Manager Standalone Services 2024 R2.01
Remote Solve Manager Standalone Services 2024 R2.02
Remote Solve Manager Standalone Services 2024 R2.03
Additive 2024 R2
Additive 2024 R2.01
Additive 2024 R2.02
Additive 2024 R2.03
Aqwa 2024 R2
Aqwa 2024 R2.01
Aqwa 2024 R2.02
Aqwa 2024 R2.03
Autodyn 2024 R2
Autodyn 2024 R2.01
Autodyn 2024 R2.02
Autodyn 2024 R2.03
Customization Files for User Programmable Features 2024 R2
Customization Files for User Programmable Features 2024 R2.01
Customization Files for User Programmable Features 2024 R2.02
Customization Files for User Programmable Features 2024 R2.03
LS-DYNA 2024 R2
LS-DYNA 2024 R2.01
LS-DYNA 2024 R2.02
LS-DYNA 2024 R2.03
Mechanical Products 2024 R2
Mechanical Products 2024 R2.01
Mechanical Products 2024 R2.02
Mechanical Products 2024 R2.03
Motion 2024 R2
Motion 2024 R2.01
Motion 2024 R2.02
Motion 2024 R2.03
Sherlock 2024 R2
Sherlock 2024 R2.01
Sherlock 2024 R2.02
Sherlock 2024 R2.03
Sound - SAS 2024 R2
Sound - SAS 2024 R2.01
Sound - SAS 2024 R2.02
Sound - SAS 2024 R2.03
ACIS Geometry Interface 2024 R2
ACIS Geometry Interface 2024 R2.01
ACIS Geometry Interface 2024 R2.02
ACIS Geometry Interface 2024 R2.03
AutoCAD Geometry Interface 2024 R2
AutoCAD Geometry Interface 2024 R2.01
AutoCAD Geometry Interface 2024 R2.02
AutoCAD Geometry Interface 2024 R2.03
Catia, Version 4 Geometry Interface 2024 R2
Catia, Version 4 Geometry Interface 2024 R2.01
Catia, Version 4 Geometry Interface 2024 R2.02
Catia, Version 4 Geometry Interface 2024 R2.03
Catia, Version 5 Geometry Interface 2024 R2
Catia, Version 5 Geometry Interface 2024 R2.01
Catia, Version 5 Geometry Interface 2024 R2.02
Catia, Version 5 Geometry Interface 2024 R2.03
Catia, Version 6 Geometry Interface 2024 R2
Catia, Version 6 Geometry Interface 2024 R2.01
Catia, Version 6 Geometry Interface 2024 R2.02
Catia, Version 6 Geometry Interface 2024 R2.03
Creo Elements/Direct Modeling Geometry Interface 2024 R2
Creo Elements/Direct Modeling Geometry Interface 2024 R2.01
Creo Elements/Direct Modeling Geometry Interface 2024 R2.02
Creo Elements/Direct Modeling Geometry Interface 2024 R2.03
Creo Parametric Geometry Interface 2024 R2
Creo Parametric Geometry Interface 2024 R2.01
Creo Parametric Geometry Interface 2024 R2.02
Creo Parametric Geometry Interface 2024 R2.03
Inventor Geometry Interface 2024 R2
Inventor Geometry Interface 2024 R2.01
Inventor Geometry Interface 2024 R2.02
Inventor Geometry Interface 2024 R2.03
JTOpen Geometry Interface 2024 R2
JTOpen Geometry Interface 2024 R2.01
JTOpen Geometry Interface 2024 R2.02
JTOpen Geometry Interface 2024 R2.03
NX Geometry Interface 2024 R2
NX Geometry Interface 2024 R2.01
NX Geometry Interface 2024 R2.02
NX Geometry Interface 2024 R2.03
Parasolid Geometry Interface 2024 R2
Parasolid Geometry Interface 2024 R2.01
Parasolid Geometry Interface 2024 R2.02
Parasolid Geometry Interface 2024 R2.03
Solid Edge Geometry Interface 2024 R2
Solid Edge Geometry Interface 2024 R2.01
Solid Edge Geometry Interface 2024 R2.02
Solid Edge Geometry Interface 2024 R2.03
SOLIDWORKS Geometry Interface 2024 R2
SOLIDWORKS Geometry Interface 2024 R2.01
SOLIDWORKS Geometry Interface 2024 R2.02
SOLIDWORKS Geometry Interface 2024 R2.03
***** MAPDL COMMAND LINE ARGUMENTS *****
BATCH MODE REQUESTED (-b) = NOLIST
INPUT FILE COPY MODE (-c) = COPY
DISTRIBUTED MEMORY PARALLEL REQUESTED
4 PARALLEL PROCESSES REQUESTED WITH SINGLE THREAD PER PROCESS
TOTAL OF 4 CORES REQUESTED
INPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr82F5\dummy.dat
OUTPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr82F5\solve.out
START-UP FILE MODE = NOREAD
STOP FILE MODE = NOREAD
RELEASE= 2024 R2 BUILD= 24.2 UP20240603 VERSION=WINDOWS x64
CURRENT JOBNAME=file0 17:49:08 JAN 08, 2025 CP= 0.047
PARAMETER _DS_PROGRESS = 999.0000000
/INPUT FILE= ds.dat LINE= 0
*** NOTE *** CP = 0.359 TIME= 17:49:09
The /CONFIG,NOELDB command is not valid in a distributed memory
parallel solution. Command is ignored.
