Cyclic symmetry analysis#
This notebook demonstrates how to use the Workbench client to manage projects on a remote host, run scripts, and handle output files. It covers launching services, uploading files, executing scripts, and visualizing results using PyMechanical.
[1]:
import os
import pathlib
[2]:
from ansys.workbench.core import launch_workbench
from ansys.mechanical.core import connect_to_mechanical
Launch the Workbench service on a remote host machine, specifying the remote host machine name and user login credentials. Define several directories that will be used during the session. workdir
is set to the parent directory of the current file. assets
, scripts
, and cdb
are subdirectories within the working directory. The launch_workbench
function is called to start a Workbench session with specified directory.
[3]:
workdir = pathlib.Path("__file__").parent
[4]:
assets = workdir / "assets"
scripts = workdir / "scripts"
[5]:
wb = launch_workbench(client_workdir=str(workdir.absolute()))
Upload the project files to the server using the upload_file_from_example_repo
method. The file to upload is sector_model.cdb
.
[6]:
wb.upload_file_from_example_repo("cyclic-symmetry-analysis/cdb/sector_model.cdb")
Uploading sector_model.cdb: 100%|██████████| 7.86M/7.86M [00:00<00:00, 87.9MB/s]
Execute a Workbench script (project.wbjn
) to define the project and load the geometry using the run_script_file
method. The set_log_file
method is used to direct the logs to wb_log_file.log
. The name of the system created is stored in sys_name
and printed.
[7]:
export_path = 'wb_log_file.log'
wb.set_log_file(export_path)
sys_name = wb.run_script_file(str((assets / "project.wbjn").absolute()), log_level='info')
print(sys_name)
SYS 1
Start a PyMechanical server for the system using the start_mechanical_server
method. Create a PyMechanical client session connected to this server using connect_to_mechanical
. The project directory is printed to verify the connection.
[8]:
server_port = wb.start_mechanical_server(system_name=sys_name)
[9]:
mechanical = connect_to_mechanical(ip='localhost', port=server_port)
[10]:
print(mechanical.project_directory)
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\
Read and execute the script cyclic_symmetry_analysis.py
via the PyMechanical client using run_python_script
. This script typically contains commands to mesh and solve the model. The output of the script is printed.
[11]:
with open(scripts / "cyclic_symmetry_analysis.py") as sf:
mech_script = sf.read()
mech_output = mechanical.run_python_script(mech_script)
print(mech_output)
{"Total Deformation": "5.202362674608513 [mm]", "Total Deformation 2": "2.182211520602412 [mm]"}
Specify the Mechanical directory and run a script to fetch the working directory path. The path where all solver files are stored on the server is printed. Download the solver output file (solve.out
) from the server to the client’s current working directory and print its contents.
[12]:
mechanical.run_python_script(f"solve_dir=ExtAPI.DataModel.AnalysisList[5].WorkingDir")
result_solve_dir_server = mechanical.run_python_script(f"solve_dir")
print(f"All solver files are stored on the server at: {result_solve_dir_server}")
All solver files are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\
[13]:
solve_out_path = os.path.join(result_solve_dir_server, "solve.out")
[14]:
def write_file_contents_to_console(path):
"""Write file contents to console."""
with open(path, "rt") as file:
for line in file:
print(line, end="")
[15]:
current_working_directory = os.getcwd()
mechanical.download(solve_out_path, target_dir=current_working_directory)
solve_out_local_path = os.path.join(current_working_directory, "solve.out")
write_file_contents_to_console(solve_out_local_path)
os.remove(solve_out_local_path)
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\solve.out to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\solve.out: 100%|██████████| 50.3k/50.3k [00:00<?, ?B/s]
Ansys Mechanical Enterprise
*------------------------------------------------------------------*
| |
| W E L C O M E T O T H E A N S Y S (R) P R O G R A M |
| |
*------------------------------------------------------------------*
***************************************************************
* ANSYS MAPDL 2024 R2 LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2024 Ansys, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of Ansys, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the Ansys, Inc. online documentation or the Ansys, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* Ansys, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* Ansys, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the Ansys, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the Ansys, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
2024 R2
Point Releases and Patches installed:
Ansys Service Pack 2024 R2.01
Ansys Service Pack 2024 R2.02
Ansys Service Pack 2024 R2.03
Ansys, Inc. License Manager 2024 R2
Ansys, Inc. License Manager 2024 R2.01
Ansys, Inc. License Manager 2024 R2.02
Ansys, Inc. License Manager 2024 R2.03
Discovery 2024 R2
Discovery 2024 R2.01
Discovery 2024 R2.02
Discovery 2024 R2.03
Core WB Files 2024 R2
Core WB Files 2024 R2.01
Core WB Files 2024 R2.02
Core WB Files 2024 R2.03
SpaceClaim 2024 R2
SpaceClaim 2024 R2.01
SpaceClaim 2024 R2.02
SpaceClaim 2024 R2.03
Icepak (includes CFD-Post) 2024 R2