*GET _WALLSTRT FROM ACTI ITEM=TIME WALL VALUE= 17.8191667
TITLE=
wbnew--Unbalance Response (D5)
ACT Extensions:
LSDYNA, 2024.2
5f463412-bd3e-484b-87e7-cbc0a665e474, wbex
/COM, ANSYSMotion, 2024.2
20180725-3f81-49eb-9f31-41364844c769, wbex
SET PARAMETER DIMENSIONS ON _WB_PROJECTSCRATCH_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_PROJECTSCRATCH_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr82F5\
SET PARAMETER DIMENSIONS ON _WB_SOLVERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_SOLVERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-3\MECH\
SET PARAMETER DIMENSIONS ON _WB_USERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_USERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\user_files\
--- Data in consistent NMM units. See Solving Units in the help system for more
MPA UNITS SPECIFIED FOR INTERNAL
LENGTH = MILLIMETERS (mm)
MASS = TONNE (Mg)
TIME = SECONDS (sec)
TEMPERATURE = CELSIUS (C)
TOFFSET = 273.0
FORCE = NEWTON (N)
HEAT = MILLIJOULES (mJ)
INPUT UNITS ARE ALSO SET TO MPA
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:49:09 JAN 08, 2025 CP= 0.375
wbnew--Unbalance Response (D5)
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
*********** Nodes for the whole assembly ***********
*********** Nodes for all Remote Points ***********
*********** Elements for Body 1 'Solid1' ***********
*********** Elements for Body 2 'Solid4' ***********
*********** Elements for Body 3 'Solid3' ***********
*********** Elements for Body 4 'Solid2' ***********
*********** Send User Defined Coordinate System(s) ***********
*********** Set Reference Temperature ***********
*********** Send Materials ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Element Component ***********
*********** Create Remote Point "PointMass_RemotePoint" ***********
*********** Create Remote Point "RemotePoint_Bearing1" ***********
*********** Create Remote Point "RemotePoint_Bearing2" ***********
*********** Create Remote Point "RemotePoint_FreeStanding1" ***********
*********** Create Remote Point "RemotePoint_FreeStanding2" ***********
*********** Create Remote Point "PointMass_RemotePoint2" ***********
*********** Construct Remote Mass Using Remote Attachment ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*********** Construct Remote Displacement ***********
*** Create a component for all remote displacements ***
*********** Create Bearing Connection "RemotePoint_FreeStanding1 To Multiple" *
Real Constant Set For Above Bearing Connection Is 14
*********** Create Bearing Connection "RemotePoint_FreeStanding2 To Multiple" *
Real Constant Set For Above Bearing Connection Is 15
*********** Component For Rotating Forces ***********
Component For : All Bodies
*********** SYNCHRO & CMOMEGA For Rotating Forces ***********
SYNCHRONOUS NODAL FORCE EXCITATION
*********** Define Rotating Force "Rotating Force" ***********
RotatingForceComponentName,cm_SynchroComponent
RotatingForceLoc,89.0100021362305,0.5,5.29573956636733e-016
UnbalancedMass,3.8e-003
UnbalancedForce,1.9e-003
RotatingRadius,0.5
PhaseAngle,0.
RotatingForceAxisLoc,89.01,0.,0.
RotatingForceAxisComponents,1.,0.,0.
HitPointLocation,89.0100021362305,0.,0.
HitPointNodeId,17545
***** ROUTINE COMPLETED ***** CP = 0.719
--- Number of total nodes = 17554
--- Number of contact elements = 134
--- Number of spring elements = 0
--- Number of bearing elements = 0
--- Number of solid elements = 10970
--- Number of condensed parts = 0
--- Number of total elements = 11111
*GET _WALLBSOL FROM ACTI ITEM=TIME WALL VALUE= 17.8191667
***** MAPDL SOLUTION ROUTINE *****
PERFORM A HARMONIC ANALYSIS
THIS WILL BE A NEW ANALYSIS
PERFORM A FULL HARMONIC RESPONSE ANALYSIS
THERMAL STRAINS ARE NOT INCLUDED IN THE LOAD VECTOR.
STEP BOUNDARY CONDITION KEY= 1
HARMONIC FREQUENCY RANGE - END= 1666.7 BEGIN= 0.0000
USE 200 SUBSTEP(S) THIS LOAD STEP FOR ALL DEGREES OF FREEDOM
STRUCTURAL DAMPING COEFFICIENT = 0.20000E-01
CORIOLIS IN STATIONARY REFERENCE FRAME: GYROSCOPIC DAMPING MATRIX WILL BE CALCULATED
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EANG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE ETMP ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE VENG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE STRS ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPEL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE RSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE CONT ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
PRINTOUT RESUMED BY /GOP
WRITE MISC ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR THE ENTITIES DEFINED BY COMPONENT _ELMISC
*GET ANSINTER_ FROM ACTI ITEM=INT VALUE= 0.00000000
*IF ANSINTER_ ( = 0.00000 ) NE
0 ( = 0.00000 ) THEN
*ENDIF
*** NOTE *** CP = 0.828 TIME= 17:49:09
The automatic domain decomposition logic has selected the FREQ domain
decomposition method with 1 processes per frequency solution.
***** MAPDL SOLVE COMMAND *****
D I S T R I B U T E D D O M A I N D E C O M P O S E R
...Number of frequency solutions: 200
...Decompose to 4 frequency domains (with 1 processes per domain)
*** WARNING *** CP = 1.891 TIME= 17:49:10
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** WARNING *** CP = 1.938 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 1.938 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 1.938 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 1.938 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
HARMONIC EXCITATION IS UNBALANCE
Rotational velocity equals excitation frequency
Rotating component considered = CM_SYNCHROCOMPONENT
*** NOTE *** CP = 1.953 TIME= 17:49:10
The model data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr8
82F5\file0.err ) for these warning messages.
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 3 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 4 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:49:10 JAN 08, 2025 CP= 1.969
wbnew--Unbalance Response (D5)
S O L U T I O N O P T I O N S
PROBLEM DIMENSIONALITY. . . . . . . . . . . . .3-D
DEGREES OF FREEDOM. . . . . . UX UY UZ ROTX ROTY ROTZ
ANALYSIS TYPE . . . . . . . . . . . . . . . . .HARMONIC
SOLUTION METHOD. . . . . . . . . . . . . . .FULL
OFFSET TEMPERATURE FROM ABSOLUTE ZERO . . . . . 273.15
THERMAL EXPANSION . . . . . . . . . . . . . . .OFF
COMPLEX DISPLACEMENT PRINT OPTION . . . . . . .REAL AND IMAGINARY
GLOBALLY ASSEMBLED MATRIX . . . . . . . . . . .UNSYMMETRIC
*** WARNING *** CP = 2.016 TIME= 17:49:10
Material number 14 (used by element 13909) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 2.062 TIME= 17:49:10
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr8
82F5\file0.err ) for these warning messages.
*** NOTE *** CP = 2.125 TIME= 17:49:10
Internal nodes from 17555 to 17558 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
*** NOTE *** CP = 2.250 TIME= 17:49:10
Internal nodes from 17555 to 17558 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
*WARNING*: Some MPC/Lagrange based elements (e.g.13911) in real
constant set 5 overlap with other MPC/Lagrange based elements
(e.g.13998) in real constant set 11 which can cause overconstraint.
*** NOTE *** CP = 2.359 TIME= 17:49:10
Force-distributed-surface identified by real constant set 5 and
contact element type 5 has been set up. The pilot node 17545 is used
to apply the force which connects to other element 14049. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
*WARNING*: Certain contact elements (for example 13960&14047) overlap
each other.
****************************************
*** WARNING *** CP = 2.359 TIME= 17:49:10
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** WARNING *** CP = 2.359 TIME= 17:49:10
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** NOTE *** CP = 2.359 TIME= 17:49:10
Force-distributed-surface identified by real constant set 7 and
contact element type 7 has been set up. The pilot node 17546 is used
to apply the force which connects to other element 13909. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** WARNING *** CP = 2.359 TIME= 17:49:10
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** NOTE *** CP = 2.359 TIME= 17:49:10
Force-distributed-surface identified by real constant set 9 and
contact element type 9 has been set up. The pilot node 17547 is used
to apply the force which connects to other element 13910. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** WARNING *** CP = 2.359 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** NOTE *** CP = 2.359 TIME= 17:49:10
Force-distributed-surface identified by real constant set 11 and
contact element type 11 has been set up. The pilot node 17550 is used
to apply the force. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
*WARNING*: Most likely no MPC equations will be built due to
overlapping with other pairs (i.e. Real constant set 5).