Icepak (includes CFD-Post) 2024 R2.01
Icepak (includes CFD-Post) 2024 R2.02
Icepak (includes CFD-Post) 2024 R2.03
CFD-Post only 2024 R2
CFD-Post only 2024 R2.01
CFD-Post only 2024 R2.02
CFD-Post only 2024 R2.03
CFX (includes CFD-Post) 2024 R2
CFX (includes CFD-Post) 2024 R2.01
CFX (includes CFD-Post) 2024 R2.02
CFX (includes CFD-Post) 2024 R2.03
Chemkin 2024 R2
Chemkin 2024 R2.01
Chemkin 2024 R2.02
Chemkin 2024 R2.03
EnSight 2024 R2
EnSight 2024 R2.01
EnSight 2024 R2.02
EnSight 2024 R2.03
FENSAP-ICE 2024 R2
FENSAP-ICE 2024 R2.01
FENSAP-ICE 2024 R2.02
FENSAP-ICE 2024 R2.03
Fluent (includes CFD-Post) 2024 R2
Fluent (includes CFD-Post) 2024 R2.01
Fluent (includes CFD-Post) 2024 R2.02
Fluent (includes CFD-Post) 2024 R2.03
Polyflow (includes CFD-Post) 2024 R2
Polyflow (includes CFD-Post) 2024 R2.01
Polyflow (includes CFD-Post) 2024 R2.02
Polyflow (includes CFD-Post) 2024 R2.03
Forte (includes EnSight) 2024 R2
Forte (includes EnSight) 2024 R2.01
Forte (includes EnSight) 2024 R2.02
Forte (includes EnSight) 2024 R2.03
ICEM CFD 2024 R2
ICEM CFD 2024 R2.01
ICEM CFD 2024 R2.02
ICEM CFD 2024 R2.03
TurboGrid 2024 R2
TurboGrid 2024 R2.01
TurboGrid 2024 R2.02
TurboGrid 2024 R2.03
Speos 2024 R2
Speos 2024 R2.01
Speos 2024 R2.02
Speos 2024 R2.03
Speos HPC 2024 R2
Speos HPC 2024 R2.01
Speos HPC 2024 R2.02
Speos HPC 2024 R2.03
optiSLang 2024 R2
optiSLang 2024 R2.01
optiSLang 2024 R2.02
optiSLang 2024 R2.03
Remote Solve Manager Standalone Services 2024 R2
Remote Solve Manager Standalone Services 2024 R2.01
Remote Solve Manager Standalone Services 2024 R2.02
Remote Solve Manager Standalone Services 2024 R2.03
Additive 2024 R2
Additive 2024 R2.01
Additive 2024 R2.02
Additive 2024 R2.03
Aqwa 2024 R2
Aqwa 2024 R2.01
Aqwa 2024 R2.02
Aqwa 2024 R2.03
Autodyn 2024 R2
Autodyn 2024 R2.01
Autodyn 2024 R2.02
Autodyn 2024 R2.03
Customization Files for User Programmable Features 2024 R2
Customization Files for User Programmable Features 2024 R2.01
Customization Files for User Programmable Features 2024 R2.02
Customization Files for User Programmable Features 2024 R2.03
LS-DYNA 2024 R2
LS-DYNA 2024 R2.01
LS-DYNA 2024 R2.02
LS-DYNA 2024 R2.03
Mechanical Products 2024 R2
Mechanical Products 2024 R2.01
Mechanical Products 2024 R2.02
Mechanical Products 2024 R2.03
Motion 2024 R2
Motion 2024 R2.01
Motion 2024 R2.02
Motion 2024 R2.03
Sherlock 2024 R2
Sherlock 2024 R2.01
Sherlock 2024 R2.02
Sherlock 2024 R2.03
Sound - SAS 2024 R2
Sound - SAS 2024 R2.01
Sound - SAS 2024 R2.02
Sound - SAS 2024 R2.03
ACIS Geometry Interface 2024 R2
ACIS Geometry Interface 2024 R2.01
ACIS Geometry Interface 2024 R2.02
ACIS Geometry Interface 2024 R2.03
AutoCAD Geometry Interface 2024 R2
AutoCAD Geometry Interface 2024 R2.01
AutoCAD Geometry Interface 2024 R2.02
AutoCAD Geometry Interface 2024 R2.03
Catia, Version 4 Geometry Interface 2024 R2
Catia, Version 4 Geometry Interface 2024 R2.01
Catia, Version 4 Geometry Interface 2024 R2.02
Catia, Version 4 Geometry Interface 2024 R2.03
Catia, Version 5 Geometry Interface 2024 R2
Catia, Version 5 Geometry Interface 2024 R2.01
Catia, Version 5 Geometry Interface 2024 R2.02
Catia, Version 5 Geometry Interface 2024 R2.03
Catia, Version 6 Geometry Interface 2024 R2
Catia, Version 6 Geometry Interface 2024 R2.01
Catia, Version 6 Geometry Interface 2024 R2.02
Catia, Version 6 Geometry Interface 2024 R2.03
Creo Elements/Direct Modeling Geometry Interface 2024 R2
Creo Elements/Direct Modeling Geometry Interface 2024 R2.01
Creo Elements/Direct Modeling Geometry Interface 2024 R2.02
Creo Elements/Direct Modeling Geometry Interface 2024 R2.03
Creo Parametric Geometry Interface 2024 R2
Creo Parametric Geometry Interface 2024 R2.01
Creo Parametric Geometry Interface 2024 R2.02
Creo Parametric Geometry Interface 2024 R2.03
Inventor Geometry Interface 2024 R2
Inventor Geometry Interface 2024 R2.01
Inventor Geometry Interface 2024 R2.02
Inventor Geometry Interface 2024 R2.03
JTOpen Geometry Interface 2024 R2
JTOpen Geometry Interface 2024 R2.01
JTOpen Geometry Interface 2024 R2.02
JTOpen Geometry Interface 2024 R2.03
NX Geometry Interface 2024 R2
NX Geometry Interface 2024 R2.01
NX Geometry Interface 2024 R2.02
NX Geometry Interface 2024 R2.03
Parasolid Geometry Interface 2024 R2
Parasolid Geometry Interface 2024 R2.01
Parasolid Geometry Interface 2024 R2.02
Parasolid Geometry Interface 2024 R2.03
Solid Edge Geometry Interface 2024 R2
Solid Edge Geometry Interface 2024 R2.01
Solid Edge Geometry Interface 2024 R2.02
Solid Edge Geometry Interface 2024 R2.03
SOLIDWORKS Geometry Interface 2024 R2
SOLIDWORKS Geometry Interface 2024 R2.01
SOLIDWORKS Geometry Interface 2024 R2.02
SOLIDWORKS Geometry Interface 2024 R2.03
***** MAPDL COMMAND LINE ARGUMENTS *****
BATCH MODE REQUESTED (-b) = NOLIST
INPUT FILE COPY MODE (-c) = COPY
DISTRIBUTED MEMORY PARALLEL REQUESTED
4 PARALLEL PROCESSES REQUESTED WITH SINGLE THREAD PER PROCESS
TOTAL OF 4 CORES REQUESTED
INPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5FBA\dummy.dat
OUTPUT FILE NAME = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5FBA\solve.out
START-UP FILE MODE = NOREAD
STOP FILE MODE = NOREAD
RELEASE= 2024 R2 BUILD= 24.2 UP20240603 VERSION=WINDOWS x64
CURRENT JOBNAME=file0 18:12:54 JAN 08, 2025 CP= 0.094
PARAMETER _DS_PROGRESS = 999.0000000
/INPUT FILE= ds.dat LINE= 0
*** NOTE *** CP = 0.406 TIME= 18:12:54
The /CONFIG,NOELDB command is not valid in a distributed memory
parallel solution. Command is ignored.
*GET _WALLSTRT FROM ACTI ITEM=TIME WALL VALUE= 18.2150000
TITLE=
wbnew--Harmonic Response (G4)
ACT Extensions:
LSDYNA, 2024.2
5f463412-bd3e-484b-87e7-cbc0a665e474, wbex
/COM, ANSYSMotion, 2024.2
20180725-3f81-49eb-9f31-41364844c769, wbex
SET PARAMETER DIMENSIONS ON _WB_PROJECTSCRATCH_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_PROJECTSCRATCH_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5FBA\
SET PARAMETER DIMENSIONS ON _WB_SOLVERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_SOLVERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\
SET PARAMETER DIMENSIONS ON _WB_USERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_USERFILES_DIR(1) = C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\user_files\
--- Data in consistent NMM units. See Solving Units in the help system for more
MPA UNITS SPECIFIED FOR INTERNAL
LENGTH = MILLIMETERS (mm)
MASS = TONNE (Mg)
TIME = SECONDS (sec)
TEMPERATURE = CELSIUS (C)
TOFFSET = 273.0
FORCE = NEWTON (N)
HEAT = MILLIJOULES (mJ)
INPUT UNITS ARE ALSO SET TO MPA
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 18:12:54 JAN 08, 2025 CP= 0.453
wbnew--Harmonic Response (G4)
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
*********** Nodes for the whole assembly ***********
*********** Elements for Body 1 'Solid Body 1(External Model)' ***********
*********** Elements for Body 2 'Solid Body 2(External Model)' ***********
*********** Send User Defined Coordinate System(s) ***********
*********** Set Reference Temperature ***********
*********** Send Materials ***********
*********** Create Contact "Contacts(External Model) Index = 1" ***********
Real Constant Set For Above Contact Is 4 & 3
*********** Create Contact "Contacts(External Model) Index = 2" ***********
Real Constant Set For Above Contact Is 6 & 5
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Element Component ***********
*********** Send Named Selection as Node Component ***********
*********** Define Pressure Using Surface Effect Elements "Pressure" **********
*********** Create Pre-Meshed Cyclic Symmetry ***********
***** ROUTINE COMPLETED ***** CP = 2.234
--- Number of total nodes = 40644
--- Number of contact elements = 2930
--- Number of spring elements = 0
--- Number of bearing elements = 0
--- Number of solid elements = 23239
--- Number of condensed parts = 0
--- Number of total elements = 26169
*GET _WALLBSOL FROM ACTI ITEM=TIME WALL VALUE= 18.2152778
***** MAPDL SOLUTION ROUTINE *****
PERFORM A HARMONIC ANALYSIS
THIS WILL BE A NEW ANALYSIS
PERFORM A FULL HARMONIC RESPONSE ANALYSIS
THERMAL STRAINS ARE NOT INCLUDED IN THE LOAD VECTOR.
STEP BOUNDARY CONDITION KEY= 1
HARMONIC FREQUENCY RANGE - END= 5500.0 BEGIN= 1200.0
USE 20 SUBSTEP(S) THIS LOAD STEP FOR ALL DEGREES OF FREEDOM
STRUCTURAL DAMPING COEFFICIENT = 0.20000E-01
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EANG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE ETMP ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE VENG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE STRS ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPEL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE RSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE CONT ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
*GET ANSINTER_ FROM ACTI ITEM=INT VALUE= 0.00000000
*IF ANSINTER_ ( = 0.00000 ) NE
0 ( = 0.00000 ) THEN
*ENDIF
*** NOTE *** CP = 3.312 TIME= 18:12:56
The automatic domain decomposition logic has selected the FREQ domain
decomposition method with 1 processes per frequency solution.
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
*** WARNING *** CP = 6.859 TIME= 18:12:58
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** NOTE *** CP = 7.422 TIME= 18:12:59
The model data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5
5FBA\file0.err ) for these warning messages.