Please verify constraints (including rotational degrees of freedom)
on the pilot node by yourself.
****************************************
*** WARNING *** CP = 2.359 TIME= 17:49:10
Overconstraint may occur for Lagrange multiplier or MPC based contact
algorithm.
The reasons for possible overconstraint are:
*** WARNING *** CP = 2.359 TIME= 17:49:10
Certain contact elements (for example 13960 & 14047) overlap with
other.
*** WARNING *** CP = 2.359 TIME= 17:49:10
Certain contact pairs (for example 11 & 5) overlap with other.
****************************************
*** WARNING *** CP = 2.359 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** NOTE *** CP = 2.422 TIME= 17:49:10
Internal nodes from 17555 to 17558 are created.
4 internal nodes are used for handling degrees of freedom on pilot
nodes of rigid target surfaces.
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 1
FREQUENCY RANGE . . . . . . . . . . . . . . . . 0.0000 TO 1666.7
NUMBER OF SUBSTEPS. . . . . . . . . . . . . . . 200
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . YES
STRUCTURAL DAMPING COEFFICIENT. . . . . . . . . 0.20000E-01
CORIOLIS EFFECT IN STATIONARY REF. FRAME . . . ON
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
RSOL ALL
CONT ALL
MISC ALL _ELMISC
*WARNING*: Some MPC/Lagrange based elements (e.g.13911) in real
constant set 5 overlap with other MPC/Lagrange based elements
(e.g.13998) in real constant set 11 which can cause overconstraint.
*** WARNING *** CP = 3.000 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** NOTE *** CP = 3.000 TIME= 17:49:10
Force-distributed-surface identified by real constant set 5 and
contact element type 5 has been set up. The pilot node 17545 is used
to apply the force which connects to other element 14049. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
*WARNING*: Certain contact elements (for example 13960&14047) overlap
each other.
****************************************
*** WARNING *** CP = 3.000 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 3.000 TIME= 17:49:10
Material number 14 (used by element 13909) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 3.000 TIME= 17:49:10
Force-distributed-surface identified by real constant set 7 and
contact element type 7 has been set up. The pilot node 17546 is used
to apply the force which connects to other element 13909. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** WARNING *** CP = 3.000 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 3.000 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** NOTE *** CP = 3.000 TIME= 17:49:10
Force-distributed-surface identified by real constant set 9 and
contact element type 9 has been set up. The pilot node 17547 is used
to apply the force which connects to other element 13910. Internal
MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
****************************************
*** WARNING *** CP = 3.000 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 3.000 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** NOTE *** CP = 3.000 TIME= 17:49:10
Force-distributed-surface identified by real constant set 11 and
contact element type 11 has been set up. The pilot node 17550 is used
to apply the force. Internal MPC will be built.
The used degrees of freedom set is UX UY UZ ROTX ROTY ROTZ
*WARNING*: Most likely no MPC equations will be built due to
overlapping with other pairs (i.e. Real constant set 5).
Please verify constraints (including rotational degrees of freedom)
on the pilot node by yourself.
****************************************
*** WARNING *** CP = 3.000 TIME= 17:49:10
Material number 14 (used by element 13909) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** WARNING *** CP = 3.000 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 3.047 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 3.047 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 3.109 TIME= 17:49:10
Make sure that the rotating element component 1 (component name
CM_SYNCHROCOMPONENT) is axisymmetric about the axis of spin defined by
the CMOMEGA command to correctly account for the gyroscopic effect in
the stationary reference frame (CORIOLIS, ON,,, ON).
*** WARNING *** CP = 3.109 TIME= 17:49:10
Material number 14 (used by element 13909) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** WARNING *** CP = 3.109 TIME= 17:49:10
Overconstraint may occur for Lagrange multiplier or MPC based contact
algorithm.
*** WARNING *** CP = 3.109 TIME= 17:49:10
Certain contact elements (for example 13960 & 14047) overlap with
other.
*** WARNING *** CP = 3.109 TIME= 17:49:10
Certain contact pairs (for example 11 & 5) overlap with other.
*** WARNING *** CP = 3.109 TIME= 17:49:10
Overconstraint may occur for Lagrange multiplier or MPC based contact
algorithm.
*** WARNING *** CP = 3.109 TIME= 17:49:10
Certain contact elements (for example 13960 & 14047) overlap with
other.
*** WARNING *** CP = 3.109 TIME= 17:49:10
Certain contact pairs (for example 11 & 5) overlap with other.
*** WARNING *** CP = 3.297 TIME= 17:49:10
Overconstraint may occur for Lagrange multiplier or MPC based contact
algorithm.
*** WARNING *** CP = 3.297 TIME= 17:49:10
Certain contact elements (for example 13960 & 14047) overlap with
other.
*** WARNING *** CP = 3.562 TIME= 17:49:10
Certain contact pairs (for example 11 & 5) overlap with other.
*********** PRECISE MASS SUMMARY ***********
TOTAL RIGID BODY MASS MATRIX ABOUT ORIGIN
Translational mass | Coupled translational/rotational mass
0.38541E-02 0.0000 0.0000 | 0.0000 -0.31030E-07 0.13548E-06
0.0000 0.38541E-02 0.0000 | 0.31030E-07 0.0000 0.62248
0.0000 0.0000 0.38541E-02 | -0.13548E-06 -0.62248 0.0000
------------------------------------------ | ------------------------------------------
| Rotational mass (inertia)
| 2.7505 0.15255E-04 0.91712E-05
| 0.15255E-04 149.58 -0.22072E-05
| 0.91712E-05 -0.22072E-05 149.58
TOTAL MASS = 0.38541E-02
The mass principal axes coincide with the global Cartesian axes
CENTER OF MASS (X,Y,Z)= 161.51 -0.35152E-04 -0.80514E-05
TOTAL INERTIA ABOUT CENTER OF MASS
2.7505 -0.66264E-05 0.41594E-05
-0.66264E-05 49.038 -0.22072E-05
0.41594E-05 -0.22072E-05 49.038
The inertia principal axes coincide with the global Cartesian axes
*** MASS SUMMARY BY ELEMENT TYPE ***
TYPE MASS
1 0.788597E-03
2 0.274317E-03
3 0.768710E-03
4 0.621430E-03
13 0.140100E-02
Range of element maximum matrix coefficients in global coordinates
Maximum = 8468651.17 at element 4255.
Minimum = 35030 at element 13910.