GENERATE CYCLIC SYMMETRY CONSTRAINT EQUATIONS
NUMBER OF CONSTRAINT EQUATIONS GENERATED= 6669
(USING THE MATCHED NODES ALGORITHM -- MAX NODE LOCATION ERROR = 0.78308E-02)
***** MAPDL SOLVE COMMAND *****
D I S T R I B U T E D D O M A I N D E C O M P O S E R
...Number of frequency solutions: 20
...Decompose to 4 frequency domains (with 1 processes per domain)
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 18:13:00 JAN 08, 2025 CP= 9.797
wbnew--Harmonic Response (G4)
S O L U T I O N O P T I O N S
PROBLEM DIMENSIONALITY. . . . . . . . . . . . .3-D
CYCLIC SYMMETRY SECTOR ANGLE. . . . . . . . . . 27.692 DEGREES
DEGREES OF FREEDOM. . . . . . UX UY UZ
ANALYSIS TYPE . . . . . . . . . . . . . . . . .HARMONIC
SOLUTION METHOD. . . . . . . . . . . . . . .FULL
OFFSET TEMPERATURE FROM ABSOLUTE ZERO . . . . . 273.15
THERMAL EXPANSION . . . . . . . . . . . . . . .OFF
COMPLEX DISPLACEMENT PRINT OPTION . . . . . . .REAL AND IMAGINARY
GLOBALLY ASSEMBLED MATRIX . . . . . . . . . . .SYMMETRIC
*** WARNING *** CP = 10.109 TIME= 18:13:00
Material number 7 (used by element 33548) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 10.281 TIME= 18:13:00
The step data was checked and warning messages were found.
Please review output or errors file (
C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5
5FBA\file0.err ) for these warning messages.
*** NOTE *** CP = 10.281 TIME= 18:13:00
The conditions for direct assembly have been met. No .emat or .erot
files will be produced.
*** NOTE *** CP = 11.406 TIME= 18:13:01
Symmetric Deformable- deformable contact pair identified by real
constant set 3 and contact element type 3 has been set up. The
companion pair has real constant set ID 5. Both pairs should have the
same behavior.
For asymmetric contact analysis, you may deactivate the current pair
and keep its companion pair.
Contact algorithm: Penalty method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.27905E+08
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.14334
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Default elastic slip factor SLTOL 0.50000E-02
The resulting elastic slip tolerance 0.92303E-02
Update contact stiffness at each iteration
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 1.8461
Average contact pair depth 1.4334
Average target surface length 1.4202
Default pinball region factor PINB 0.25000
The resulting pinball region 0.35836
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 11.406 TIME= 18:13:01
Min. Initial gap 0.119687086 was detected between contact element
32561 and target element 32831.
Contact is detected due to initial settings.
Max. Geometric gap 0.136413796 has been detected between contact
element 32668 and target element 32894.
****************************************
*** WARNING *** CP = 11.406 TIME= 18:13:01
Material number 7 (used by element 33548) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** WARNING *** CP = 11.406 TIME= 18:13:01
Material number 7 (used by element 33548) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 11.406 TIME= 18:13:01
Symmetric Deformable- deformable contact pair identified by real
constant set 5 and contact element type 5 has been set up. The
companion pair has real constant set ID 3. Both pairs should have the
same behavior.
For asymmetric contact analysis, you may keep the current pair and
deactivate its companion pair.
Contact algorithm: Penalty method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.27905E+08
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.11335
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Default elastic slip factor SLTOL 0.50000E-02
The resulting elastic slip tolerance 0.71970E-02
Update contact stiffness at each iteration
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 1.4394
Average contact pair depth 1.1335
Average target surface length 1.8316
Default pinball region factor PINB 0.25000
The resulting pinball region 0.28337
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 11.406 TIME= 18:13:01
Min. Initial gap 0.121762256 was detected between contact element
32999 and target element 33214.
Contact is detected due to initial settings.
Max. Geometric gap 0.136856675 has been detected between contact
element 33059 and target element 33401.
****************************************
*** WARNING *** CP = 11.406 TIME= 18:13:01
Material number 7 (used by element 33548) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 11.406 TIME= 18:13:01
Symmetric Deformable- deformable contact pair identified by real
constant set 10 and contact element type 3 has been set up. The
companion pair has real constant set ID 12. Both pairs should have
the same behavior.
For asymmetric contact analysis, you may deactivate the current pair
and keep its companion pair.
Contact algorithm: Penalty method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.27905E+08
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.14334
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Default elastic slip factor SLTOL 0.50000E-02
The resulting elastic slip tolerance 0.92303E-02
Update contact stiffness at each iteration
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 1.8461
Average contact pair depth 1.4334
Average target surface length 1.4202
Default pinball region factor PINB 0.25000
The resulting pinball region 0.35836
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 11.406 TIME= 18:13:01
Min. Initial gap 0.119687086 was detected between contact element
67902 and target element 68172.
Contact is detected due to initial settings.
Max. Geometric gap 0.136413796 has been detected between contact
element 68009 and target element 68235.
****************************************
*** NOTE *** CP = 11.406 TIME= 18:13:01
Symmetric Deformable- deformable contact pair identified by real
constant set 12 and contact element type 5 has been set up. The
companion pair has real constant set ID 10. Both pairs should have
the same behavior.
For asymmetric contact analysis, you may keep the current pair and
deactivate its companion pair.
Contact algorithm: Penalty method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.27905E+08
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.11335
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Default elastic slip factor SLTOL 0.50000E-02
The resulting elastic slip tolerance 0.71970E-02
Update contact stiffness at each iteration
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 1.4394
Average contact pair depth 1.1335
Average target surface length 1.8316
Default pinball region factor PINB 0.25000
The resulting pinball region 0.28337
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 11.406 TIME= 18:13:01
Min. Initial gap 0.121762256 was detected between contact element
68340 and target element 68555.
Contact is detected due to initial settings.
Max. Geometric gap 0.136856675 has been detected between contact
element 68400 and target element 68742.
****************************************
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 1
CYCLIC SYMMETRY HARMONIC INDEX. . . . . . . . . 0
FREQUENCY RANGE . . . . . . . . . . . . . . . . 1200.0 TO 5500.0
NUMBER OF SUBSTEPS. . . . . . . . . . . . . . . 20
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . YES
STRUCTURAL DAMPING COEFFICIENT. . . . . . . . . 0.20000E-01
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
RSOL ALL
CONT ALL
*** NOTE *** CP = 14.391 TIME= 18:13:01
Symmetric Deformable- deformable contact pair identified by real
constant set 3 and contact element type 3 has been set up. The
companion pair has real constant set ID 5. Both pairs should have the
same behavior.
For asymmetric contact analysis, you may deactivate the current pair
and keep its companion pair.
Contact algorithm: Penalty method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.27905E+08
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.14334
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Default elastic slip factor SLTOL 0.50000E-02
The resulting elastic slip tolerance 0.92303E-02
Update contact stiffness at each iteration
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 1.8461
Average contact pair depth 1.4334
Average target surface length 1.4202
Default pinball region factor PINB 0.25000
The resulting pinball region 0.35836
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 14.391 TIME= 18:13:01
Min. Initial gap 0.119687086 was detected between contact element
32561 and target element 32831.
Contact is detected due to initial settings.
Max. Geometric gap 0.136413796 has been detected between contact
element 32668 and target element 32894.
****************************************
*** NOTE *** CP = 14.391 TIME= 18:13:01
Symmetric Deformable- deformable contact pair identified by real
constant set 5 and contact element type 5 has been set up. The
companion pair has real constant set ID 3. Both pairs should have the
same behavior.
For asymmetric contact analysis, you may keep the current pair and
deactivate its companion pair.
Contact algorithm: Penalty method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.27905E+08
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.11335
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Default elastic slip factor SLTOL 0.50000E-02
The resulting elastic slip tolerance 0.71970E-02
Update contact stiffness at each iteration
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 1.4394
Average contact pair depth 1.1335
Average target surface length 1.8316
Default pinball region factor PINB 0.25000
The resulting pinball region 0.28337
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 14.391 TIME= 18:13:01
Min. Initial gap 0.121762256 was detected between contact element
32999 and target element 33214.
Contact is detected due to initial settings.
Max. Geometric gap 0.136856675 has been detected between contact
element 33059 and target element 33401.
****************************************
*** NOTE *** CP = 14.391 TIME= 18:13:01
Symmetric Deformable- deformable contact pair identified by real
constant set 10 and contact element type 3 has been set up. The
companion pair has real constant set ID 12. Both pairs should have
the same behavior.
For asymmetric contact analysis, you may deactivate the current pair
and keep its companion pair.
Contact algorithm: Penalty method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.27905E+08
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.14334
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Default elastic slip factor SLTOL 0.50000E-02
The resulting elastic slip tolerance 0.92303E-02
Update contact stiffness at each iteration
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 1.8461
Average contact pair depth 1.4334
Average target surface length 1.4202
Default pinball region factor PINB 0.25000
The resulting pinball region 0.35836
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 14.391 TIME= 18:13:01
Min. Initial gap 0.119687086 was detected between contact element
67902 and target element 68172.
Contact is detected due to initial settings.
Max. Geometric gap 0.136413796 has been detected between contact
element 68009 and target element 68235.
****************************************
*** NOTE *** CP = 14.391 TIME= 18:13:01
Symmetric Deformable- deformable contact pair identified by real
constant set 12 and contact element type 5 has been set up. The
companion pair has real constant set ID 10. Both pairs should have
the same behavior.
For asymmetric contact analysis, you may keep the current pair and
deactivate its companion pair.
Contact algorithm: Penalty method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 10.000
The resulting initial contact stiffness 0.27905E+08
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.11335
Default opening contact stiffness OPSF will be used.
Default tangent stiffness factor FKT 1.0000
Default elastic slip factor SLTOL 0.50000E-02
The resulting elastic slip tolerance 0.71970E-02
Update contact stiffness at each iteration
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 1.4394
Average contact pair depth 1.1335
Average target surface length 1.8316
Default pinball region factor PINB 0.25000
The resulting pinball region 0.28337
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 14.391 TIME= 18:13:01
Min. Initial gap 0.121762256 was detected between contact element
68340 and target element 68555.
Contact is detected due to initial settings.
Max. Geometric gap 0.136856675 has been detected between contact
element 68400 and target element 68742.
****************************************
**** CENTER OF MASS, MASS, AND MASS MOMENTS OF INERTIA ****
CALCULATIONS ASSUME ELEMENT MASS AT ELEMENT CENTROID
TOTAL MASS = 0.38387E-03
MOM. OF INERTIA MOM. OF INERTIA
CENTER OF MASS ABOUT ORIGIN ABOUT CENTER OF MASS
XC = 36.912 IXX = 5.701 IXX = 0.7970E-01
YC = 117.35 IYY = 1.140 IYY = 0.2817
ZC = 29.541 IZZ = 6.082 IZZ = 0.2729
IXY = -1.699 IXY = -0.3613E-01
IYZ = -1.329 IYZ = 0.1306E-02
IZX = -0.4730 IZX = -0.5439E-01
*** MASS SUMMARY BY ELEMENT TYPE ***
TYPE MASS
1 0.190222E-03
2 0.193645E-03
Range of element maximum matrix coefficients in global coordinates
Maximum = 9031711.02 at element 67895.
Minimum = 40118.4069 at element 49928.
*** ELEMENT MATRIX FORMULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 24706 SOLID187 2.891 0.000117
2 21772 SOLID187 2.750 0.000126
3 806 CONTA174 0.172 0.000213
4 330 TARGE170 0.000 0.000000
5 330 CONTA174 0.094 0.000284
6 806 TARGE170 0.000 0.000000
7 3588 SURF154 0.266 0.000074
Time at end of element matrix formulation CP = 21.296875.