*** ELEMENT MATRIX FORMULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 3498 SOLID187 0.438 0.000125
2 1408 SOLID187 0.141 0.000100
3 3842 SOLID187 0.531 0.000138
4 2222 SOLID187 0.219 0.000098
5 50 CONTA174 0.000 0.000000
6 1 TARGE170 0.000 0.000000
7 17 CONTA174 0.000 0.000000
8 1 TARGE170 0.000 0.000000
9 17 CONTA174 0.000 0.000000
10 1 TARGE170 0.000 0.000000
11 50 CONTA174 0.000 0.000000
12 1 TARGE170 0.000 0.000000
13 1 MASS21 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 COMBI214 0.000 0.000000
Time at end of element matrix formulation CP = 4.875.
*** GYROSCOPIC DAMPING MATRIX CALCULATED FOR LISTED ELEMENTS:
TYPE NUMBER ENAME
1 3498 SOLID187
2 1408 SOLID187
3 3842 SOLID187
4 2222 SOLID187
13 1 MASS21
SPARSE MATRIX DIRECT SOLVER.
Number of equations = 52628, Maximum wavefront = 1062
*** NOTE *** CP = 6.391 TIME= 17:49:14
The initial memory allocation (-m) has been exceeded.
Supplemental memory allocations are being used.
Memory allocated on this process
-------------------------------------------------------------------
Equation solver memory allocated = 951.501 MB
Equation solver memory required for in-core mode = 911.084 MB
Equation solver memory required for out-of-core mode = 296.625 MB
Total (solver and non-solver) memory allocated = 1967.087 MB
*** NOTE *** CP = 6.391 TIME= 17:49:14
The Sparse Matrix Solver is currently running in the in-core memory
mode. This memory mode uses the most amount of memory in order to
avoid using the hard drive as much as possible, which most often
results in the fastest solution time. This mode is recommended if
enough physical memory is present to accommodate all of the solver
data.
*** NOTE *** CP = 6.391 TIME= 17:49:14
The system matrix is unsymmetric.
Sparse solver maximum pivot= 8675105.35 at node 4349 UX.
Sparse solver minimum pivot= 11011.7424 at node 209 UY.
Sparse solver minimum pivot in absolute value= 11011.7424 at node 209
UY.
*** ELEMENT RESULT CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 3498 SOLID187 0.422 0.000121
2 1408 SOLID187 0.219 0.000155
3 3842 SOLID187 0.516 0.000134
4 2222 SOLID187 0.219 0.000098
5 50 CONTA174 0.000 0.000000
7 17 CONTA174 0.000 0.000000
9 17 CONTA174 0.000 0.000000
11 50 CONTA174 0.000 0.000000
13 1 MASS21 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 COMBI214 0.000 0.000000
*** NODAL LOAD CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 3498 SOLID187 0.156 0.000045
2 1408 SOLID187 0.047 0.000033
3 3842 SOLID187 0.094 0.000024
4 2222 SOLID187 0.047 0.000021
5 50 CONTA174 0.000 0.000000
7 17 CONTA174 0.000 0.000000
9 17 CONTA174 0.000 0.000000
11 50 CONTA174 0.000 0.000000
13 1 MASS21 0.000 0.000000
14 1 COMBI214 0.000 0.000000
15 1 COMBI214 0.000 0.000000
*** LOAD STEP 1 SUBSTEP 1 COMPLETED. FREQUENCY= 8.33350
--- CMOMEGA for CM_SYNCH= 52.361 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 2 COMPLETED. FREQUENCY= 16.6670
--- CMOMEGA for CM_SYNCH= 104.72 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 3 COMPLETED. FREQUENCY= 25.0005
--- CMOMEGA for CM_SYNCH= 157.08 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 4 COMPLETED. FREQUENCY= 33.3340
--- CMOMEGA for CM_SYNCH= 209.44 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 5 COMPLETED. FREQUENCY= 41.6675
--- CMOMEGA for CM_SYNCH= 261.80 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 6 COMPLETED. FREQUENCY= 50.0010
--- CMOMEGA for CM_SYNCH= 314.17 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 7 COMPLETED. FREQUENCY= 58.3345
--- CMOMEGA for CM_SYNCH= 366.53 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 8 COMPLETED. FREQUENCY= 66.6680
--- CMOMEGA for CM_SYNCH= 418.89 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 9 COMPLETED. FREQUENCY= 75.0015
--- CMOMEGA for CM_SYNCH= 471.25 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 10 COMPLETED. FREQUENCY= 83.3350
--- CMOMEGA for CM_SYNCH= 523.61 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 11 COMPLETED. FREQUENCY= 91.6685
--- CMOMEGA for CM_SYNCH= 575.97 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 12 COMPLETED. FREQUENCY= 100.002
--- CMOMEGA for CM_SYNCH= 628.33 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 13 COMPLETED. FREQUENCY= 108.336
--- CMOMEGA for CM_SYNCH= 680.69 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 14 COMPLETED. FREQUENCY= 116.669
--- CMOMEGA for CM_SYNCH= 733.05 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 15 COMPLETED. FREQUENCY= 125.002
--- CMOMEGA for CM_SYNCH= 785.41 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 16 COMPLETED. FREQUENCY= 133.336
--- CMOMEGA for CM_SYNCH= 837.77 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 17 COMPLETED. FREQUENCY= 141.670
--- CMOMEGA for CM_SYNCH= 890.14 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 18 COMPLETED. FREQUENCY= 150.003
--- CMOMEGA for CM_SYNCH= 942.50 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 19 COMPLETED. FREQUENCY= 158.337
--- CMOMEGA for CM_SYNCH= 994.86 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 20 COMPLETED. FREQUENCY= 166.670
--- CMOMEGA for CM_SYNCH= 1047.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 21 COMPLETED. FREQUENCY= 175.004
--- CMOMEGA for CM_SYNCH= 1099.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 22 COMPLETED. FREQUENCY= 183.337
--- CMOMEGA for CM_SYNCH= 1151.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 23 COMPLETED. FREQUENCY= 191.671
--- CMOMEGA for CM_SYNCH= 1204.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 24 COMPLETED. FREQUENCY= 200.004
--- CMOMEGA for CM_SYNCH= 1256.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 25 COMPLETED. FREQUENCY= 208.338
--- CMOMEGA for CM_SYNCH= 1309.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 26 COMPLETED. FREQUENCY= 216.671
--- CMOMEGA for CM_SYNCH= 1361.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 27 COMPLETED. FREQUENCY= 225.005
--- CMOMEGA for CM_SYNCH= 1413.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 28 COMPLETED. FREQUENCY= 233.338
--- CMOMEGA for CM_SYNCH= 1466.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 29 COMPLETED. FREQUENCY= 241.671
--- CMOMEGA for CM_SYNCH= 1518.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 30 COMPLETED. FREQUENCY= 250.005
--- CMOMEGA for CM_SYNCH= 1570.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 31 COMPLETED. FREQUENCY= 258.339
--- CMOMEGA for CM_SYNCH= 1623.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 32 COMPLETED. FREQUENCY= 266.672
--- CMOMEGA for CM_SYNCH= 1675.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 33 COMPLETED. FREQUENCY= 275.006
--- CMOMEGA for CM_SYNCH= 1727.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 34 COMPLETED. FREQUENCY= 283.339
--- CMOMEGA for CM_SYNCH= 1780.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 35 COMPLETED. FREQUENCY= 291.673