*** NOTE *** CP = 22.438 TIME= 18:13:11
The initial memory allocation (-m) has been exceeded.
Supplemental memory allocations are being used.
SPARSE MATRIX DIRECT SOLVER.
Number of equations = 229620, Maximum wavefront = 276
Memory allocated on this process
-------------------------------------------------------------------
Equation solver memory allocated = 2531.406 MB
Equation solver memory required for in-core mode = 2421.917 MB
Equation solver memory required for out-of-core mode = 701.570 MB
Total (solver and non-solver) memory allocated = 4171.383 MB
*** NOTE *** CP = 24.594 TIME= 18:13:14
The Sparse Matrix Solver is currently running in the in-core memory
mode. This memory mode uses the most amount of memory in order to
avoid using the hard drive as much as possible, which most often
results in the fastest solution time. This mode is recommended if
enough physical memory is present to accommodate all of the solver
data.
Sparse solver maximum pivot= 17281797.7 at node 27603 UY.
Sparse solver minimum pivot= 10088.7419 at node 1358 UY.
Sparse solver minimum pivot in absolute value= 10088.7419 at node 1358
UY.
*** ELEMENT RESULT CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 24706 SOLID187 2.391 0.000097
2 21772 SOLID187 2.312 0.000106
3 806 CONTA174 0.031 0.000039
5 330 CONTA174 0.078 0.000237
7 3588 SURF154 0.234 0.000065
*** NODAL LOAD CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 24706 SOLID187 0.641 0.000026
2 21772 SOLID187 0.641 0.000029
3 806 CONTA174 0.000 0.000000
5 330 CONTA174 0.016 0.000047
7 3588 SURF154 0.047 0.000013
*** LOAD STEP 1 SUBSTEP 1 COMPLETED. FREQUENCY= 1415.00
*** LOAD STEP 1 SUBSTEP 2 COMPLETED. FREQUENCY= 1630.00
*** LOAD STEP 1 SUBSTEP 3 COMPLETED. FREQUENCY= 1845.00
*** LOAD STEP 1 SUBSTEP 4 COMPLETED. FREQUENCY= 2060.00
*** LOAD STEP 1 SUBSTEP 5 COMPLETED. FREQUENCY= 2275.00
*** LOAD STEP 1 SUBSTEP 6 COMPLETED. FREQUENCY= 2490.00
*** LOAD STEP 1 SUBSTEP 7 COMPLETED. FREQUENCY= 2705.00
*** LOAD STEP 1 SUBSTEP 8 COMPLETED. FREQUENCY= 2920.00
*** LOAD STEP 1 SUBSTEP 9 COMPLETED. FREQUENCY= 3135.00
*** LOAD STEP 1 SUBSTEP 10 COMPLETED. FREQUENCY= 3350.00
*** LOAD STEP 1 SUBSTEP 11 COMPLETED. FREQUENCY= 3565.00
*** LOAD STEP 1 SUBSTEP 12 COMPLETED. FREQUENCY= 3780.00
*** LOAD STEP 1 SUBSTEP 13 COMPLETED. FREQUENCY= 3995.00
*** LOAD STEP 1 SUBSTEP 14 COMPLETED. FREQUENCY= 4210.00
*** LOAD STEP 1 SUBSTEP 15 COMPLETED. FREQUENCY= 4425.00
*** LOAD STEP 1 SUBSTEP 16 COMPLETED. FREQUENCY= 4640.00
*** LOAD STEP 1 SUBSTEP 17 COMPLETED. FREQUENCY= 4855.00
*** LOAD STEP 1 SUBSTEP 18 COMPLETED. FREQUENCY= 5070.00
*** LOAD STEP 1 SUBSTEP 19 COMPLETED. FREQUENCY= 5285.00
*** LOAD STEP 1 SUBSTEP 20 COMPLETED. FREQUENCY= 5500.00
*** MAPDL BINARY FILE STATISTICS
BUFFER SIZE USED= 16384
24.000 MB WRITTEN ON ELEMENT SAVED DATA FILE: file0.esav
192.062 MB WRITTEN ON ASSEMBLED MATRIX FILE: file0.full
197.125 MB WRITTEN ON RESULTS FILE: file0.rst
*************** Write FE CONNECTORS *********
WRITE OUT CONSTRAINT EQUATIONS TO FILE= file.ce
************ Write Pre-Meshed Cyclic Pairs ************
*GET NUM_PAIRS FROM PARM CYCLIC_XREF_N ITEM=DIM 2 VALUE= 4446.00000
*IF num_pairs ( = 4446.00 ) NE
0 ( = 0.00000 ) THEN
OPENED FILE= cyclic_map.json FOR COMMAND FILE DATA
*DO LOOP ON PARAMETER= I FROM 1.0000 TO 4446.0 BY 1.0000
*ENDDO INDEX= I
COMMAND FILE CLOSED
*ENDIF
FINISH SOLUTION PROCESSING
***** ROUTINE COMPLETED ***** CP = 211.078
PRINTOUT RESUMED BY /GOP
*GET _WALLASOL FROM ACTI ITEM=TIME WALL VALUE= 18.2805556
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R2 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=WINDOWS x64 18:16:50 JAN 08, 2025 CP= 211.109
wbnew--Harmonic Response (G4)
***** MAPDL RESULTS INTERPRETATION (POST1) *****
*** NOTE *** CP = 211.109 TIME= 18:16:50
Reading results into the database (SET command) will update the current
displacement and force boundary conditions in the database with the
values from the results file for that load set. Note that any
subsequent solutions will use these values unless action is taken to
either SAVE the current values or not overwrite them (/EXIT,NOSAVE).
Set Encoding of XML File to:ISO-8859-1
Set Output of XML File to:
PARM, , , , , , , , , , , ,
, , , , , , ,
DATABASE WRITTEN ON FILE parm.xml
EXIT THE MAPDL POST1 DATABASE PROCESSOR
***** ROUTINE COMPLETED ***** CP = 211.125
PRINTOUT RESUMED BY /GOP
*GET _WALLDONE FROM ACTI ITEM=TIME WALL VALUE= 18.2805556
PARAMETER _PREPTIME = 1.000000000
PARAMETER _SOLVTIME = 235.0000000
PARAMETER _POSTTIME = 0.000000000
PARAMETER _TOTALTIM = 236.0000000
*GET _DLBRATIO FROM ACTI ITEM=SOLU DLBR VALUE= 0.00000000
*GET _COMBTIME FROM ACTI ITEM=SOLU COMB VALUE= 1.52494450
*GET _SSMODE FROM ACTI ITEM=SOLU SSMM VALUE= 2.00000000
*GET _NDOFS FROM ACTI ITEM=SOLU NDOF VALUE= 229620.000
/FCLEAN COMMAND REMOVING ALL LOCAL FILES
--- Total number of nodes = 81288
--- Total number of elements = 52338
--- Element load balance ratio = 0
--- Time to combine distributed files = 1.5249445
--- Sparse memory mode = 2
--- Number of DOF = 229620
EXIT MAPDL WITHOUT SAVING DATABASE
NUMBER OF WARNING MESSAGES ENCOUNTERED= 5
NUMBER OF ERROR MESSAGES ENCOUNTERED= 0
+--------------------- M A P D L S T A T I S T I C S ------------------------+
Release: 2024 R2 Build: 24.2 Update: UP20240603 Platform: WINDOWS x64
Date Run: 01/08/2025 Time: 18:16 Process ID: 14472
Operating System: Windows 11 (Build: 22631)
Processor Model: Intel(R) Xeon(R) Platinum 8171M CPU @ 2.60GHz
Compiler: Intel(R) Fortran Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) C/C++ Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) oneAPI Math Kernel Library Version 2023.1-Product Build 20230303
Number of machines requested : 1
Total number of cores available : 8
Number of physical cores available : 4
Number of processes requested : 4
Number of threads per process requested : 1
Total number of cores requested : 4 (Distributed Memory Parallel)
MPI Type: INTELMPI
MPI Version: Intel(R) MPI Library 2021.11 for Windows* OS
GPU Acceleration: Not Requested
Job Name: file0
Input File: dummy.dat
Core Machine Name Working Directory
-----------------------------------------------------
0 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5FBA
1 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5FBA
2 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5FBA
3 pyworkbench C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\_ProjectScratch\Scr5FBA
Latency time from master to core 1 = 3.113 microseconds
Latency time from master to core 2 = 3.361 microseconds
Latency time from master to core 3 = 3.056 microseconds
Communication speed from master to core 1 = 4604.21 MB/sec
Communication speed from master to core 2 = 4652.46 MB/sec
Communication speed from master to core 3 = 5233.72 MB/sec
Total CPU time for main thread : 203.2 seconds
Total CPU time summed for all threads : 211.3 seconds
Elapsed time spent obtaining a license : 0.5 seconds
Elapsed time spent pre-processing model (/PREP7) : 0.6 seconds
Elapsed time spent solution - preprocessing : 2.3 seconds
Elapsed time spent computing solution : 226.2 seconds
Elapsed time spent solution - postprocessing : 1.5 seconds
Elapsed time spent post-processing model (/POST1) : 0.0 seconds
Equation solver used : Sparse (symmetric)
Equation solver computational rate : 6.8 Gflops
Sum of disk space used on all processes : 2301.8 MB
Sum of memory used on all processes : 11719.0 MB
Sum of memory allocated on all processes : 15207.0 MB
Physical memory available : 32 GB
Total amount of I/O written to disk : 2.3 GB
Total amount of I/O read from disk : 1.8 GB
+------------------ E N D M A P D L S T A T I S T I C S -------------------+
*-----------------------------------------------------------------------------*
| |
| RUN COMPLETED |
| |
|-----------------------------------------------------------------------------|
| |
| Ansys MAPDL 2024 R2 Build 24.2 UP20240603 WINDOWS x64 |
| |
|-----------------------------------------------------------------------------|
| |
| Database Requested(-db) 1024 MB Scratch Memory Requested 1024 MB |
| Max Database Used(Master) 61 MB Max Scratch Used(Master) 2871 MB |
| Max Database Used(Workers) 59 MB Max Scratch Used(Workers) 2870 MB |
| Sum Database Used(All) 238 MB Sum Scratch Used(All) 11481 MB |
| |
|-----------------------------------------------------------------------------|
| |
| CP Time (sec) = 211.266 Time = 18:16:52 |
| Elapsed Time (sec) = 240.000 Date = 01/08/2025 |
| |
*-----------------------------------------------------------------------------*
Specify the Mechanical directory path for images and run a script to fetch the directory path. The path where images are stored on the server is printed. Download an image file (deformation.png
) from the server to the client’s current working directory and display it using matplotlib
.