--- CMOMEGA for CM_SYNCH= 1832.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 36 COMPLETED. FREQUENCY= 300.006
--- CMOMEGA for CM_SYNCH= 1885.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 37 COMPLETED. FREQUENCY= 308.339
--- CMOMEGA for CM_SYNCH= 1937.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 38 COMPLETED. FREQUENCY= 316.673
--- CMOMEGA for CM_SYNCH= 1989.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 39 COMPLETED. FREQUENCY= 325.007
--- CMOMEGA for CM_SYNCH= 2042.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 40 COMPLETED. FREQUENCY= 333.340
--- CMOMEGA for CM_SYNCH= 2094.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 41 COMPLETED. FREQUENCY= 341.674
--- CMOMEGA for CM_SYNCH= 2146.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 42 COMPLETED. FREQUENCY= 350.007
--- CMOMEGA for CM_SYNCH= 2199.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 43 COMPLETED. FREQUENCY= 358.341
--- CMOMEGA for CM_SYNCH= 2251.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 44 COMPLETED. FREQUENCY= 366.674
--- CMOMEGA for CM_SYNCH= 2303.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 45 COMPLETED. FREQUENCY= 375.007
--- CMOMEGA for CM_SYNCH= 2356.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 46 COMPLETED. FREQUENCY= 383.341
--- CMOMEGA for CM_SYNCH= 2408.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 47 COMPLETED. FREQUENCY= 391.675
--- CMOMEGA for CM_SYNCH= 2461.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 48 COMPLETED. FREQUENCY= 400.008
--- CMOMEGA for CM_SYNCH= 2513.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 49 COMPLETED. FREQUENCY= 408.341
--- CMOMEGA for CM_SYNCH= 2565.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 50 COMPLETED. FREQUENCY= 416.675
--- CMOMEGA for CM_SYNCH= 2618.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 51 COMPLETED. FREQUENCY= 425.009
--- CMOMEGA for CM_SYNCH= 2670.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 52 COMPLETED. FREQUENCY= 433.342
--- CMOMEGA for CM_SYNCH= 2722.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 53 COMPLETED. FREQUENCY= 441.676
--- CMOMEGA for CM_SYNCH= 2775.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 54 COMPLETED. FREQUENCY= 450.009
--- CMOMEGA for CM_SYNCH= 2827.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 55 COMPLETED. FREQUENCY= 458.343
--- CMOMEGA for CM_SYNCH= 2879.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 56 COMPLETED. FREQUENCY= 466.676
--- CMOMEGA for CM_SYNCH= 2932.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 57 COMPLETED. FREQUENCY= 475.010
--- CMOMEGA for CM_SYNCH= 2984.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 58 COMPLETED. FREQUENCY= 483.343
--- CMOMEGA for CM_SYNCH= 3036.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 59 COMPLETED. FREQUENCY= 491.676
--- CMOMEGA for CM_SYNCH= 3089.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 60 COMPLETED. FREQUENCY= 500.010
--- CMOMEGA for CM_SYNCH= 3141.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 61 COMPLETED. FREQUENCY= 508.344
--- CMOMEGA for CM_SYNCH= 3194.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 62 COMPLETED. FREQUENCY= 516.677
--- CMOMEGA for CM_SYNCH= 3246.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 63 COMPLETED. FREQUENCY= 525.010
--- CMOMEGA for CM_SYNCH= 3298.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 64 COMPLETED. FREQUENCY= 533.344
--- CMOMEGA for CM_SYNCH= 3351.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 65 COMPLETED. FREQUENCY= 541.678
--- CMOMEGA for CM_SYNCH= 3403.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 66 COMPLETED. FREQUENCY= 550.011
--- CMOMEGA for CM_SYNCH= 3455.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 67 COMPLETED. FREQUENCY= 558.345
--- CMOMEGA for CM_SYNCH= 3508.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 68 COMPLETED. FREQUENCY= 566.678
--- CMOMEGA for CM_SYNCH= 3560.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 69 COMPLETED. FREQUENCY= 575.012
--- CMOMEGA for CM_SYNCH= 3612.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 70 COMPLETED. FREQUENCY= 583.345
--- CMOMEGA for CM_SYNCH= 3665.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 71 COMPLETED. FREQUENCY= 591.678
--- CMOMEGA for CM_SYNCH= 3717.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 72 COMPLETED. FREQUENCY= 600.012
--- CMOMEGA for CM_SYNCH= 3770.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 73 COMPLETED. FREQUENCY= 608.346
--- CMOMEGA for CM_SYNCH= 3822.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 74 COMPLETED. FREQUENCY= 616.679
--- CMOMEGA for CM_SYNCH= 3874.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 75 COMPLETED. FREQUENCY= 625.013
--- CMOMEGA for CM_SYNCH= 3927.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 76 COMPLETED. FREQUENCY= 633.346
--- CMOMEGA for CM_SYNCH= 3979.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 77 COMPLETED. FREQUENCY= 641.680
--- CMOMEGA for CM_SYNCH= 4031.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 78 COMPLETED. FREQUENCY= 650.013
--- CMOMEGA for CM_SYNCH= 4084.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 79 COMPLETED. FREQUENCY= 658.346
--- CMOMEGA for CM_SYNCH= 4136.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 80 COMPLETED. FREQUENCY= 666.680
--- CMOMEGA for CM_SYNCH= 4188.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 81 COMPLETED. FREQUENCY= 675.014
--- CMOMEGA for CM_SYNCH= 4241.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 82 COMPLETED. FREQUENCY= 683.347
--- CMOMEGA for CM_SYNCH= 4293.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 83 COMPLETED. FREQUENCY= 691.681
--- CMOMEGA for CM_SYNCH= 4346.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 84 COMPLETED. FREQUENCY= 700.014
--- CMOMEGA for CM_SYNCH= 4398.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 85 COMPLETED. FREQUENCY= 708.347
--- CMOMEGA for CM_SYNCH= 4450.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 86 COMPLETED. FREQUENCY= 716.681
--- CMOMEGA for CM_SYNCH= 4503.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 87 COMPLETED. FREQUENCY= 725.014
--- CMOMEGA for CM_SYNCH= 4555.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 88 COMPLETED. FREQUENCY= 733.348
--- CMOMEGA for CM_SYNCH= 4607.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 89 COMPLETED. FREQUENCY= 741.682
--- CMOMEGA for CM_SYNCH= 4660.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 90 COMPLETED. FREQUENCY= 750.015
--- CMOMEGA for CM_SYNCH= 4712.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 91 COMPLETED. FREQUENCY= 758.349
--- CMOMEGA for CM_SYNCH= 4764.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 92 COMPLETED. FREQUENCY= 766.682
--- CMOMEGA for CM_SYNCH= 4817.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 93 COMPLETED. FREQUENCY= 775.016
--- CMOMEGA for CM_SYNCH= 4869.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 94 COMPLETED. FREQUENCY= 783.349
--- CMOMEGA for CM_SYNCH= 4921.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 95 COMPLETED. FREQUENCY= 791.683
--- CMOMEGA for CM_SYNCH= 4974.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 96 COMPLETED. FREQUENCY= 800.016
--- CMOMEGA for CM_SYNCH= 5026.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 97 COMPLETED. FREQUENCY= 808.350
--- CMOMEGA for CM_SYNCH= 5079.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 98 COMPLETED. FREQUENCY= 816.683
--- CMOMEGA for CM_SYNCH= 5131.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 99 COMPLETED. FREQUENCY= 825.017
--- CMOMEGA for CM_SYNCH= 5183.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 100 COMPLETED. FREQUENCY= 833.350
--- CMOMEGA for CM_SYNCH= 5236.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 101 COMPLETED. FREQUENCY= 841.683
--- CMOMEGA for CM_SYNCH= 5288.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 102 COMPLETED. FREQUENCY= 850.017
--- CMOMEGA for CM_SYNCH= 5340.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 103 COMPLETED. FREQUENCY= 858.351
--- CMOMEGA for CM_SYNCH= 5393.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 104 COMPLETED. FREQUENCY= 866.684
--- CMOMEGA for CM_SYNCH= 5445.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 105 COMPLETED. FREQUENCY= 875.018
--- CMOMEGA for CM_SYNCH= 5497.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 106 COMPLETED. FREQUENCY= 883.351
--- CMOMEGA for CM_SYNCH= 5550.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 107 COMPLETED. FREQUENCY= 891.685
--- CMOMEGA for CM_SYNCH= 5602.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 108 COMPLETED. FREQUENCY= 900.018
--- CMOMEGA for CM_SYNCH= 5655.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 109 COMPLETED. FREQUENCY= 908.352
--- CMOMEGA for CM_SYNCH= 5707.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 110 COMPLETED. FREQUENCY= 916.685
--- CMOMEGA for CM_SYNCH= 5759.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 111 COMPLETED. FREQUENCY= 925.019
--- CMOMEGA for CM_SYNCH= 5812.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 112 COMPLETED. FREQUENCY= 933.352
--- CMOMEGA for CM_SYNCH= 5864.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 113 COMPLETED. FREQUENCY= 941.686
--- CMOMEGA for CM_SYNCH= 5916.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 114 COMPLETED. FREQUENCY= 950.019
--- CMOMEGA for CM_SYNCH= 5969.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 115 COMPLETED. FREQUENCY= 958.353
--- CMOMEGA for CM_SYNCH= 6021.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 116 COMPLETED. FREQUENCY= 966.686
--- CMOMEGA for CM_SYNCH= 6073.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 117 COMPLETED. FREQUENCY= 975.019
--- CMOMEGA for CM_SYNCH= 6126.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 118 COMPLETED. FREQUENCY= 983.353
--- CMOMEGA for CM_SYNCH= 6178.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 119 COMPLETED. FREQUENCY= 991.687
--- CMOMEGA for CM_SYNCH= 6231.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 120 COMPLETED. FREQUENCY= 1000.02
--- CMOMEGA for CM_SYNCH= 6283.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 121 COMPLETED. FREQUENCY= 1008.35
--- CMOMEGA for CM_SYNCH= 6335.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 122 COMPLETED. FREQUENCY= 1016.69
--- CMOMEGA for CM_SYNCH= 6388.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 123 COMPLETED. FREQUENCY= 1025.02
--- CMOMEGA for CM_SYNCH= 6440.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 124 COMPLETED. FREQUENCY= 1033.35
--- CMOMEGA for CM_SYNCH= 6492.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 125 COMPLETED. FREQUENCY= 1041.69
--- CMOMEGA for CM_SYNCH= 6545.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 126 COMPLETED. FREQUENCY= 1050.02
--- CMOMEGA for CM_SYNCH= 6597.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 127 COMPLETED. FREQUENCY= 1058.35
--- CMOMEGA for CM_SYNCH= 6649.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 128 COMPLETED. FREQUENCY= 1066.69
--- CMOMEGA for CM_SYNCH= 6702.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 129 COMPLETED. FREQUENCY= 1075.02
--- CMOMEGA for CM_SYNCH= 6754.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 130 COMPLETED. FREQUENCY= 1083.36
--- CMOMEGA for CM_SYNCH= 6806.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 131 COMPLETED. FREQUENCY= 1091.69
--- CMOMEGA for CM_SYNCH= 6859.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 132 COMPLETED. FREQUENCY= 1100.02
--- CMOMEGA for CM_SYNCH= 6911.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 133 COMPLETED. FREQUENCY= 1108.36
--- CMOMEGA for CM_SYNCH= 6964.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 134 COMPLETED. FREQUENCY= 1116.69
--- CMOMEGA for CM_SYNCH= 7016.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 135 COMPLETED. FREQUENCY= 1125.02
--- CMOMEGA for CM_SYNCH= 7068.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 136 COMPLETED. FREQUENCY= 1133.36
--- CMOMEGA for CM_SYNCH= 7121.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 137 COMPLETED. FREQUENCY= 1141.69
--- CMOMEGA for CM_SYNCH= 7173.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 138 COMPLETED. FREQUENCY= 1150.02
--- CMOMEGA for CM_SYNCH= 7225.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 139 COMPLETED. FREQUENCY= 1158.36
--- CMOMEGA for CM_SYNCH= 7278.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 140 COMPLETED. FREQUENCY= 1166.69
--- CMOMEGA for CM_SYNCH= 7330.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 141 COMPLETED. FREQUENCY= 1175.02
--- CMOMEGA for CM_SYNCH= 7382.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 142 COMPLETED. FREQUENCY= 1183.36
--- CMOMEGA for CM_SYNCH= 7435.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 143 COMPLETED. FREQUENCY= 1191.69
--- CMOMEGA for CM_SYNCH= 7487.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 144 COMPLETED. FREQUENCY= 1200.02
--- CMOMEGA for CM_SYNCH= 7540.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 145 COMPLETED. FREQUENCY= 1208.36
--- CMOMEGA for CM_SYNCH= 7592.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 146 COMPLETED. FREQUENCY= 1216.69
--- CMOMEGA for CM_SYNCH= 7644.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 147 COMPLETED. FREQUENCY= 1225.02
--- CMOMEGA for CM_SYNCH= 7697.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 148 COMPLETED. FREQUENCY= 1233.36
--- CMOMEGA for CM_SYNCH= 7749.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 149 COMPLETED. FREQUENCY= 1241.69
--- CMOMEGA for CM_SYNCH= 7801.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 150 COMPLETED. FREQUENCY= 1250.03
--- CMOMEGA for CM_SYNCH= 7854.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 151 COMPLETED. FREQUENCY= 1258.36