[16]:
from matplotlib import image as mpimg
from matplotlib import pyplot as plt
[17]:
mechanical.run_python_script(f"image_dir=ExtAPI.DataModel.AnalysisList[5].WorkingDir")
result_image_dir_server = mechanical.run_python_script(f"image_dir")
print(f"Images are stored on the server at: {result_image_dir_server}")
Images are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\
[18]:
def get_image_path(image_name):
return os.path.join(result_image_dir_server, image_name)
[19]:
def display_image(path):
print(f"Printing {path} using matplotlib")
image1 = mpimg.imread(path)
plt.figure(figsize=(15, 15))
plt.axis("off")
plt.imshow(image1)
plt.show()
[20]:
image_name = "deformation.png"
image_path_server = get_image_path(image_name)
[21]:
if image_path_server != "":
current_working_directory = os.getcwd()
local_file_path_list = mechanical.download(
image_path_server, target_dir=current_working_directory
)
image_local_path = local_file_path_list[0]
print(f"Local image path : {image_local_path}")
display_image(image_local_path)
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\deformation.png to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\deformation.png: 100%|██████████| 11.6k/11.6k [00:00<?, ?B/s]
Local image path : C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\deformation.png
Printing C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\deformation.png using matplotlib
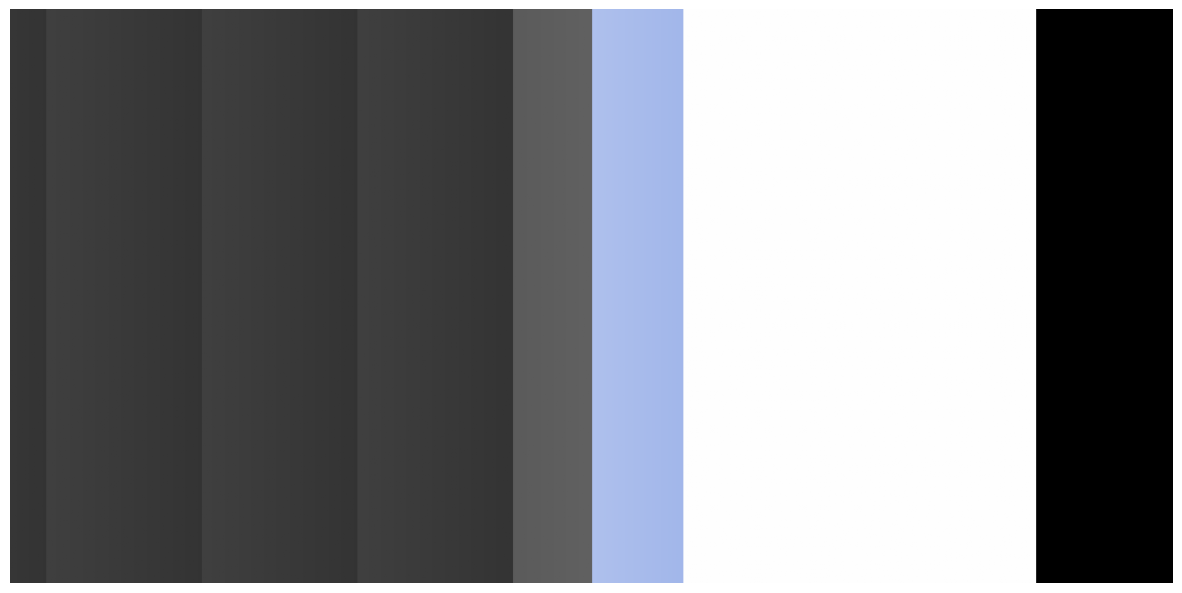
Download all the files from the server to the current working directory. Verify the source path for the directory and copy all files from the server to the client.
[22]:
mechanical.run_python_script(f"solve_dir=ExtAPI.DataModel.AnalysisList[5].WorkingDir")
result_solve_dir_server = mechanical.run_python_script(f"solve_dir")
print(f"All solver files are stored on the server at: {result_solve_dir_server}")
All solver files are stored on the server at: C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\
[23]:
solve_out_path = os.path.join(result_solve_dir_server, "*.*")
[24]:
current_working_directory = os.getcwd()
mechanical.download(solve_out_path, target_dir=current_working_directory)
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\CAERep.xml to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\CAERep.xml: 100%|██████████| 171k/171k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\CAERepOutput.xml to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\CAERepOutput.xml: 100%|██████████| 789/789 [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\cyclic_map.json to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\cyclic_map.json: 100%|██████████| 109k/109k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\deformation.png to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\deformation.png: 100%|██████████| 11.6k/11.6k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\ds.dat to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\ds.dat: 100%|██████████| 7.50M/7.50M [00:00<00:00, 503MB/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\file.aapresults to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\file.aapresults: 100%|██████████| 1.07k/1.07k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\file.rst to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\file.rst: 100%|██████████| 751M/751M [00:06<00:00, 117MB/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\file0.err to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\file0.err: 100%|██████████| 1.48k/1.48k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\MatML.xml to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\MatML.xml: 100%|██████████| 28.4k/28.4k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:57020:C:\Users\ansys\AppData\Local\Temp\WB_ansys_19576_2\wbnew_files\dp0\SYS-6\MECH\solve.out to C:\Users\ansys\actions-runner\_work\pyworkbench-examples\pyworkbench-examples\pyworkbench-examples\doc\source\examples\cyclic-symmetry-analysis\solve.out: 100%|██████████| 50.3k/50.3k [00:00<?, ?B/s]
[24]:
['C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\CAERep.xml',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\CAERepOutput.xml',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\cyclic_map.json',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\deformation.png',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\ds.dat',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\file.aapresults',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\file.rst',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\file0.err',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\MatML.xml',
'C:\\Users\\ansys\\actions-runner\\_work\\pyworkbench-examples\\pyworkbench-examples\\pyworkbench-examples\\doc\\source\\examples\\cyclic-symmetry-analysis\\solve.out']
Finally, the exit
method is called on both the PyMechanical and Workbench clients to gracefully shut down the services, ensuring that all resources are properly released.
[25]:
mechanical.exit()
wb.exit()