--- CMOMEGA for CM_SYNCH= 7906.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 152 COMPLETED. FREQUENCY= 1266.69
--- CMOMEGA for CM_SYNCH= 7958.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 153 COMPLETED. FREQUENCY= 1275.03
--- CMOMEGA for CM_SYNCH= 8011.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 154 COMPLETED. FREQUENCY= 1283.36
--- CMOMEGA for CM_SYNCH= 8063.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 155 COMPLETED. FREQUENCY= 1291.69
--- CMOMEGA for CM_SYNCH= 8115.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 156 COMPLETED. FREQUENCY= 1300.03
--- CMOMEGA for CM_SYNCH= 8168.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 157 COMPLETED. FREQUENCY= 1308.36
--- CMOMEGA for CM_SYNCH= 8220.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 158 COMPLETED. FREQUENCY= 1316.69
--- CMOMEGA for CM_SYNCH= 8273.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 159 COMPLETED. FREQUENCY= 1325.03
--- CMOMEGA for CM_SYNCH= 8325.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 160 COMPLETED. FREQUENCY= 1333.36
--- CMOMEGA for CM_SYNCH= 8377.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 161 COMPLETED. FREQUENCY= 1341.69
--- CMOMEGA for CM_SYNCH= 8430.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 162 COMPLETED. FREQUENCY= 1350.03
--- CMOMEGA for CM_SYNCH= 8482.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 163 COMPLETED. FREQUENCY= 1358.36
--- CMOMEGA for CM_SYNCH= 8534.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 164 COMPLETED. FREQUENCY= 1366.69
--- CMOMEGA for CM_SYNCH= 8587.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 165 COMPLETED. FREQUENCY= 1375.03
--- CMOMEGA for CM_SYNCH= 8639.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 166 COMPLETED. FREQUENCY= 1383.36
--- CMOMEGA for CM_SYNCH= 8691.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 167 COMPLETED. FREQUENCY= 1391.69
--- CMOMEGA for CM_SYNCH= 8744.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 168 COMPLETED. FREQUENCY= 1400.03
--- CMOMEGA for CM_SYNCH= 8796.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 169 COMPLETED. FREQUENCY= 1408.36
--- CMOMEGA for CM_SYNCH= 8849.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 170 COMPLETED. FREQUENCY= 1416.69
--- CMOMEGA for CM_SYNCH= 8901.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 171 COMPLETED. FREQUENCY= 1425.03
--- CMOMEGA for CM_SYNCH= 8953.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 172 COMPLETED. FREQUENCY= 1433.36
--- CMOMEGA for CM_SYNCH= 9006.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 173 COMPLETED. FREQUENCY= 1441.70
--- CMOMEGA for CM_SYNCH= 9058.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 174 COMPLETED. FREQUENCY= 1450.03
--- CMOMEGA for CM_SYNCH= 9110.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 175 COMPLETED. FREQUENCY= 1458.36
--- CMOMEGA for CM_SYNCH= 9163.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 176 COMPLETED. FREQUENCY= 1466.70
--- CMOMEGA for CM_SYNCH= 9215.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 177 COMPLETED. FREQUENCY= 1475.03
--- CMOMEGA for CM_SYNCH= 9267.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 178 COMPLETED. FREQUENCY= 1483.36
--- CMOMEGA for CM_SYNCH= 9320.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 179 COMPLETED. FREQUENCY= 1491.70
--- CMOMEGA for CM_SYNCH= 9372.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 180 COMPLETED. FREQUENCY= 1500.03
--- CMOMEGA for CM_SYNCH= 9425.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 181 COMPLETED. FREQUENCY= 1508.36
--- CMOMEGA for CM_SYNCH= 9477.3 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 182 COMPLETED. FREQUENCY= 1516.70
--- CMOMEGA for CM_SYNCH= 9529.7 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 183 COMPLETED. FREQUENCY= 1525.03
--- CMOMEGA for CM_SYNCH= 9582.0 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 184 COMPLETED. FREQUENCY= 1533.36
--- CMOMEGA for CM_SYNCH= 9634.4 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 185 COMPLETED. FREQUENCY= 1541.70
--- CMOMEGA for CM_SYNCH= 9686.8 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 186 COMPLETED. FREQUENCY= 1550.03
--- CMOMEGA for CM_SYNCH= 9739.1 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 187 COMPLETED. FREQUENCY= 1558.36
--- CMOMEGA for CM_SYNCH= 9791.5 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 188 COMPLETED. FREQUENCY= 1566.70
--- CMOMEGA for CM_SYNCH= 9843.9 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 189 COMPLETED. FREQUENCY= 1575.03
--- CMOMEGA for CM_SYNCH= 9896.2 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 190 COMPLETED. FREQUENCY= 1583.37
--- CMOMEGA for CM_SYNCH= 9948.6 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 191 COMPLETED. FREQUENCY= 1591.70
--- CMOMEGA for CM_SYNCH= 10001. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 192 COMPLETED. FREQUENCY= 1600.03
--- CMOMEGA for CM_SYNCH= 10053. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 193 COMPLETED. FREQUENCY= 1608.37
--- CMOMEGA for CM_SYNCH= 10106. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 194 COMPLETED. FREQUENCY= 1616.70
--- CMOMEGA for CM_SYNCH= 10158. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 195 COMPLETED. FREQUENCY= 1625.03
--- CMOMEGA for CM_SYNCH= 10210. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 196 COMPLETED. FREQUENCY= 1633.37
--- CMOMEGA for CM_SYNCH= 10263. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 197 COMPLETED. FREQUENCY= 1641.70
--- CMOMEGA for CM_SYNCH= 10315. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 198 COMPLETED. FREQUENCY= 1650.03
--- CMOMEGA for CM_SYNCH= 10367. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 199 COMPLETED. FREQUENCY= 1658.37
--- CMOMEGA for CM_SYNCH= 10420. 0.0000 0.0000
*** LOAD STEP 1 SUBSTEP 200 COMPLETED. FREQUENCY= 1666.70
--- CMOMEGA for CM_SYNCH= 10472. 0.0000 0.0000
*** MAPDL BINARY FILE STATISTICS
BUFFER SIZE USED= 16384
0.625 MB WRITTEN ON ELEMENT MATRIX FILE: file0.emat
5.438 MB WRITTEN ON ELEMENT SAVED DATA FILE: file0.esav
84.875 MB WRITTEN ON ASSEMBLED MATRIX FILE: file0.full
420.000 MB WRITTEN ON RESULTS FILE: file0.rst
*************** Write FE CONNECTORS *********
WRITE OUT CONSTRAINT EQUATIONS TO FILE= file.ce
FINISH SOLUTION PROCESSING
***** ROUTINE COMPLETED ***** CP = 457.562
PRINTOUT RESUMED BY /GOP
*GET _WALLASOL FROM ACTI ITEM=TIME WALL VALUE= 17.9663889
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 17:57:59 JAN 08, 2025 CP= 457.562
wbnew--Unbalance Response (D5)
***** MAPDL RESULTS INTERPRETATION (POST1) *****
*** NOTE *** CP = 457.562 TIME= 17:57:59
The model contains an element type ( COMBI214 ) that operates entirely
in the nodal coordinate system. Viewing nodal displacements or forces
in other than the nodal coordinate system may be invalid. See the
elements manual description for more information.
*** NOTE *** CP = 457.562 TIME= 17:57:59
Reading results into the database (SET command) will update the current
displacement and force boundary conditions in the database with the
values from the results file for that load set. Note that any
subsequent solutions will use these values unless action is taken to
either SAVE the current values or not overwrite them (/EXIT,NOSAVE).
Set Encoding of XML File to:ISO-8859-1
Set Output of XML File to:
PARM, , , , , , , , , , , ,
, , , , , , ,
DATABASE WRITTEN ON FILE parm.xml
EXIT THE MAPDL POST1 DATABASE PROCESSOR
***** ROUTINE COMPLETED ***** CP = 457.578
PRINTOUT RESUMED BY /GOP
*GET _WALLDONE FROM ACTI ITEM=TIME WALL VALUE= 17.9663889
PARAMETER _PREPTIME = 0.000000000
PARAMETER _SOLVTIME = 530.0000000
PARAMETER _POSTTIME = 0.000000000
PARAMETER _TOTALTIM = 530.0000000
*GET _DLBRATIO FROM ACTI ITEM=SOLU DLBR VALUE= 0.00000000
*GET _COMBTIME FROM ACTI ITEM=SOLU COMB VALUE= 2.78889360
*GET _SSMODE FROM ACTI ITEM=SOLU SSMM VALUE= 2.00000000
*GET _NDOFS FROM ACTI ITEM=SOLU NDOF VALUE= 52628.0000
/FCLEAN COMMAND REMOVING ALL LOCAL FILES
--- Total number of nodes = 17554
--- Total number of elements = 11111
--- Element load balance ratio = 0
--- Time to combine distributed files = 2.7888936
--- Sparse memory mode = 2
--- Number of DOF = 52628
EXIT MAPDL WITHOUT SAVING DATABASE
NUMBER OF WARNING MESSAGES ENCOUNTERED= 36
NUMBER OF ERROR MESSAGES ENCOUNTERED= 0
*** NOTE *** CP = 458.375 TIME= 17:58:01
The solution for the current simulation was performed using 1 cores.
The parallel performance of this simulation on this hardware indicates
that this simulation would benefit from using additional CPU cores.
Consider using more CPU cores, which may require additional HPC
licenses, when running this simulation again on the same hardware.
+--------------------- M A P D L S T A T I S T I C S ------------------------+
Release: 2024 R2 Build: 24.2 Update: UP20240603 Platform: WINDOWS x64
Date Run: 01/08/2025 Time: 17:58 Process ID: 19508
Operating System: Windows 11 (Build: 22631)
Processor Model: Intel(R) Xeon(R) Platinum 8171M CPU @ 2.60GHz
Compiler: Intel(R) Fortran Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) C/C++ Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) oneAPI Math Kernel Library Version 2023.1-Product Build 20230303
Number of machines requested : 1
Total number of cores available : 8
Number of physical cores available : 4
Number of processes requested : 4
Number of threads per process requested : 1
Total number of cores requested : 4 (Distributed Memory Parallel)
MPI Type: INTELMPI
MPI Version: Intel(R) MPI Library 2021.11 for Windows* OS
GPU Acceleration: Not Requested
Job Name: file0
Input File: dummy.dat
Core Machine Name Working Directory
-----------------------------------------------------
0 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr82F5
1 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr82F5
2 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr82F5
3 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\_ProjectScratch\Scr82F5
Latency time from master to core 1 = 4.961 microseconds
Latency time from master to core 2 = 3.347 microseconds
Latency time from master to core 3 = 3.189 microseconds
Communication speed from master to core 1 = 4067.71 MB/sec
Communication speed from master to core 2 = 4785.18 MB/sec
Communication speed from master to core 3 = 5324.49 MB/sec
Total CPU time for main thread : 455.9 seconds
Total CPU time summed for all threads : 458.4 seconds
Elapsed time spent obtaining a license : 0.5 seconds
Elapsed time spent pre-processing model (/PREP7) : 0.1 seconds
Elapsed time spent solution - preprocessing : 0.5 seconds
Elapsed time spent computing solution : 526.3 seconds
Elapsed time spent solution - postprocessing : 2.8 seconds
Elapsed time spent post-processing model (/POST1) : 0.0 seconds
Equation solver used : Sparse (unsymmetric)
Equation solver computational rate : 6.9 Gflops
Sum of disk space used on all processes : 3316.6 MB
Sum of memory used on all processes : 4489.0 MB
Sum of memory allocated on all processes : 7523.0 MB
Physical memory available : 32 GB
Total amount of I/O written to disk : 3.8 GB
Total amount of I/O read from disk : 0.7 GB
+------------------ E N D M A P D L S T A T I S T I C S -------------------+
*-----------------------------------------------------------------------------*
| |
| RUN COMPLETED |
| |
|-----------------------------------------------------------------------------|
| |
| Ansys MAPDL 2024 R2 Build 24.2 UP20240603 WINDOWS x64 |
| |
|-----------------------------------------------------------------------------|
| |
| Database Requested(-db) 1024 MB Scratch Memory Requested 1024 MB |
| Max Database Used(Master) 13 MB Max Scratch Used(Master) 1110 MB |
| Max Database Used(Workers) 12 MB Max Scratch Used(Workers) 1110 MB |
| Sum Database Used(All) 49 MB Sum Scratch Used(All) 4440 MB |
| |
|-----------------------------------------------------------------------------|
| |
| CP Time (sec) = 458.375 Time = 17:58:01 |
| Elapsed Time (sec) = 534.000 Date = 01/08/2025 |
| |
*-----------------------------------------------------------------------------*
Download all the files from the server to the current working directory for the 3D rotor model. Verify the source path for the directory and copy all files from the server to the client.
[45]:
import shutil
import glob
[46]:
current_working_directory = os.getcwd()
target_dir2 = current_working_directory
print(f"Files to be copied from server path at: {target_dir2}")
print(f"All the solver files are stored on the server at: {result_solve_dir_server}")
Files to be copied from server path at: C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\axisymmetric-rotor
All the solver files are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19944_2\wbnew_files\dp0\SYS-3\MECH\
[47]:
source_dir = result_solve_dir_server
destination_dir = target_dir2
[48]:
for file in glob.glob(source_dir + '/*'):
shutil.copy(file, destination_dir)
Finally, call the exit
method on both the PyMechanical and Workbench clients to gracefully shut down the services.
[49]:
mechanical.exit()
wb.exit()